MimmoSharedPointer is a custom implementation of shared pointer. More...
#include <MimmoSharedPointer.hpp>

Public Member Functions | |
MimmoSharedPointer () | |
MimmoSharedPointer (const MimmoSharedPointer< O > &other) | |
MimmoSharedPointer (MimmoSharedPointer< O > &&other) | |
MimmoSharedPointer (O *object) | |
~MimmoSharedPointer () | |
O * | get () const |
std::size_t | getCounter () const |
operator bool () const | |
bool | operator! () const |
bool | operator!= (const long int lrhs) const |
bool | operator!= (const mimmo::MimmoSharedPointer< O > &rhs) const |
bool | operator!= (const O *_rhs) const |
bool | operator!= (const std::nullptr_t _rhs) const |
bool | operator!= (const void *_rhs) const |
O & | operator* () const |
O * | operator-> () const |
MimmoSharedPointer & | operator= (const MimmoSharedPointer< O > &other) |
MimmoSharedPointer & | operator= (MimmoSharedPointer< O > &&other) |
bool | operator== (const long int lrhs) const |
bool | operator== (const mimmo::MimmoSharedPointer< O > &rhs) const |
bool | operator== (const O *_rhs) const |
bool | operator== (const std::nullptr_t _rhs) const |
bool | operator== (const void *_rhs) const |
void | reset (O *object=nullptr) |
void | swap (MimmoSharedPointer< O > &other) noexcept |
Protected Member Functions | |
void | _decrement () |
void | _init (O *object=nullptr, std::size_t *counter=nullptr) |
void | _reset (O *object=nullptr, std::size_t *counter=nullptr) |
Protected Attributes | |
std::size_t * | m_counter |
O * | m_object |
Friends | |
template<class T > | |
mimmo::OBinaryStream & | operator<<) (mimmo::OBinaryStream &buffer, const mimmo::MimmoSharedPointer< T > &element) |
template<class T > | |
mimmo::IBinaryStream & | operator>>) (mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer< T > &element) |
Detailed Description
MimmoSharedPointer is a custom implementation of shared pointer.
MimmoSharedPointer is a custom implementation of shared pointer of mimmo library. It is basically a smart pointer who shares the ownership of a base pointer of a dynamically allocated class O (using c++ new) throughout its own multiple instances. Instances multiplicity is tracked incrementing/decrementing an internal counter. Each copy assignment/construction of a shared pointer increment the counter with respect to the copy source. A move assignment/construction does not increment counter, but move the contents of the source as they are to the new shared pointer; hence the source becomes a void object. When the last instance of the smart pointer is deleted (counter to 0), the internal object pointed is deleted too. Please beware, once a pointer to an allocated object is delegated to the shared pointer, the last one is responsible of the object destruction. If a forced external destruction occurs with a direct delete on the raw pointer object, it leads to memory violations inside the shared pointer.
Example of correct/incorrect usage of mimmo shared pointer are reported below. Please note, despite the examples don't due to the aim to highlight the incorrectness of usage, that it is a best practice to allocate dynamically using c++ new directly in a MimmoSharedPointer constructor.
SAMPLE 1 CORRECT
mimmo::MimmoSharedPointer<std::string> hold_ptr; //create a new empty pointer.
{
std::string * data = new std::string("haveSomeStringDataHere"); //allocate dynamically some string data
mimmo::MimmoSharedPointer<std::string> create_ptr(data); //use data pointer to create a mimmo shared pointer with counter 1.
hold_ptr = create_ptr; //share data instance with another shared pointer. Now counter is set to 2;
}
(after exiting scope create_ptr is destroyed, counter return to 1.
Now hold_ptr owns data instance with counter 1 and it will worry to destroy data,
when it destroys himself.)
SAMPLE 1 WRONG
mimmo::MimmoSharedPointer<std::string> hold_ptr; //create a new empty pointer.
{
std::string data("haveSomeStringDataHere"); //allocate statically some string data
mimmo::MimmoSharedPointer<std::string> create_ptr(&data); // use data pointer to create a mimmo shared pointer with counter 1.
hold_ptr = create_ptr; // share data instance with another shared pointer. Now counter is set to 2;
}
(after exiting scope create_ptr is destroyed, counter return to 1,
but string data allocated statically are destroyed when out of scope!
Now hold_ptr has counter 1 and holds a data pointer pointing to an already destroyed object
and when it tries to destroy himself(and data instance) this will lead to a memory violation).
SAMPLE 2 CORRECT
std::string * data = new std::string("haveSomeStringDataHere"); //allocate dynamically some string data
mimmo::MimmoSharedPointer<std::string> ptr1(data);//pass it to a mimmo shared pointer using base constructor
{
mimmo::MimmoSharedPointer<std::string> ptr2(ptr1); //pass the same data to another pointer usign base constructor
(create a safe copy of the pointer using always copy constructor or assignment,
that increment counter to 2.)
