BaseManipulation is the base class of any manipulation object of the library. More...
#include <BaseManipulation.hpp>
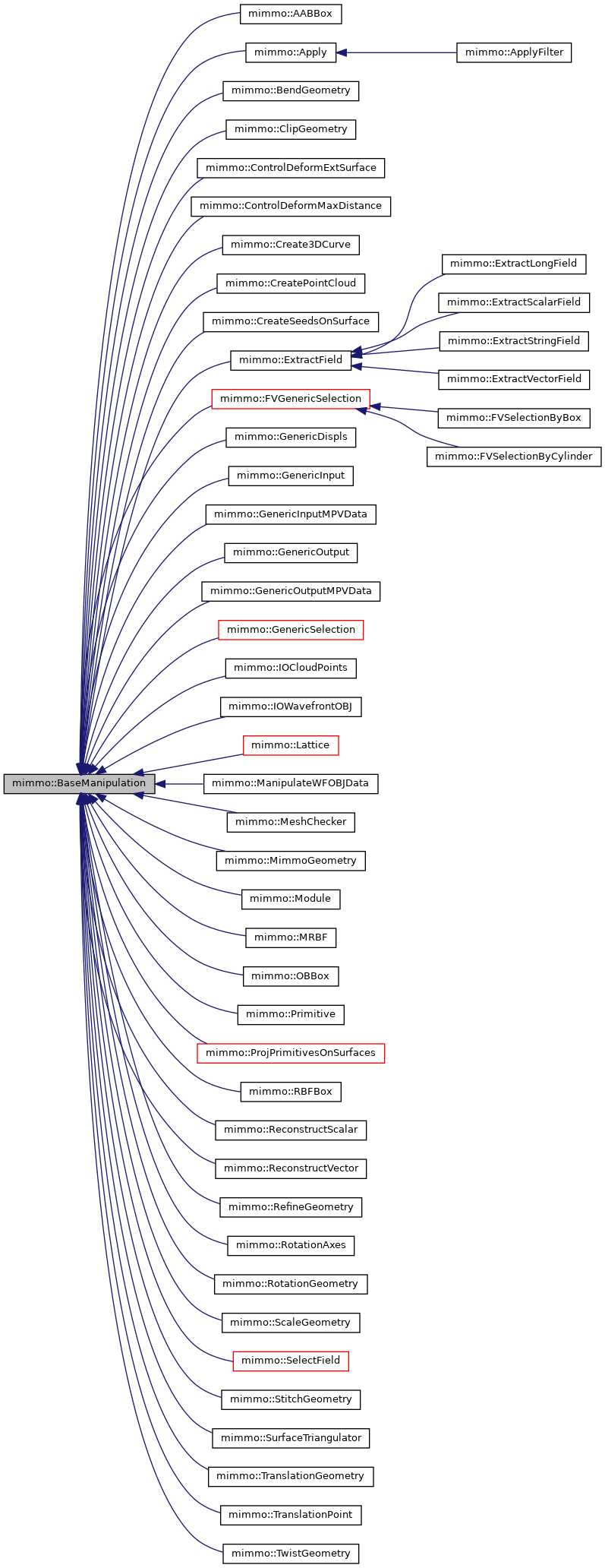
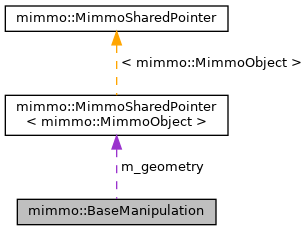
Public Types | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
Public Member Functions | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
virtual void | buildPorts ()=0 |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
virtual void | execute ()=0 |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Static Protected Attributes | |
static int | sm_baseManipulationCounter |
Friends | |
void | mimmo::setLogger (std::string log) |
void | mimmo::setLoggerDirectory (std::string dir) |
bool | pin::addPin (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced) |
bool | pin::checkCompatibility (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR) |
void | pin::removeAllPins (BaseManipulation *objSend, BaseManipulation *objRec) |
void | pin::removePin (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR) |
void | PortOut::exec () |
Detailed Description
BaseManipulation is the base class of any manipulation object of the library.
BaseManipulation is the base class used to derive each manipulation object of mimmo API.
This base class provides some common interface methods, as the base get/set methods.
To execute an object derived from BaseManipulation call the method exec() of the base class. In the exec() function the pure virtual execute() method is called. Pure virtual execute() is the real working function of a manipulation object and has to be implemented in each derived class.
A manipulation base object has a linked geometry, a MimmoObject, that is the target geometry to manipulate.
A set of manipulation objects can be rearranged in an execution chain and linked in order to communicate each other useful data and geometries. The exchange of such data is realized through ports of input/output; two objects (sender/receiver) can be linked by two ports (output/input) that communicate the same type of data.
The ports of a manipulation object are built in buildPorts() method. This function is a pure virtual method for the base class, i.e. buildPorts() has to be implemented for each derived. In buildPorts() of the derived class function will be defined all the input/output allowed connections of a manipulation object.
See mimmo::pin namespace and examples for further information about the linking procedure of BaseManipulation derived objects.
