Class for projecting 1D/2D primitives on a target 3D surface mesh. More...
#include <ProjPrimitivesOnSurfaces.hpp>
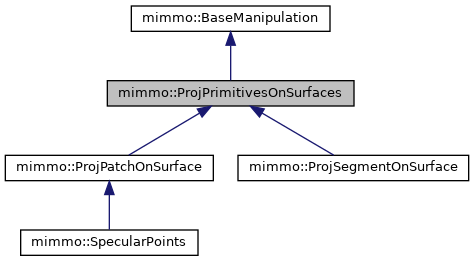
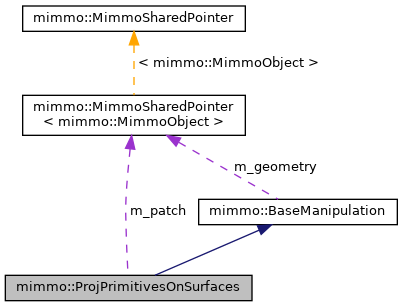
Protected Member Functions | |
virtual void | buildPorts () |
virtual void | projection ()=0 |
void | swap (ProjPrimitivesOnSurfaces &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_buildKdTree |
bool | m_buildSkdTree |
int | m_nC |
MimmoSharedPointer< MimmoObject > | m_patch |
int | m_topo |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Class for projecting 1D/2D primitives on a target 3D surface mesh.
Abstract class used as interface for objects of projecting elemental 1D or 2D primitives such as segments, circumferences or circles, triangles etc... on a 3D surface mesh defined by a MimmoObject.
Ports available in ProjPrimitivesOnSurfaces Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getProjectedElement | (MC_SCALAR, MD_MIMMO_) |
Definition at line 70 of file ProjPrimitivesOnSurfaces.hpp.
Constructor & Destructor Documentation
◆ ProjPrimitivesOnSurfaces() [1/2]
mimmo::ProjPrimitivesOnSurfaces::ProjPrimitivesOnSurfaces | ( | ) |
Default constructor of ProjPrimitivesOnSurfaces.
Definition at line 42 of file ProjPrimitivesOnSurfaces.cpp.
◆ ~ProjPrimitivesOnSurfaces()
|
virtual |
Default destructor of ProjPrimitivesOnSurfaces.
Definition at line 54 of file ProjPrimitivesOnSurfaces.cpp.
◆ ProjPrimitivesOnSurfaces() [2/2]
mimmo::ProjPrimitivesOnSurfaces::ProjPrimitivesOnSurfaces | ( | const ProjPrimitivesOnSurfaces & | other | ) |
Copy constructor of ProjPrimitivesOnSurfaces. No resulting projected data structure is copied.
Definition at line 59 of file ProjPrimitivesOnSurfaces.cpp.
Member Function Documentation
◆ buildPorts()
|
protectedvirtual |
Building ports of the class
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::ProjPatchOnSurface, and mimmo::SpecularPoints.
Definition at line 96 of file ProjPrimitivesOnSurfaces.cpp.
◆ clear()
void mimmo::ProjPrimitivesOnSurfaces::clear | ( | ) |
Clear all stuffs in your class
Definition at line 190 of file ProjPrimitivesOnSurfaces.cpp.
◆ execute()
|
virtual |
Execution command. Project the primitive on the target 3D surface.
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::SpecularPoints.
- Examples
- utils_example_00005.cpp.
Definition at line 201 of file ProjPrimitivesOnSurfaces.cpp.
◆ getProjectedElement()
MimmoSharedPointer< MimmoObject > mimmo::ProjPrimitivesOnSurfaces::getProjectedElement | ( | ) |
Get your current projected primitive as a 3D mesh
- Returns
- pointer to projected primitive as MimmoObject
- Examples
- utils_example_00005.cpp.
Definition at line 129 of file ProjPrimitivesOnSurfaces.cpp.
◆ getProjElementTargetNCells()
|
virtual |
Get the actual number of the cells that will be used to represent a discrete mesh of the primitive projection.
- Returns
- number of cells
Definition at line 119 of file ProjPrimitivesOnSurfaces.cpp.
