mimmo_binary_stream.hpp
93 mimmo::OBinaryStream& operator<<(mimmo::OBinaryStream &buf, const std::unordered_map<T, Q >& element);
mimmo custom derivation of bitpit OBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:55
mimmo::OBinaryStream & operator<<(mimmo::OBinaryStream &buf, const std::string &element)
Definition: mimmo_binary_stream.cpp:66
mimmo::IBinaryStream & operator>>(mimmo::IBinaryStream &buf, std::string &element)
Definition: mimmo_binary_stream.cpp:87
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
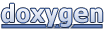