Please note, when copying a derived object by operators/constructors of the base class, ports and linking are not copied and left empty. Copy of BaseManipulation members in itself or in its derivations retains only the following parameters of BaseManipulation:
- link to target geometry;
- name;
- supported type of Ports.
For further information about PortType specification please refer to PortType enum documentation.
BaseManipulation controls a initial set of xml attributes which can be read from a xml file interface or written to it, through absorbSectionXML/flushSectionXML methods. Such parameters are:
- ClassName: specific name identifying the class as "mimmo.XXXXX"
- Priority: uint marking priority in multi-chain execution;
- Apply: boolean 0/1 activate apply result directly in execution;
- PlotInExecution: boolean 0/1 print optional results of the class, for debugging purpose.
- OutputPlot: target directory for optional results writing.
All BaseManipulation derived classes inherite these attributes.
Definition at line 102 of file BaseManipulation.hpp.
Member Typedef Documentation
◆ ConnectionType
Connection type specification for Manipulation object.
Definition at line 137 of file BaseManipulation.hpp.
◆ PortID
typedef std::string mimmo::BaseManipulation::PortID |
Port ID (identifier of the port).
Definition at line 138 of file BaseManipulation.hpp.
Constructor & Destructor Documentation
◆ BaseManipulation() [1/2]
mimmo::BaseManipulation::BaseManipulation | ( | ) |
Default constructor of BaseManipulation. It sets to zero/null each member/pointer.
Definition at line 36 of file BaseManipulation.cpp.
◆ ~BaseManipulation()
|
virtual |
Default destructor of BaseManipulation.
Definition at line 74 of file BaseManipulation.cpp.
◆ BaseManipulation() [2/2]
mimmo::BaseManipulation::BaseManipulation | ( | const BaseManipulation & | other | ) |
Copy constructor of BaseManipulation. Preexistent port connections will not be copied.
- Parameters
-
[in] other class of type BaseManipulation
Definition at line 83 of file BaseManipulation.cpp.
Member Function Documentation
◆ _apply()
|
protected |
Apply a deformation displacements field to the linked geometry. After the method call the geometry is permanently modified.
- Parameters
-
[in] displacements deformation vector field
Definition at line 956 of file BaseManipulation.cpp.
◆ absorbSectionXML()
|
virtual |
Base method to absorb parameter infos from an XML parser class of bitpit.This method absorbs only the base attributes specified in the BaseManipulation class general documentation. Derived classes using their own attributes, need to write a personal absorbSectionXML.
- Parameters
-
[in] slotXML reference to a Section slot of bitpit::Config class. [in] name string name associated to the slot. OPTIONAL
Reimplemented in mimmo::SelectionByElementList, mimmo::SelectionByBoxWithScalar, mimmo::SelectionByPID, mimmo::SelectionByMapping, mimmo::SelectStringField, mimmo::FVSelectionBySphere, mimmo::SelectLongField, mimmo::IOWavefrontOBJ, mimmo::SelectionBySphere, mimmo::SelectVectorField, mimmo::FVSelectionByCylinder, mimmo::SelectionByCylinder, mimmo::GenericInputMPVData, mimmo::GenericOutputMPVData, mimmo::MimmoGeometry, mimmo::MRBF, mimmo::ReconstructVector, mimmo::ManipulateWFOBJData, mimmo::SelectScalarField, mimmo::FVSelectionByBox, mimmo::FFDLattice, mimmo::SelectionByBox, mimmo::IOCloudPoints, mimmo::CreateSeedsOnSurface, mimmo::GenericInput, mimmo::BendGeometry, mimmo::ControlDeformExtSurface, mimmo::Create3DCurve, mimmo::GenericOutput, mimmo::GenericDispls, mimmo::SpecularPoints, mimmo::OBBox, mimmo::Lattice, mimmo::ReconstructScalar, mimmo::MeshChecker, mimmo::AABBox, mimmo::CreatePointCloud, mimmo::SelectField, mimmo::ExtractField, mimmo::RefineGeometry, mimmo::ProjPatchOnSurface, mimmo::Apply, mimmo::TwistGeometry, mimmo::ProjSegmentOnSurface, mimmo::ClipGeometry, mimmo::RotationAxes, mimmo::RotationGeometry, mimmo::ControlDeformMaxDistance, mimmo::RBFBox, mimmo::Primitive, mimmo::StitchGeometry, mimmo::ScaleGeometry, mimmo::SurfaceTriangulator, mimmo::TranslationGeometry, mimmo::TranslationPoint, mimmo::ApplyFilter, and mimmo::Module.
Definition at line 615 of file BaseManipulation.cpp.
◆ activate()
void mimmo::BaseManipulation::activate | ( | ) |
It activates the object during the execution.
Definition at line 480 of file BaseManipulation.cpp.
◆ addChild()
|
protected |
It adds a child manipulator object to the children linked by this object.
- Parameters
-
[in] child Pointer to child manipulator object.
Definition at line 749 of file BaseManipulation.cpp.
◆ addParent()
|
protected |
It adds a manipulator object linked by this object.
- Parameters
-
[in] parent Pointer to parent manipulator object.
Definition at line 735 of file BaseManipulation.cpp.
◆ addPinIn()
|
protected |
It adds an input pin (connection) of the object.
