ProjPrimitivesOnSurfaces.cpp
59 ProjPrimitivesOnSurfaces::ProjPrimitivesOnSurfaces(const ProjPrimitivesOnSurfaces & other):BaseManipulation(other){
69 ProjPrimitivesOnSurfaces & ProjPrimitivesOnSurfaces::operator=(const ProjPrimitivesOnSurfaces &other){
98 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ProjPrimitivesOnSurfaces>(this, &mimmo::ProjPrimitivesOnSurfaces::setGeometry,M_GEOM, true));
100 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, ProjPrimitivesOnSurfaces>(this, &mimmo::ProjPrimitivesOnSurfaces::getProjectedElement, M_GEOM));
bool m_buildKdTree
Definition: ProjPrimitivesOnSurfaces.hpp:76
virtual void buildPorts()
Definition: ProjPrimitivesOnSurfaces.cpp:96
void plotOptionalResults()
Definition: ProjPrimitivesOnSurfaces.cpp:222
virtual int getProjElementTargetNCells()
Definition: ProjPrimitivesOnSurfaces.cpp:119
MimmoSharedPointer< MimmoObject > m_patch
Definition: ProjPrimitivesOnSurfaces.hpp:77
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: ProjPrimitivesOnSurfaces.cpp:142
ProjPrimitivesOnSurfaces()
Definition: ProjPrimitivesOnSurfaces.cpp:42
bool m_buildSkdTree
Definition: ProjPrimitivesOnSurfaces.hpp:75
int getTopology()
Definition: ProjPrimitivesOnSurfaces.cpp:110
void setProjElementTargetNCells(int nC)
Definition: ProjPrimitivesOnSurfaces.cpp:171
ProjPrimitivesOnSurfaces & operator=(const ProjPrimitivesOnSurfaces &other)
Definition: ProjPrimitivesOnSurfaces.cpp:69
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void projection()=0
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual ~ProjPrimitivesOnSurfaces()
Definition: ProjPrimitivesOnSurfaces.cpp:54
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
Class for projecting 1D/2D primitives on a target 3D surface mesh.
Definition: ProjPrimitivesOnSurfaces.hpp:70
void swap(ProjPrimitivesOnSurfaces &x) noexcept
Definition: ProjPrimitivesOnSurfaces.cpp:82
void setBuildSkdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:154
MimmoSharedPointer< MimmoObject > getProjectedElement()
Definition: ProjPrimitivesOnSurfaces.cpp:129
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setBuildKdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:163
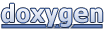