BaseManipulation.cpp
167 bitpit::log::manager().initialize(bitpit::log::Mode::COMBINED, MIMMO_LOG_FILE, true, MIMMO_LOG_DIR, m_nprocs, m_rank);
169 bitpit::log::manager().initialize(bitpit::log::Mode::COMBINED, MIMMO_LOG_FILE, true, MIMMO_LOG_DIR);
564 for (std::map<int, std::vector<PortIn*> >::iterator i=families.begin(); i!=families.end(); i++){
570 (*m_log) << "error: " << m_name << " mandatory port " << itID->second[count] << " not linked -> exit! " << std::endl;
583 (*m_log) << "error: " << m_name << " none of mandatory ports " << itID->second << " linked -> exit! " << std::endl;
596 for (std::unordered_map<PortID, PortOut*>::iterator i=m_portOut.begin(); i!=m_portOut.end(); i++){
691 for (std::unordered_map<PortID, PortOut*>::iterator i = m_portOut.begin(); i != m_portOut.end(); i++){
695 for (std::unordered_map<PortID, PortIn*>::iterator i = m_portIn.begin(); i != m_portIn.end(); i++){
PortID findPinOut(PortOut &pin)
Definition: BaseManipulation.cpp:809
void addPinOut(BaseManipulation *objOut, PortID portS, PortID portR)
Definition: BaseManipulation.cpp:833
BaseManipulation * getChild(int i=0)
Definition: BaseManipulation.cpp:301
virtual void plotOptionalResults()
Definition: BaseManipulation.cpp:908
ConnectionType getConnectionType()
Definition: BaseManipulation.cpp:328
void addParent(BaseManipulation *parent)
Definition: BaseManipulation.cpp:735
virtual void execute()=0
void removePinIn(BaseManipulation *objIn, PortID portR)
Definition: BaseManipulation.cpp:847
std::unordered_map< PortID, PortOut * > getPortsOut()
Definition: BaseManipulation.cpp:364
void setBufferIn(PortID port, mimmo::IBinaryStream &input)
Definition: BaseManipulation.cpp:707
void setOutputPlot(std::string path)
Definition: BaseManipulation.cpp:453
virtual ~BaseManipulation()
Definition: BaseManipulation.cpp:74
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void addPinIn(BaseManipulation *objIn, PortID portR)
Definition: BaseManipulation.cpp:821
BaseManipulation & operator=(const BaseManipulation &other)
Definition: BaseManipulation.cpp:113
void unsetChild(BaseManipulation *child)
Definition: BaseManipulation.cpp:780
BaseManipulation * getParent(int i=0)
Definition: BaseManipulation.cpp:264
ConnectionType
Type of allowed connections of the object: bidirectional, only input or only output.
Definition: MimmoNamespace.hpp:83
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
PortIn is the abstract PIN base class dedicated to carry data to a target class from other ones (inpu...
Definition: InOut.hpp:201
PortOut is the abstract PIN base class dedicated to exchange data from a target class to other ones (...
Definition: InOut.hpp:96
void addChild(BaseManipulation *child)
Definition: BaseManipulation.cpp:749
static int sm_baseManipulationCounter
Definition: BaseManipulation.hpp:164
bool isPlotInExecution()
Definition: BaseManipulation.cpp:372
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
bool isParent(BaseManipulation *, int &)
Definition: BaseManipulation.cpp:276
void initializeLogger(bool logexists)
Definition: BaseManipulation.cpp:164
std::unordered_map< PortID, PortOut * > m_portOut
Definition: BaseManipulation.hpp:153
void cleanBufferIn(PortID port)
Definition: BaseManipulation.cpp:726
void readBufferIn(PortID port)
Definition: BaseManipulation.cpp:717
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void removePinOut(BaseManipulation *objOut, PortID portS)
Definition: BaseManipulation.cpp:863
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
std::unordered_map< PortID, PortIn * > m_portIn
Definition: BaseManipulation.hpp:152
bool isChild(BaseManipulation *, int &)
Definition: BaseManipulation.cpp:313
std::unordered_map< PortID, PortIn * > getPortsIn()
Definition: BaseManipulation.cpp:355
void setPriority(uint priority)
Definition: BaseManipulation.cpp:416
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void removeAllPins(BaseManipulation *objSend, BaseManipulation *objRec)
Definition: MimmoNamespace.cpp:108
MimmoSharedPointer< MimmoObject > & getGeometryReference()
Definition: BaseManipulation.cpp:245
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual std::vector< BaseManipulation * > getSubBlocksEmbedded()
Definition: BaseManipulation.cpp:927
void unsetParent(BaseManipulation *parent)
Definition: BaseManipulation.cpp:764
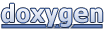