- Parameters
-
[in] objIn Pointer to sender BaseManipulation object. [in] portR ID of target input port.
Definition at line 821 of file BaseManipulation.cpp.
◆ addPinOut()
|
protected |
It adds an output pin (connection) of the object.
- Parameters
-
[in] objOut Pointer to receiver BaseManipulation object. [in] portS ID of target output port of sender. [in] portR ID of target input port of receiver.
Definition at line 833 of file BaseManipulation.cpp.
◆ apply()
|
protectedvirtual |
Apply directly the result of your blocks without the need of a follower applier block. The current method for the BaseManipulation class do nothing. For Programmers only: to customize your optional direct apply create your own implementation of this method in each BaseManipulation derived block.
Reimplemented in mimmo::MRBF, mimmo::FFDLattice, mimmo::BendGeometry, mimmo::TwistGeometry, mimmo::RotationGeometry, mimmo::ScaleGeometry, and mimmo::TranslationGeometry.
Definition at line 915 of file BaseManipulation.cpp.
◆ arePortsBuilt()
bool mimmo::BaseManipulation::arePortsBuilt | ( | ) |
It gets if the ports of this object are already built.
- Returns
- true/false if ports are set.
Definition at line 206 of file BaseManipulation.cpp.
◆ buildPorts()
|
protectedpure virtual |
Build ports of the class. Pure virtual method.
Implemented in mimmo::SelectionByElementList, mimmo::SelectionByBoxWithScalar, mimmo::SelectionByPID, mimmo::SelectStringField, mimmo::SelectionByMapping, mimmo::ExtractStringField, mimmo::FVSelectionBySphere, mimmo::IOWavefrontOBJ, mimmo::SelectLongField, mimmo::SelectionBySphere, mimmo::ExtractLongField, mimmo::FVSelectionByCylinder, mimmo::SelectVectorField, mimmo::SelectionByCylinder, mimmo::ExtractVectorField, mimmo::GenericInputMPVData, mimmo::GenericOutputMPVData, mimmo::ManipulateWFOBJData, mimmo::ReconstructVector, mimmo::FVSelectionByBox, mimmo::SelectScalarField, mimmo::SelectionByBox, mimmo::ExtractScalarField, mimmo::MimmoGeometry, mimmo::MRBF, mimmo::FFDLattice, mimmo::Create3DCurve, mimmo::IOCloudPoints, mimmo::GenericInput, mimmo::BendGeometry, mimmo::CreateSeedsOnSurface, mimmo::ControlDeformExtSurface, mimmo::CreatePointCloud, mimmo::MeshChecker, mimmo::GenericOutput, mimmo::GenericDispls, mimmo::ProjPatchOnSurface, mimmo::ReconstructScalar, mimmo::Lattice, mimmo::SpecularPoints, mimmo::SelectField, mimmo::RefineGeometry, mimmo::OBBox, mimmo::ExtractField, mimmo::FVGenericSelection, mimmo::TwistGeometry, mimmo::Primitive, mimmo::AABBox, mimmo::RotationAxes, mimmo::ClipGeometry, mimmo::Apply, mimmo::ControlDeformMaxDistance, mimmo::RotationGeometry, mimmo::GenericSelection, mimmo::StitchGeometry, mimmo::ScaleGeometry, mimmo::TranslationGeometry, mimmo::RBFBox, mimmo::SurfaceTriangulator, mimmo::ProjPrimitivesOnSurfaces, mimmo::TranslationPoint, mimmo::ApplyFilter, and mimmo::Module.
◆ cleanBufferIn()
|
protected |
It cleans the buffer stored in an input port of the object.
- Parameters
-
[in] port ID of the port.
Definition at line 726 of file BaseManipulation.cpp.
◆ clear()
void mimmo::BaseManipulation::clear | ( | ) |
It clears the object, by setting to zero/nullptr each member/pointer in the object.
Definition at line 540 of file BaseManipulation.cpp.
◆ createPortIn() [1/2]
|
protected |
It adds an input port to the object.The output ports compatible tags can send data to this input port. The compatibility between ports with same tag is automatic.
- Parameters
-
[in] obj_ Pointer to BaseManipulation object. [in] setVar_ Get function of the object (copy return) linked by the sender port. [in] portR marker of the port; the port will be created at portR-th slot of the input ports of the object. [in] mandatory does the port have to be mandatorily linked? [in] family tag of family of mandatory ports (family=0 independent ports with no alternative).
- Returns
- true/false, if port is created
Definition at line 125 of file BaseManipulation.tpp.
◆ createPortIn() [2/2]
|
protected |
It adds an input port to the object. The output ports with compatible container/data tags can send data to this input port. The compatibility between ports with same tag is automatic.
- Parameters
-
[in] var_ Pointer to member to fill. [in] portR marker of the port; the port will be created at portR-th slot of the input ports of the object. [in] mandatory does the port have to be mandatorily linked? [in] family tag of family of mandatory ports (family=0 independent ports with no alternative).
- Returns
- true/false, if port is created
Definition at line 94 of file BaseManipulation.tpp.
◆ createPortOut() [1/2]
|
protected |
It adds an output port to the object.The input ports with compatible tags can receive data form this output port. The compatibility between ports with same tag is automatic.
