NastranInterface is an interface class for I/O handling of BDF bulk nastran format *.nas. More...
#include <MimmoGeometry.hpp>
Public Member Functions | |
NastranInterface () | |
void | absorbRBE2 (std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components) |
void | absorbRBE3 (std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components) |
void | appendLineRecords (std::string &sread, std::size_t recordlength, std::vector< std::string > &records) |
std::string | convertVertex (std::string in) |
bool | isEnabled (NastranElementType type) |
bool | isInteger (std::string &str) |
void | read (std::string &inputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D &PIDS) |
void | setWFormat (WFORMAT) |
std::string | trim (std::string in) |
void | write (std::string &outputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D *PIDS=nullptr, std::unordered_set< long > *PIDSSET=nullptr) |
void | writeCoord (const darray3E &p, const long &pointI, std::ofstream &os) |
void | writeFace (std::string faceType, const livector1D &facePts, const long &nFace, std::ofstream &os, long PID) |
void | writeFooter (std::ofstream &os, std::unordered_set< long > *PIDSSET=nullptr) |
bool | writeGeometry (dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, std::ofstream &os, livector1D *PIDS=nullptr) |
void | writeKeyword (std::string key, std::ofstream &os) |
Public Attributes | |
std::map< NastranElementType, bool > | m_enabled |
WFORMAT | m_wformat |
Protected Member Functions | |
void | disable (NastranElementType type) |
void | enable (NastranElementType type) |
template<class Type > | |
void | writeValue (Type &value, std::ofstream &os) |
Detailed Description
NastranInterface is an interface class for I/O handling of BDF bulk nastran format *.nas.
Definition at line 258 of file MimmoGeometry.hpp.
Constructor & Destructor Documentation
◆ NastranInterface()
mimmo::NastranInterface::NastranInterface | ( | ) |
Default constructor
Definition at line 1282 of file MimmoGeometry.cpp.
Member Function Documentation
◆ absorbRBE2()
void mimmo::NastranInterface::absorbRBE2 | ( | std::vector< std::string > & | records, |
long & | ID, | ||
long & | PID, | ||
std::vector< long > & | connectivity, | ||
std::string & | components | ||
) |
Absorb rbe2 element from a records structure
- Parameters
-
[in] records vector of string records [out] ID ID of the RBE2 element [out] PID PID of the RBE2 element [out] connectivity connectivity of the RBE2 element [out] components reaction components of the element
Definition at line 1895 of file MimmoGeometry.cpp.
◆ absorbRBE3()
void mimmo::NastranInterface::absorbRBE3 | ( | std::vector< std::string > & | records, |
long & | ID, | ||
long & | PID, | ||
std::vector< long > & | connectivity, | ||
std::string & | components | ||
) |
Absorb rbe3 element from a records structure
- Parameters
-
[in] records vector of string records [out] ID ID of the RBE3 element [out] PID PID of the RBE3 element [out] connectivity connectivity of the RBE3 element [out] components reaction components of the element
Definition at line 1846 of file MimmoGeometry.cpp.
◆ appendLineRecords()
void mimmo::NastranInterface::appendLineRecords | ( | std::string & | sread, |
std::size_t | recordlength, | ||
std::vector< std::string > & | records | ||
) |
Append records of given length of an input line in a records structure
- Parameters
-
[in] sread input line given as string [in] recordlength length of single record string [out] records vector of string records to be filled
Definition at line 1822 of file MimmoGeometry.cpp.
◆ convertVertex()
std::string mimmo::NastranInterface::convertVertex | ( | std::string | in | ) |
Convert nas string of numerical floats
- Returns
- string of regular floats
Definition at line 1801 of file MimmoGeometry.cpp.
◆ disable()
|
protected |
Disable an element type for the current read session
- Parameters
-
[in] type element type
Definition at line 1954 of file MimmoGeometry.cpp.
◆ enable()
|
protected |
Enable an element type for the current read session
- Parameters
-
[in] type element type
Definition at line 1945 of file MimmoGeometry.cpp.
◆ isEnabled()
bool mimmo::NastranInterface::isEnabled | ( | NastranElementType | type | ) |
Get if an element type is enbaled
- Parameters
-
[in] type element type
- Returns
- true if the element type is enbaled for the current read session
Definition at line 1932 of file MimmoGeometry.cpp.
◆ isInteger()
bool mimmo::NastranInterface::isInteger | ( | std::string & | str | ) |
Check if a string contains an integer
- Parameters
-
[in] str string to test
- Returns
- true if the string has only digits
Definition at line 1918 of file MimmoGeometry.cpp.
