MimmoSharedPointer.hpp
41 mimmo::IBinaryStream& operator>>(mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer<T>& element);
44 mimmo::OBinaryStream& operator<<(mimmo::OBinaryStream &buffer, const mimmo::MimmoSharedPointer<T>& element);
144 friend mimmo::IBinaryStream& (::operator>>) (mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer<T>& element);
147 friend mimmo::OBinaryStream& (::operator<<) (mimmo::OBinaryStream &buffer, const mimmo::MimmoSharedPointer<T>& element);
mimmo custom derivation of bitpit OBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:55
mimmo::OBinaryStream & operator<<(mimmo::OBinaryStream &buffer, const mimmo::MimmoSharedPointer< T > &element)
Definition: MimmoSharedPointer.tpp:49
mimmo::IBinaryStream & operator>>(mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer< T > &element)
Definition: MimmoSharedPointer.tpp:33
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
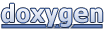