- Parameters
-
[in] obj_ Pointer to BaseManipulation object. [in] getVar_ Get function of the object (copy return) linked by the sender port. [in] portS marker of the port; the port will be created at portS-th slot of the output ports of the object.
- Returns
- true/false, if port is created
Definition at line 64 of file BaseManipulation.tpp.
◆ createPortOut() [2/2]
|
protected |
It adds an output port to the object.The input ports with compatible tags can receive data form this output port. The compatibility between ports with same tag is automatic.
- Parameters
-
[in] var_ Pointer to T member to communicate. [in] portS marker of the port; the port will be created at portS-th slot of the output ports of the object.
- Returns
- true/false, if port is created
Definition at line 35 of file BaseManipulation.tpp.
◆ deletePorts()
|
protected |
Protected utility to delete all port of a class BaseManipulation
Definition at line 690 of file BaseManipulation.cpp.
◆ disable()
void mimmo::BaseManipulation::disable | ( | ) |
It disables the object during the execution.
Definition at line 488 of file BaseManipulation.cpp.
◆ exec()
void mimmo::BaseManipulation::exec | ( | ) |
Execution command. exec() runs the execution of output pins (connections) at the end of the execution. execute is pure virtual and it has to be implemented in a derived class.
- Examples
- core_example_00002.cpp, utils_example_00001.cpp, and utils_example_00002.cpp.
Definition at line 552 of file BaseManipulation.cpp.
◆ execute()
|
protectedpure virtual |
Execution method. Pure virtual method, it has to be implemented in derived classes.
Implemented in mimmo::SelectionByBoxWithScalar, mimmo::IOWavefrontOBJ, mimmo::GenericInputMPVData, mimmo::GenericOutputMPVData, mimmo::MimmoGeometry, mimmo::MRBF, mimmo::ReconstructVector, mimmo::ManipulateWFOBJData, mimmo::FFDLattice, mimmo::IOCloudPoints, mimmo::CreateSeedsOnSurface, mimmo::GenericInput, mimmo::BendGeometry, mimmo::ControlDeformExtSurface, mimmo::Create3DCurve, mimmo::GenericOutput, mimmo::GenericDispls, mimmo::SpecularPoints, mimmo::OBBox, mimmo::Lattice, mimmo::ReconstructScalar, mimmo::MeshChecker, mimmo::AABBox, mimmo::CreatePointCloud, mimmo::SelectField, mimmo::ExtractField, mimmo::RefineGeometry, mimmo::TwistGeometry, mimmo::ClipGeometry, mimmo::RotationAxes, mimmo::Apply, mimmo::GenericSelection, mimmo::ControlDeformMaxDistance, mimmo::RotationGeometry, mimmo::RBFBox, mimmo::Primitive, mimmo::StitchGeometry, mimmo::ProjPrimitivesOnSurfaces, mimmo::ScaleGeometry, mimmo::FVGenericSelection, mimmo::TranslationPoint, mimmo::SurfaceTriangulator, mimmo::TranslationGeometry, mimmo::ApplyFilter, and mimmo::Module.
◆ findPinIn()
It finds an input pin (connection) of the object
- Parameters
-
[in] pin Target pin (connection).
- Returns
- Index of target pin in the input pins structure. Return "NONE" if pin (connection) not found.
Definition at line 795 of file BaseManipulation.cpp.
◆ findPinOut()
It finds an output pin (connection) of the object
- Parameters
-
[in] pin Target pin (connection).
- Returns
- Index of target pin in the output pins structure. Return "NONE" if pin (connection) not found.
Definition at line 809 of file BaseManipulation.cpp.
◆ flushSectionXML()
|
virtual |
Base method to flush parameter infos to an XML parser class of bitpit. This method flushes only the base attributes specified in the BaseManipulation class general documentation. Derived classes using their own attributes, need to write a personal flushSectionXML.
- Parameters
-
[in] slotXML reference to a Section slot of bitpit::Config class. [in] name string name associated to the slot.OPTIONAL
Reimplemented in mimmo::SelectionByElementList, mimmo::SelectionByBoxWithScalar, mimmo::SelectionByPID, mimmo::SelectionByMapping, mimmo::SelectStringField, mimmo::FVSelectionBySphere, mimmo::SelectLongField, mimmo::IOWavefrontOBJ, mimmo::SelectionBySphere, mimmo::SelectVectorField, mimmo::FVSelectionByCylinder, mimmo::SelectionByCylinder, mimmo::GenericInputMPVData, mimmo::GenericOutputMPVData, mimmo::MimmoGeometry, mimmo::MRBF, mimmo::ReconstructVector, mimmo::ManipulateWFOBJData, mimmo::SelectScalarField, mimmo::FVSelectionByBox, mimmo::FFDLattice, mimmo::SelectionByBox, mimmo::IOCloudPoints, mimmo::CreateSeedsOnSurface, mimmo::GenericInput, mimmo::BendGeometry, mimmo::ControlDeformExtSurface, mimmo::Create3DCurve, mimmo::GenericOutput, mimmo::GenericDispls, mimmo::SpecularPoints, mimmo::OBBox, mimmo::Lattice, mimmo::ReconstructScalar, mimmo::MeshChecker, mimmo::AABBox, mimmo::CreatePointCloud, mimmo::SelectField, mimmo::ExtractField, mimmo::RefineGeometry, mimmo::ProjPatchOnSurface, mimmo::Apply, mimmo::TwistGeometry, mimmo::ProjSegmentOnSurface, mimmo::ClipGeometry, mimmo::RotationAxes, mimmo::RotationGeometry, mimmo::ControlDeformMaxDistance, mimmo::RBFBox, mimmo::Primitive, mimmo::StitchGeometry, mimmo::ScaleGeometry, mimmo::SurfaceTriangulator, mimmo::TranslationGeometry, mimmo::TranslationPoint, mimmo::ApplyFilter, and mimmo::Module.
