Utilities to create port connections between executable blocks. More...
Typedefs | |
typedef std::string | PortID |
Enumerations | |
enum | ConnectionType { ConnectionType::BOTH, ConnectionType::BACKWARD, ConnectionType::FORWARD } |
Type of allowed connections of the object: bidirectional, only input or only output. More... | |
Functions | |
bool | addPin (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced) |
bool | checkCompatibility (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR) |
void | removeAllPins (BaseManipulation *objSend, BaseManipulation *objRec) |
void | removePin (BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR) |
Detailed Description
Utilities to create port connections between executable blocks.
Here are collected enums and methods to create connections between blocks throughout established ports. All the available port types are registered (or need to be if not) in the API singleton mimmo::PortManager
Typedef Documentation
◆ PortID
typedef std::string mimmo::pin::PortID |
mimmo custom definition
Definition at line 89 of file MimmoNamespace.hpp.
Enumeration Type Documentation
◆ ConnectionType
|
strong |
Type of allowed connections of the object: bidirectional, only input or only output.
Definition at line 83 of file MimmoNamespace.hpp.
Function Documentation
◆ addPin()
bool mimmo::pin::addPin | ( | BaseManipulation * | objSend, |
BaseManipulation * | objRec, | ||
PortID | portS, | ||
PortID | portR, | ||
bool | forced | ||
) |
It adds a pin between two objects.
- Parameters
-
[in] objSend Pointer to BaseManipulation sender object. [in] objRec Pointer to BaseManipulation receiver object. [in] portS Port ID of the output port of sender object. [in] portR Port ID of the input port of receiver object. [in] forced If true it forces to build the connection without checking the compatibility between ports.
- Returns
- true if the pin is added.
- Examples
- genericinput_example_00001.cpp, genericinput_example_00002.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, genericinput_example_00005.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, geohandlers_example_00004.cpp, iocgns_example_00001.cpp, iocgns_example_00002.cpp, ioofoam_example_00001.cpp, ioofoam_example_00002.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00003.cpp, and utils_example_00004.cpp.
Definition at line 68 of file MimmoNamespace.cpp.
◆ checkCompatibility()
bool mimmo::pin::checkCompatibility | ( | BaseManipulation * | objSend, |
BaseManipulation * | objRec, | ||
PortID | portS, | ||
PortID | portR | ||
) |
It checks the compatibility between input and output ports of two objects.
- Parameters
-
[in] objSend Pointer to BaseManipulation sender object. [in] objRec Pointer to BaseManipulation receiver object. [in] portS Port ID of the output port of sender object. [in] portR Port ID of the input port of receiver object.
- Returns
- True if the pin is added.
Definition at line 165 of file MimmoNamespace.cpp.
◆ removeAllPins()
void mimmo::pin::removeAllPins | ( | BaseManipulation * | objSend, |
BaseManipulation * | objRec | ||
) |
It removes all pins between two objects.
- Parameters
-
[in] objSend Pointer to BaseManipulation sender object. [in] objRec Pointer to BaseManipulation receiver object.
Definition at line 108 of file MimmoNamespace.cpp.
◆ removePin()
void mimmo::pin::removePin | ( | BaseManipulation * | objSend, |
BaseManipulation * | objRec, | ||
PortID | portS, | ||
PortID | portR | ||
) |
It removes a pin between two objects. If the pin is found it is removed, otherwise nothing is done.
- Parameters
-
[in] objSend Pointer to BaseManipulation sender object. [in] objRec Pointer to BaseManipulation receiver object. [in] portS Port ID of the output port of sender object. [in] portR Port ID of the input port of receiver object.
Definition at line 147 of file MimmoNamespace.cpp.
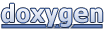