genericinput_example_00003.cpp
Example of reading displacements related to a 3x2x2 FFDLattice manipulator, and applying deformation onto a target mesh.Using: GenericDispls, FFDLattice, MimmoGeometry, Chain
To run : ./genericinput_example_00003
To run (MPI version): mpirun -np X genericinput_example_00003
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_iogeneric.hpp"
#include "FFDLattice.hpp"
// =================================================================================== //
void test00003() {
/*
Read and Write a STL geometry of a plane
*/
read->setReadFileType(FileType::STL);
read->setWriteFileType(FileType::SURFVTU);
/*
Creating a FFDLattice manipulator of dimension 3x2x2 around a box centered in 0,0,-0.5
with span 2,2,1
*/
latt->setOrigin({{0.0,0.0,-0.5}});
latt->setSpan({{2.0,2.0,1.0}});
latt->build();
latt->setApply(true); // when chain executed, this will apply directly deformation onto the target mesh
/*
Create a reader for the displacement file associated to the lattice and
available from file
*/
/*
Define pin connections between blocks.
*/
/*
Define execution chain.
*/
mimmo::Chain ch0;
ch0.addObject(read);
ch0.addObject(latt);
ch0.addObject(iodispls);
/*
Execute the chain.
Use debug flag true to print out the execution steps.
*/
/*
print out the file the deformed geometry
*/
/* Clean up & exit;
*/
delete read;
delete iodispls;
delete latt;
}
// =================================================================================== //
int main( int argc, char *argv[] ) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI
MPI_Init(&argc, &argv);
#endif
try{
test00003();
}
catch(std::exception & e){
std::cout<<"genericinput_example_00003 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI
MPI_Finalize();
#endif
return 0;
}
@ CUBE
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setReadFilename(std::string filename)
Definition: GenericDispls.cpp:178
GenericDispls is the class to read from file an initial set of displacements as a generic vector fiel...
Definition: GenericDispls.hpp:107
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
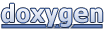