Definition at line 670 of file BaseManipulation.cpp.
◆ getChild()
BaseManipulation * mimmo::BaseManipulation::getChild | ( | int | i = 0 | ) |
It gets one child object linked by this object.
- Parameters
-
[in] i Index of target child.
- Returns
- Pointer to i-th child manipulator object.
Definition at line 301 of file BaseManipulation.cpp.
◆ getConnectionType()
BaseManipulation::ConnectionType mimmo::BaseManipulation::getConnectionType | ( | ) |
It gets the ports-type of the manipulation object.
- Returns
- ConnectionType of manipulation object (bi-directional, only backward, only forward).
Definition at line 328 of file BaseManipulation.cpp.
◆ getGeometry()
MimmoSharedPointer< MimmoObject > mimmo::BaseManipulation::getGeometry | ( | ) |
It gets the geometry linked by the manipulator object.
- Returns
- Pointer to geometry to be deformed by the manipulator object. Note. It gets a copy of a MimmoSharedPointer of the geometry.
- Examples
- core_example_00001.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 235 of file BaseManipulation.cpp.
◆ getGeometryReference()
MimmoSharedPointer< MimmoObject > & mimmo::BaseManipulation::getGeometryReference | ( | ) |
It gets the geometry linked by the manipulator object.
- Returns
- Pointer reference to geometry to be deformed by the manipulator object. Note. It gets the reference of a MimmoSharedPointer of the geometry.
Definition at line 245 of file BaseManipulation.cpp.
◆ getId()
int mimmo::BaseManipulation::getId | ( | ) |
- Returns
- integer identifier of the object
Definition at line 398 of file BaseManipulation.cpp.
◆ getLog()
bitpit::Logger & mimmo::BaseManipulation::getLog | ( | ) |
Get the logger.
- Returns
- Reference to logger object.
Definition at line 197 of file BaseManipulation.cpp.
◆ getName()
std::string mimmo::BaseManipulation::getName | ( | ) |
It gets the name of the manipulator object.
- Returns
- Name of the manipulator object.
Definition at line 225 of file BaseManipulation.cpp.
◆ getNChild()
int mimmo::BaseManipulation::getNChild | ( | ) |
It gets the number of children linked to the manipulator object.
- Returns
- Number of children.
Definition at line 291 of file BaseManipulation.cpp.
◆ getNParent()
int mimmo::BaseManipulation::getNParent | ( | ) |
It gets the number of parents linked to the manipulator object.
- Returns
- Number of parents.
Definition at line 254 of file BaseManipulation.cpp.
◆ getNPortsIn()
int mimmo::BaseManipulation::getNPortsIn | ( | ) |
It gets the number of input ports of the object.
- Returns
- Number of input ports of the object.
Definition at line 337 of file BaseManipulation.cpp.
◆ getNPortsOut()
int mimmo::BaseManipulation::getNPortsOut | ( | ) |
It gets the number of output ports of the object.
- Returns
- Number of output ports of the object.
Definition at line 346 of file BaseManipulation.cpp.
◆ getParent()
BaseManipulation * mimmo::BaseManipulation::getParent | ( | int | i = 0 | ) |
It gets the manipulator object linked by this object.
- Parameters
-
[in] i Index of target parent.
- Returns
- Pointer to i-th parent manipulator object.
Definition at line 264 of file BaseManipulation.cpp.
◆ getPortsIn()
It gets all the input ports of the object
- Returns
- Map with PortID as key and pointer to input ports as value.
Definition at line 355 of file BaseManipulation.cpp.
◆ getPortsOut()
It gets all the output ports of the object
- Returns
- Map with PortID as key and pointer to output ports as value.
Definition at line 364 of file BaseManipulation.cpp.
◆ getPriority()
uint mimmo::BaseManipulation::getPriority | ( | ) |
Get current unsigned int marking priority of execution of class in a multi-chain frame.
- Returns
- priority mark
Definition at line 216 of file BaseManipulation.cpp.
◆ getSubBlocksEmbedded()
|
virtual |
Method to return all possible executable bloks of type BaseManipulation embedded in the current class. For example create a BaseManipulation executable object with its own ports which wraps a certain number of other BaseManipulation executable objects with their own i/o ports, independent from their father. By default this method return nothing. It needs to be customized according to functionality of your executable derived class.
- Returns
- list of children BaseManipulation object pointers
Definition at line 927 of file BaseManipulation.cpp.
◆ initializeLogger()
|
protected |
Initialize the logger.