◆ getTopology()
int mimmo::ProjPrimitivesOnSurfaces::getTopology | ( | ) |
Return the topology of the primitive element support. 0-0D(point clouds), 1-1D(3D curve), 2-2D (3D surfaces)
- Returns
- topology of the primitive element
Definition at line 110 of file ProjPrimitivesOnSurfaces.cpp.
◆ isEmpty()
bool mimmo::ProjPrimitivesOnSurfaces::isEmpty | ( | ) |
Check if resulting primitive element is present or not. True - no geometry evaluated, False otherwise.
- Returns
- empty flag
Definition at line 181 of file ProjPrimitivesOnSurfaces.cpp.
◆ operator=()
ProjPrimitivesOnSurfaces & mimmo::ProjPrimitivesOnSurfaces::operator= | ( | const ProjPrimitivesOnSurfaces & | other | ) |
Assignement operator of ProjPrimitivesOnSurfaces. No resulting projected data structure is copied.
Definition at line 69 of file ProjPrimitivesOnSurfaces.cpp.
◆ plotOptionalResults()
|
virtual |
Plot resulting projected element in a vtu mesh file;
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SpecularPoints.
Definition at line 222 of file ProjPrimitivesOnSurfaces.cpp.
◆ projection()
|
protectedpure virtual |
Projection function - Pure virtual method
Implemented in mimmo::ProjPatchOnSurface, and mimmo::ProjSegmentOnSurface.
◆ setBuildKdTree()
void mimmo::ProjPrimitivesOnSurfaces::setBuildKdTree | ( | bool | build | ) |
It sets if the KdTree the projected primitive element data structure has to be built during execution.
- Parameters
-
[in] build If true the KdTree is built in execution and stored in the related MimmoObject member.
Definition at line 163 of file ProjPrimitivesOnSurfaces.cpp.
◆ setBuildSkdTree()
void mimmo::ProjPrimitivesOnSurfaces::setBuildSkdTree | ( | bool | build | ) |
It sets if the SkdTree of the projected primitive element data structure has to be built during execution.
- Parameters
-
[in] build If true the skdTree is built in execution and stored in the related MimmoObject member.
Definition at line 154 of file ProjPrimitivesOnSurfaces.cpp.
◆ setGeometry()
void mimmo::ProjPrimitivesOnSurfaces::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Set a target external surface mesh where primitive projection need to be performed. Topology of the geometry must be of superficial type, so that MimmoObject::getType() must return 1, otherwise nothing will be set.
- Parameters
-
[in] geo pointer to MimmoObject
- Examples
- utils_example_00005.cpp.
Definition at line 142 of file ProjPrimitivesOnSurfaces.cpp.
◆ setProjElementTargetNCells()
void mimmo::ProjPrimitivesOnSurfaces::setProjElementTargetNCells | ( | int | nC | ) |
It sets the number of the cells to be used to represent in a discrete mesh the primitive projection.
- Parameters
-
[in] nC number of cells
Definition at line 171 of file ProjPrimitivesOnSurfaces.cpp.
◆ swap()
|
protectednoexcept |
Swap function.
- Parameters
-
[in] x object ot be swapped
Definition at line 82 of file ProjPrimitivesOnSurfaces.cpp.
Member Data Documentation
◆ m_buildKdTree
|
protected |
If true build KdTree of the projected element mesh.
Definition at line 76 of file ProjPrimitivesOnSurfaces.hpp.
◆ m_buildSkdTree
|
protected |
If true build SkdTree of of the projected element mesh.
Definition at line 75 of file ProjPrimitivesOnSurfaces.hpp.
◆ m_nC
|
protected |
Number of target elements of your 3D curve discrete projection
Definition at line 74 of file ProjPrimitivesOnSurfaces.hpp.
◆ m_patch
|
protected |
resulting projected elements stored as MimmoObject
Definition at line 77 of file ProjPrimitivesOnSurfaces.hpp.
◆ m_topo
|
protected |
Mark topology of your primitive element 1-one dimensional, 2- bi-dimensional
Definition at line 73 of file ProjPrimitivesOnSurfaces.hpp.
The documentation for this class was generated from the following files:
- src/utils/ProjPrimitivesOnSurfaces.hpp
- src/utils/ProjPrimitivesOnSurfaces.cpp
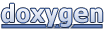