}
(after exiting ptr2 is destroyed and counter is decremented by 1, but data are still safe.
ptr1 is still alive with counter = 1 and a pointer pointing safely to data).
SAMPLE 2 WRONG
std::string * data = new std::string("haveSomeStringDataHere"); //allocate dynamically some string data
mimmo::MimmoSharedPointer<std::string> ptr1(data);//pass it to a mimmo shared pointer using base constructor
{
mimmo::MimmoSharedPointer<std::string> ptr2(data);//pass the same data to another pointer using base constructor
(both ptr1 and ptr2 owns the same data pointer with counter 1)
}
(after exiting ptr2 is destroyed and with him also data (ptr2 counter reached 0):
but ptr1 is still alive with counter 1 and a pointer pointing to a data already destroyed.
Destroying ptr1 will lead to a memory violation).
Definition at line 32 of file MimmoSharedPointer.hpp.
Constructor & Destructor Documentation
◆ MimmoSharedPointer() [1/4]
mimmo::MimmoSharedPointer::MimmoSharedPointer | ( | ) |
Default Constructor of MimmoSharedPointer.
Definition at line 63 of file MimmoSharedPointer.tpp.
◆ MimmoSharedPointer() [2/4]
mimmo::MimmoSharedPointer::MimmoSharedPointer | ( | O * | object | ) |
Constructor of MimmoSharedPointer. The class must be initialized with a pointer to a O type class, dynamically allocated. If a valid pointer is passed, the class will set its counter to 1.
- Parameters
-
[in] object raw pointer to a target object
Definition at line 76 of file MimmoSharedPointer.tpp.
◆ ~MimmoSharedPointer()
mimmo::MimmoSharedPointer::~MimmoSharedPointer | ( | ) |
Destructor. When a MimmoSharedPointer is destroyed the internal counter is decreased by one. If the counter reaches zero, the pointed object is explicitly deleted.
Definition at line 87 of file MimmoSharedPointer.tpp.
◆ MimmoSharedPointer() [3/4]
mimmo::MimmoSharedPointer::MimmoSharedPointer | ( | const MimmoSharedPointer< O > & | other | ) |
Copy constructor of MimmoSharedPointer. Counter is increased by one with respect the copy source.
- Parameters
-
[in] other Copied MimmoSharedPointer
Definition at line 97 of file MimmoSharedPointer.tpp.
◆ MimmoSharedPointer() [4/4]
mimmo::MimmoSharedPointer::MimmoSharedPointer | ( | MimmoSharedPointer< O > && | other | ) |
Move constructor of MimmoSharedPointer. Contents are moved from the target source to the current class, without incrementing internal counter. Target source becomes an empty shell, ready to be destroyed, without affecting its original contents.
- Parameters
-
[in] other Source MimmoSharedPointer to be moved
Definition at line 113 of file MimmoSharedPointer.tpp.
Member Function Documentation
◆ _decrement()
|
protected |
Method to decrement the internal class counter of 1. If the counter reaches zero, the internal pointed object is explicitly deleted.
Definition at line 309 of file MimmoSharedPointer.tpp.
◆ _init()
|
protected |
Initialize the shared pointer and the counter.
- Parameters
-
[in] object Pointer to target object [in] counter Pointer to counter of sharing pointers. If nullptr a new counter object is created. If object pointer is not nullptr the counter is increased by one.
Definition at line 277 of file MimmoSharedPointer.tpp.
◆ _reset()
|
protected |
Reset the class to new contents (raw object pointer and counter pointer). First old contents are released using _decrement() function. New contents are then allocated using _init(object, counter).
- Parameters
-
[in] object Pointer to target object [in] counter Pointer to counter of sharing pointers.
Definition at line 298 of file MimmoSharedPointer.tpp.
◆ get()
O * mimmo::MimmoSharedPointer::get | ( | ) | const |
- Returns
- raw pointer to internal object controlled by the class
Definition at line 198 of file MimmoSharedPointer.tpp.
◆ getCounter()
std::size_t mimmo::MimmoSharedPointer::getCounter | ( | ) | const |
Return the number of the multiple instances sharing the current pointed objects. In case of the raw internal pointer is nullptr, return 0.
- Returns
- internal counter status
Definition at line 209 of file MimmoSharedPointer.tpp.
◆ operator bool()
mimmo::MimmoSharedPointer::operator bool | ( | ) | const |
Boolean conversion operator.
- Returns
- Boolean conversion of raw pointer to object.
Definition at line 266 of file MimmoSharedPointer.tpp.
◆ operator!()
bool mimmo::MimmoSharedPointer::operator! | ( | ) | const |
Logical NOT operator.
- Returns
- True if raw internal pointer to object is nullptr.
Definition at line 256 of file MimmoSharedPointer.tpp.
◆ operator!=() [1/5]
|
inline |
Not equal operator of MimmoSharedPointer to long int.
- Parameters
-
[in] lrhs Tested long int
Definition at line 269 of file MimmoSharedPointer.hpp.