- Parameters
-
[in] logexist does a logger already exist? NOTE: console verbosity set to NORMAL as default. If a logger already exists the verbosities of the external logger are used.
Definition at line 164 of file BaseManipulation.cpp.
◆ isActive()
bool mimmo::BaseManipulation::isActive | ( | ) |
It gets if the object is activates or disable during the execution.
- Returns
- True/false if the object is activates or disable during the execution.
Definition at line 389 of file BaseManipulation.cpp.
◆ isApply()
bool mimmo::BaseManipulation::isApply | ( | ) |
- Returns
- true if the feature to apply the results of the block is active.
Definition at line 380 of file BaseManipulation.cpp.
◆ isChild()
bool mimmo::BaseManipulation::isChild | ( | BaseManipulation * | target, |
int & | index | ||
) |
Return true if the target is contained in the child list.
- Parameters
-
[in] target BaseManipulation target object [out] index Actual position in the list.
- Returns
- false if not found.
Definition at line 313 of file BaseManipulation.cpp.
◆ isParent()
bool mimmo::BaseManipulation::isParent | ( | BaseManipulation * | target, |
int & | index | ||
) |
Return true if the target is contained in the parent list.
- Parameters
-
[in] target BaseManipulation target object [out] index Actual position in the list (may vary if the parent list is modified).
- Returns
- false if not found.
Definition at line 276 of file BaseManipulation.cpp.
◆ isPlotInExecution()
bool mimmo::BaseManipulation::isPlotInExecution | ( | ) |
- Returns
- true if the feature to plot optional results of the block is active.
Definition at line 372 of file BaseManipulation.cpp.
◆ operator=()
BaseManipulation & mimmo::BaseManipulation::operator= | ( | const BaseManipulation & | other | ) |
Assignement operator of BaseManipulation.
- Parameters
-
[in] other class of type BaseManipulation
Definition at line 113 of file BaseManipulation.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot optional data related to your blocks. The current method for the BaseManipulation class do nothing. For Programmers only: to customize your optional results plotting create your own reimplementation of this method in each BaseManipulation derived block.
Reimplemented in mimmo::SelectionByBoxWithScalar, mimmo::SelectStringField, mimmo::ExtractStringField, mimmo::SelectLongField, mimmo::ExtractLongField, mimmo::SelectVectorField, mimmo::ExtractVectorField, mimmo::MRBF, mimmo::ReconstructVector, mimmo::FFDLattice, mimmo::SelectScalarField, mimmo::ExtractScalarField, mimmo::IOCloudPoints, mimmo::CreateSeedsOnSurface, mimmo::ControlDeformExtSurface, mimmo::Create3DCurve, mimmo::SpecularPoints, mimmo::OBBox, mimmo::Lattice, mimmo::ReconstructScalar, mimmo::CreatePointCloud, mimmo::AABBox, mimmo::MeshChecker, mimmo::RefineGeometry, mimmo::ClipGeometry, mimmo::ControlDeformMaxDistance, mimmo::RBFBox, mimmo::StitchGeometry, mimmo::GenericSelection, mimmo::FVGenericSelection, mimmo::ProjPrimitivesOnSurfaces, mimmo::SurfaceTriangulator, and mimmo::Module.
Definition at line 908 of file BaseManipulation.cpp.
◆ readBufferIn()
|
protected |
It reads the buffer stored in an input port of the object.
- Parameters
-
[in] port ID of the port that reads the buffer and stores the value in the related variable.
Definition at line 717 of file BaseManipulation.cpp.
◆ removePinIn() [1/2]
|
protected |
It removes an input pin (connection) of the object and the related output pin (connection) of the linked object.
- Parameters
-
[in] objIn Pointer to sender BaseManipulation object. [in] portR ID of target input port of receiver.
Definition at line 847 of file BaseManipulation.cpp.
◆ removePinIn() [2/2]
|
protected |
It removes an input pin (connection) of the object and the related output pin (connection) of the linked object.
- Parameters
-
[in] portR Port ID of target input pin (connection). [in] j Index of target output pin (connection) of the portR input port.
Definition at line 881 of file BaseManipulation.cpp.
◆ removePinOut() [1/2]
|
protected |
It removes an output pin (connection) of the object and the related input pin (connection) of the linked object.
- Parameters
-
[in] objOut Pointer to receiver BaseManipulation object. [in] portS ID of target output port of sender.
Definition at line 863 of file BaseManipulation.cpp.
◆ removePinOut() [2/2]
|
protected |
It removes an output pin (connection) of the object and the related input pin (connection) of the linked object.
- Parameters
-
[in] portS Port ID of target output pin (connection). [in] j Index of target output pin (connection) of the portS output port.
Definition at line 895 of file BaseManipulation.cpp.
◆ removePins()
void mimmo::BaseManipulation::removePins | ( | ) |
It removes all the pins (connections) of the object and the related pins of the linked objects.
Definition at line 505 of file BaseManipulation.cpp.
◆ removePinsIn()
void mimmo::BaseManipulation::removePinsIn | ( | ) |
It removes all the input pins (connections) of the object and the related output pins (connections) of the linked objects.
Definition at line 515 of file BaseManipulation.cpp.
