MimmoGeometry.hpp
32 BETTER_ENUM(FileType, int, STL = 0, SURFVTU = 1, VOLVTU = 2, NAS = 3, PCVTU = 4, CURVEVTU = 5, MIMMO = 99);
34 BETTER_ENUM(NastranElementType, int, GRID = 0, CTRIA = 1, CQUAD = 2, CBAR = 3, RBE2 = 4, RBE3 = 5);
271 void writeFace(std::string faceType, const livector1D& facePts, const long& nFace, std::ofstream& os, long PID);
272 bool writeGeometry(dvecarr3E &points,livector1D &pointsID, livector2D &faces, livector1D &facesID, std::ofstream &os, livector1D *PIDS = nullptr);
274 void write(std::string& outputDir, std::string& surfaceName, dvecarr3E& points, livector1D& pointsID, livector2D& faces, livector1D& facesID, livector1D* PIDS = nullptr, std::unordered_set<long>* PIDSSET = nullptr);
275 void read(std::string& inputDir, std::string& surfaceName, dvecarr3E& points, livector1D& pointsID, livector2D& faces, livector1D& facesID, livector1D& PIDS);
280 void appendLineRecords(std::string & sread, std::size_t recordlength, std::vector<std::string> & records);
281 void absorbRBE2(std::vector<std::string> & records, long & ID, long & PID, std::vector<long> & connectivity, std::string & components);
282 void absorbRBE3(std::vector<std::string> & records, long & ID, long & PID, std::vector<long> & connectivity, std::string & components);
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: MimmoGeometry.cpp:1004
FileDataInfo is a struct to stock data relative to names of external files.
Definition: MimmoNamespace.hpp:40
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setBuildSkdTree(bool build)
Definition: MimmoGeometry.cpp:466
void write(std::string &outputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D *PIDS=nullptr, std::unordered_set< long > *PIDSSET=nullptr)
Definition: MimmoGeometry.cpp:1561
void setReferencePID(long pid)
Definition: MimmoGeometry.cpp:456
void setBuildKdTree(bool build)
Definition: MimmoGeometry.cpp:475
void writeFace(std::string faceType, const livector1D &facePts, const long &nFace, std::ofstream &os, long PID)
Definition: MimmoGeometry.cpp:1391
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void writeKeyword(std::string key, std::ofstream &os)
Definition: MimmoGeometry.cpp:1306
bitpit::PiercedVector< bitpit::Vertex > * getVertices()
Definition: MimmoGeometry.cpp:418
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: MimmoGeometry.cpp:1209
void writeValue(Type &value, std::ofstream &os)
Definition: MimmoGeometry.hpp:298
void appendLineRecords(std::string &sread, std::size_t recordlength, std::vector< std::string > &records)
Definition: MimmoGeometry.cpp:1822
bool fileExist(const std::string &filename)
Definition: MimmoGeometry.cpp:957
bool isInteger(std::string &str)
Definition: MimmoGeometry.cpp:1918
void writeFooter(std::ofstream &os, std::unordered_set< long > *PIDSSET=nullptr)
Definition: MimmoGeometry.cpp:1502
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
void setMultiSolidSTL(bool multi=true)
Definition: MimmoGeometry.cpp:366
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void writeCoord(const darray3E &p, const long &pointI, std::ofstream &os)
Definition: MimmoGeometry.cpp:1330
std::string convertVertex(std::string in)
Definition: MimmoGeometry.cpp:1801
void absorbRBE3(std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components)
Definition: MimmoGeometry.cpp:1846
bool isEnabled(NastranElementType type)
Definition: MimmoGeometry.cpp:1932
void read(std::string &inputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D &PIDS)
Definition: MimmoGeometry.cpp:1587
void setParallelRestore(bool parallelRestore=0)
Definition: MimmoGeometry.cpp:395
void setFilename(std::string filename)
Definition: MimmoGeometry.cpp:324
MimmoGeometry(IOMode mode=IOMode::READ)
Definition: MimmoGeometry.cpp:40
NastranInterface is an interface class for I/O handling of BDF bulk nastran format *....
Definition: MimmoGeometry.hpp:258
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
void enable(NastranElementType type)
Definition: MimmoGeometry.cpp:1945
void absorbRBE2(std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components)
Definition: MimmoGeometry.cpp:1895
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
bitpit::PiercedVector< bitpit::Cell > * getCells()
Definition: MimmoGeometry.cpp:427
void disable(NastranElementType type)
Definition: MimmoGeometry.cpp:1954
bool writeGeometry(dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, std::ofstream &os, livector1D *PIDS=nullptr)
Definition: MimmoGeometry.cpp:1465
MimmoGeometry & operator=(MimmoGeometry other)
Definition: MimmoGeometry.cpp:105
std::map< NastranElementType, bool > m_enabled
Definition: MimmoGeometry.hpp:264
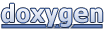