◆ operator!=() [2/5]
|
inline |
Not equal to operator of MimmoSharedPointer. It compares only pointer to object.
- Parameters
-
[in] rhs Tested MimmoSharedPointer
Definition at line 188 of file MimmoSharedPointer.hpp.
◆ operator!=() [3/5]
|
inline |
Not equal operator of MimmoSharedPointer to pure pointer to object type.
- Parameters
-
[in] _rhs Constant tested pointer to object
Definition at line 207 of file MimmoSharedPointer.hpp.
◆ operator!=() [4/5]
|
inline |
Not equal operator of MimmoSharedPointer to null pointer.
- Parameters
-
[in] _rhs Tested null pointer
Definition at line 245 of file MimmoSharedPointer.hpp.
◆ operator!=() [5/5]
|
inline |
Not equal operator of MimmoSharedPointer to void pointer.
- Parameters
-
[in] _rhs Tested void pointer
Definition at line 226 of file MimmoSharedPointer.hpp.
◆ operator*()
O & mimmo::MimmoSharedPointer::operator* | ( | ) | const |
Dereference operator.
- Returns
- Reference to internal pointed object.
Definition at line 236 of file MimmoSharedPointer.tpp.
◆ operator->()
O * mimmo::MimmoSharedPointer::operator-> | ( | ) | const |
Dereference operator.
- Returns
- Raw pointer to object.
Definition at line 246 of file MimmoSharedPointer.tpp.
◆ operator=() [1/2]
MimmoSharedPointer< O > & mimmo::MimmoSharedPointer::operator= | ( | const MimmoSharedPointer< O > & | other | ) |
Copy Assignment operator of MimmoSharedPointer. First, original contents of the class are released decrementing the relative counter of 1 (destruction of contents occurs only if such counter reaches 0). Then new contents from target source are copied into the current class, incrementing the counter by one.
- Parameters
-
[in] other Copied MimmoSharedPointer source
Definition at line 132 of file MimmoSharedPointer.tpp.
◆ operator=() [2/2]
MimmoSharedPointer< O > & mimmo::MimmoSharedPointer::operator= | ( | MimmoSharedPointer< O > && | other | ) |
Move Assignment operator of MimmoSharedPointer. First, original contents of the class are released decrementing the relative counter of 1 (destruction of contents occurs only if such counter reaches 0). Then, new contents are moved from the target source to the current class, without incrementing their internal counter. Finally, target source becomes an empty shell, ready to be destroyed, without affecting the contents.
- Parameters
-
[in] other Source MimmoSharedPointer to be moved
Definition at line 161 of file MimmoSharedPointer.tpp.
◆ operator==() [1/5]
|
inline |
Equal operator of MimmoSharedPointer to long int.
- Parameters
-
[in] lrhs Tested long int
Definition at line 254 of file MimmoSharedPointer.hpp.
◆ operator==() [2/5]
|
inline |
Equal to operator of MimmoSharedPointer. It compares only pointer to object.
- Parameters
-
[in] rhs Tested MimmoSharedPointer
Definition at line 179 of file MimmoSharedPointer.hpp.
◆ operator==() [3/5]
|
inline |
Equal operator of MimmoSharedPointer to pure pointer to object type.
- Parameters
-
[in] _rhs Tested pointer to object
Definition at line 197 of file MimmoSharedPointer.hpp.
◆ operator==() [4/5]
|
inline |
Equal operator of MimmoSharedPointer to null pointer.
- Parameters
-
[in] _rhs Tested null pointer
Definition at line 235 of file MimmoSharedPointer.hpp.
◆ operator==() [5/5]
|
inline |
Equal operator of MimmoSharedPointer to void pointer.
- Parameters
-
[in] _rhs Tested void pointer
Definition at line 216 of file MimmoSharedPointer.hpp.
◆ reset()
void mimmo::MimmoSharedPointer::reset | ( | O * | object = nullptr | ) |
Reset MimmoSharedPointer to a new target pointer or to nullptr. Old contents of the class are released decrementing their counter by one (ed eventually destroyed if the counter reaches 0). New target pointer will be held, with counter 1. If the new pointer is nullptr the class will be set to empty.
- Parameters
-
[in] object Pointer to target object
Definition at line 226 of file MimmoSharedPointer.tpp.
◆ swap()
|
noexcept |
Swap MimmoSharedPointer method. Swap contents between current class and another one of the same type, keeping both reference counting untouched.
- Parameters
-
[in] other Target shared pointer to swap
Definition at line 187 of file MimmoSharedPointer.tpp.
Member Data Documentation
◆ m_counter
|
protected |
Pointer to counter of MimmoSharedPointer objects sharing the pointer to target.
Definition at line 282 of file MimmoSharedPointer.hpp.
◆ m_object
|
protected |
Pointer to target objet.
Definition at line 281 of file MimmoSharedPointer.hpp.
The documentation for this class was generated from the following files:
- src/core/MimmoSharedPointer.hpp
- src/core/MimmoSharedPointer.tpp
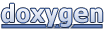