◆ removePinsOut()
void mimmo::BaseManipulation::removePinsOut | ( | ) |
It removes all the output (connections) pins of the object and the related input pins (connections) of the linked objects.
Definition at line 528 of file BaseManipulation.cpp.
◆ setApply()
void mimmo::BaseManipulation::setApply | ( | bool | flag = true | ) |
Activates the feature to apply directly the results of the block if possible.
- Parameters
-
[in] flag true/false to activate/deactivate the feature
- Examples
- genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00003.cpp, manipulators_example_00001.cpp, and utils_example_00004.cpp.
Definition at line 463 of file BaseManipulation.cpp.
◆ setBufferIn()
|
protected |
It sets the buffer stored in an input port of the object.
- Parameters
-
[in] port ID of the input port. [in] input Reference to mimmo::IBinaryStream to store in m_ibuffer member of the port.
Definition at line 707 of file BaseManipulation.cpp.
◆ setGeometry()
void mimmo::BaseManipulation::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geometry | ) |
It sets the geometry linked by the manipulator object.
- Parameters
-
[in] geometry Pointer to geometry to be deformed by the manipulator object.
Definition at line 433 of file BaseManipulation.cpp.
◆ setId()
void mimmo::BaseManipulation::setId | ( | int | id | ) |
Set (force) integer identifier of the object
- Parameters
-
[in] id integer identifier
Definition at line 472 of file BaseManipulation.cpp.
◆ setLog()
void mimmo::BaseManipulation::setLog | ( | bitpit::Logger & | log | ) |
Set the logger.
- Parameters
-
[in] log logger object.
Definition at line 406 of file BaseManipulation.cpp.
◆ setName()
void mimmo::BaseManipulation::setName | ( | std::string | name | ) |
It sets the name of the manipulator object.
- Parameters
-
[in] name Name of the manipulator object.
- Examples
- genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, and utils_example_00004.cpp.
Definition at line 425 of file BaseManipulation.cpp.
◆ setOutputPlot()
void mimmo::BaseManipulation::setOutputPlot | ( | std::string | path | ) |
Set path to directory where the optional results will be stored, if setPlotInExecution feature is set active.
- Parameters
-
[in] path absolute path to specified directory, if empty use the default value "."
- Examples
- geohandlers_example_00002.cpp.
Definition at line 453 of file BaseManipulation.cpp.
◆ setPlotInExecution()
void mimmo::BaseManipulation::setPlotInExecution | ( | bool | flag | ) |
Activates the feature to plot optional results of the block. This features is meant for debug purpose only.
- Parameters
-
[in] flag true/false to activate/deactivate the feature
- Examples
- core_example_00002.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, genericinput_example_00005.cpp, geohandlers_example_00000.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, iocgns_example_00001.cpp, iocgns_example_00002.cpp, ioofoam_example_00001.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, and utils_example_00004.cpp.
Definition at line 443 of file BaseManipulation.cpp.
◆ setPriority()
void mimmo::BaseManipulation::setPriority | ( | uint | priority | ) |
Set unsigned int priority of execution of class in a multi-chain frame. value must be > 0;
- Parameters
-
[in] priority priority mark
Definition at line 416 of file BaseManipulation.cpp.
◆ swap()
|
protectednoexcept |
Exchange data between the current class and an external BaseManipulation object. Data will be swapped (data of A assigned to B and vice versa). Port connections/building info will not be exchanged, as well as class unique-id.
- Parameters
-
[in] x BaseManipulation object to be swapped with current class.
Definition at line 140 of file BaseManipulation.cpp.
◆ unsetChild()
|
protected |
Decrement target child multiplicity, contained in member m_child. If multiplicity is zero, erase target from list. The method is meant to be used in together to manual cut off of object pins.
- Parameters
-
[in] child Pointer to BaseManipulation object
Definition at line 780 of file BaseManipulation.cpp.
◆ unsetGeometry()
void mimmo::BaseManipulation::unsetGeometry | ( | ) |
It clears the pointer to the geometry linked by the object.
Definition at line 496 of file BaseManipulation.cpp.
◆ unsetParent()
|
protected |
Decrement target parent multiplicity, contained in member m_parent. If multiplicity is zero, erase target from list. The method is meant to be used together to manual cut off of object pins.
- Parameters
-
[in] parent Pointer to BaseManipulation object
Definition at line 764 of file BaseManipulation.cpp.
◆ write() [1/7]
|
protected |
Write an input geometry given as MimmoObject pointer. It writes into the m_output directory with m_name+m_counter
- Parameters
-
[in] geometry MimmoObject pointer to target geometry
Definition at line 979 of file BaseManipulation.cpp.
◆ write() [2/7]
|
protected |
Write an input geometry given as MimmoObject pointer with an input data field. Input geometry and the geometry linked in the MimmoPiercedVector of data have to be consistent, otherwise the data is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data field by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] data MimmoPiercedVector with data field to write
Definition at line 153 of file BaseManipulation.tpp.
◆ write() [3/7]
|
protected |
Write an input geometry given as MimmoObject pointer with a series of input data field (template variadic function). Input geometry and the geometry linked in the MimmoPiercedVectors of data have to be consistent, otherwise the data that doesn't satisfy the coherence is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data fields by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] data MimmoPiercedVector with data fields to write [in] args other data field series to write (template variadic function)
Definition at line 226 of file BaseManipulation.tpp.
