MimmoSharedPointer.tpp
33 mimmo::IBinaryStream& operator>>(mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer<T>& element){
49 mimmo::OBinaryStream& operator<<(mimmo::OBinaryStream &buffer, const mimmo::MimmoSharedPointer<T>& element)
330 size_t hash<mimmo::MimmoSharedPointer<O>>::operator()(const mimmo::MimmoSharedPointer<O> & obj) const
mimmo custom derivation of bitpit OBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:55
mimmo::OBinaryStream & operator<<(mimmo::OBinaryStream &buf, const std::string &element)
Definition: mimmo_binary_stream.cpp:66
mimmo::IBinaryStream & operator>>(mimmo::IBinaryStream &buffer, mimmo::MimmoSharedPointer< T > &element)
Definition: MimmoSharedPointer.tpp:33
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
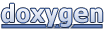