◆ write() [4/7]
|
protected |
Write an input geometry given as MimmoObject pointer with a series of input data field (vector of data fields). Input geometry and the geometry linked in the MimmoPiercedVectors of data have to be consistent, otherwise the data that doesn't satisfy the coherence is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data fields by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] vdata vector of MimmoPiercedVector pointers with data fields to write
Definition at line 470 of file BaseManipulation.tpp.
◆ write() [5/7]
|
protected |
Write an input geometry given as MimmoObject pointer with a series of vectors of input data fields (template variadic function). Input geometry and the geometry linked in the MimmoPiercedVectors of data have to be consistent, otherwise the data that doesn't satisfy the coherence is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data fields by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] vdata vector of MimmoPiercedVector pointers with data fields to write [in] args other data field series to write (template variadic function)
Definition at line 554 of file BaseManipulation.tpp.
◆ write() [6/7]
|
protected |
Write an input geometry given as MimmoObject pointer with a series of input data field (vector of data fields). Input geometry and the geometry linked in the MimmoPiercedVectors of data have to be consistent, otherwise the data that doesn't satisfy the coherence is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data fields by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] vdata vector of MimmoPiercedVectors with data fields to write
Definition at line 297 of file BaseManipulation.tpp.
◆ write() [7/7]
|
protected |
Write an input geometry given as MimmoObject pointer with a series of vectors of input data fields (template variadic function). Input geometry and the geometry linked in the MimmoPiercedVectors of data have to be consistent, otherwise the data that doesn't satisfy the coherence is skipped. It writes into the m_output directory with m_name+m_counter. It adds the input data fields by deducing the type. Allowed types are : all scalars that verify is_floating_point or is_integral functions; mimmo vector types, i.e. std::array<double,3>.
- Parameters
-
[in] geometry MimmoObject shared pointer to target geometry [in] vdata vector of MimmoPiercedVector with data fields to write [in] args other data fields series to write (template variadic function)
Definition at line 384 of file BaseManipulation.tpp.
Friends And Related Function Documentation
◆ mimmo::setLogger
|
friend |
see mimmo::setLogger
◆ mimmo::setLoggerDirectory
|
friend |
see mimmo::setLoggerDirectory
◆ pin::addPin
|
friend |
see pin::addPin
◆ pin::checkCompatibility
|
friend |
◆ pin::removeAllPins
|
friend |
◆ pin::removePin
|
friend |
see pin::removePin
◆ PortOut::exec
|
friend |
see PortOut::exec
Member Data Documentation
◆ m_active
|
protected |
True/false to activate/disable the object during the execution.
Definition at line 156 of file BaseManipulation.hpp.
◆ m_apply
|
protected |
Activate apply result directly in execution.
Definition at line 158 of file BaseManipulation.hpp.
◆ m_arePortsBuilt
|
protected |
True or false is the ports are already set or not.
Definition at line 154 of file BaseManipulation.hpp.
◆ m_child
|
protected |
Pointers list to manipulation objects CHILD of the current class.List retains for each pointer a counter. When this counter is 0, pointer is released
Definition at line 147 of file BaseManipulation.hpp.
◆ m_counter
|
protected |
Counter ID associated to the object
Definition at line 143 of file BaseManipulation.hpp.
◆ m_execPlot
|
protected |
Activate plotting of optional result directly in execution.
Definition at line 157 of file BaseManipulation.hpp.
◆ m_geometry
|
protected |
Mimmo shared pointer to manipulated geometry.
Definition at line 144 of file BaseManipulation.hpp.
◆ m_log
|
protected |
Pointer to logger.
Definition at line 161 of file BaseManipulation.hpp.
◆ m_name
|
protected |
Name of the manipulation object.
Definition at line 142 of file BaseManipulation.hpp.
◆ m_outputPlot
|
protected |
Define path for plotting optional results in execution.
Definition at line 159 of file BaseManipulation.hpp.
◆ m_parent
|
protected |
Pointers list to manipulation objects FATHER of the current class. List retains for each pointer a counter. When this counter is 0, pointer is released
Definition at line 145 of file BaseManipulation.hpp.
◆ m_portIn
Input ports map.
Definition at line 152 of file BaseManipulation.hpp.
◆ m_portOut
Output ports map.
Definition at line 153 of file BaseManipulation.hpp.
◆ m_portsType
|
protected |
Type of ports of the object: BOTH (bidirectional), BACKWARD (only input) or FORWARD (only output).
Definition at line 150 of file BaseManipulation.hpp.
◆ m_priority
|
protected |
} Flag marking priority of execution of the object (0 - highest priority) >
Definition at line 141 of file BaseManipulation.hpp.
◆ sm_baseManipulationCounter
|
staticprotected |
Current global number of BaseManipulation object in the instance.
Definition at line 164 of file BaseManipulation.hpp.
The documentation for this class was generated from the following files:
- src/core/BaseManipulation.hpp
- src/core/BaseManipulation.cpp
- src/core/BaseManipulation.tpp
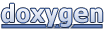