◆ read()
void mimmo::NastranInterface::read | ( | std::string & | inputDir, |
std::string & | surfaceName, | ||
dvecarr3E & | points, | ||
livector1D & | pointsID, | ||
livector2D & | faces, | ||
livector1D & | facesID, | ||
livector1D & | PIDS | ||
) |
Read a bdf nastran file
- Parameters
-
[in] inputDir input directory [in] surfaceName input filename [out] points reference of a point container that has to be filled [out] pointsID reference to a label point container to be filled [out] faces reference to element-point connectivity that has to be filled [out] facesID reference to a label element container to be filled [out] PIDS reference to long int vector for Part Identifier storage
Definition at line 1587 of file MimmoGeometry.cpp.
◆ setWFormat()
void mimmo::NastranInterface::setWFormat | ( | WFORMAT | wf | ) |
Set the format Short/Long of data in your nastran file
- Parameters
-
[in] wf WFORMAT Short/Long of your nastran file
Definition at line 1297 of file MimmoGeometry.cpp.
◆ trim()
std::string mimmo::NastranInterface::trim | ( | std::string | in | ) |
Custom trimming of nas string
- Returns
- trimmed string
Definition at line 1781 of file MimmoGeometry.cpp.
◆ write()
void mimmo::NastranInterface::write | ( | std::string & | outputDir, |
std::string & | surfaceName, | ||
dvecarr3E & | points, | ||
livector1D & | pointsID, | ||
livector2D & | faces, | ||
livector1D & | facesID, | ||
livector1D * | PIDS = nullptr , |
||
std::unordered_set< long > * | PIDSSET = nullptr |
||
) |
Write a bdf nastran file
- Parameters
-
[in] outputDir output directory [in] surfaceName output filename [in] points reference of a point container that has to be written [in] pointsID reference of a label point container [in] faces reference to element-point connectivity that has to be written [in] facesID reference to a label element container [in] PIDS reference to long int vector for Part Identifiers that need to be associated to elements [in] PIDSSET map of overall available Part Identifiers
Definition at line 1561 of file MimmoGeometry.cpp.
◆ writeCoord()
void mimmo::NastranInterface::writeCoord | ( | const darray3E & | p, |
const long & | pointI, | ||
std::ofstream & | os | ||
) |
Write a 3D point to an ofstream
- Parameters
-
[in] p point [in] pointI point label [in,out] os ofstream where the point is written
Definition at line 1330 of file MimmoGeometry.cpp.
◆ writeFace()
void mimmo::NastranInterface::writeFace | ( | std::string | faceType, |
const livector1D & | facePts, | ||
const long & | nFace, | ||
std::ofstream & | os, | ||
long | PID | ||
) |
Write a face to an ofstream
- Parameters
-
[in] faceType string reporting label fo the type of element [in] facePts Element Points connectivity [in] nFace element label [in,out] os ofstream where the face is written [in] PID part identifier associated to the element
Definition at line 1391 of file MimmoGeometry.cpp.
◆ writeFooter()
void mimmo::NastranInterface::writeFooter | ( | std::ofstream & | os, |
std::unordered_set< long > * | PIDSSET = nullptr |
||
) |
Write nastran footer to an ofstream
- Parameters
-
[in,out] os ofstream where the footer is written [in] PIDSSET list of overall available part identifiers
Definition at line 1502 of file MimmoGeometry.cpp.
◆ writeGeometry()
bool mimmo::NastranInterface::writeGeometry | ( | dvecarr3E & | points, |
livector1D & | pointsID, | ||
livector2D & | faces, | ||
livector1D & | facesID, | ||
std::ofstream & | os, | ||
livector1D * | PIDS = nullptr |
||
) |
Write a face to an std::ofstream
- Parameters
-
[in] points list of vertices [in] pointsID list of vertices labels [in] faces element points connectivity [in] facesID list of element labels [in,out] os ofstream where the geometry is written [in] PIDS list of Part Identifiers for each cells (optional)
- Returns
- true if the geometry is correctly written.
Definition at line 1465 of file MimmoGeometry.cpp.
◆ writeKeyword()
void mimmo::NastranInterface::writeKeyword | ( | std::string | key, |
std::ofstream & | os | ||
) |
Write a keyword string according to WFORMAT chosen by the User
- Parameters
-
[in] key keyword [in,out] os ofstream where the keyword is written
Definition at line 1306 of file MimmoGeometry.cpp.
◆ writeValue()
|
inlineprotected |
Write a template value according to WFORMAT chosen by the User
- Parameters
-
[in] value value of class Type [in,out] os ofstream where the value is written
Definition at line 298 of file MimmoGeometry.hpp.
Member Data Documentation
◆ m_enabled
std::map<NastranElementType, bool> mimmo::NastranInterface::m_enabled |
element type enabled for the current session
Definition at line 264 of file MimmoGeometry.hpp.
◆ m_wformat
WFORMAT mimmo::NastranInterface::m_wformat |
member storing the file type format Short/Long
Definition at line 262 of file MimmoGeometry.hpp.
The documentation for this class was generated from the following files:
- src/iogeneric/MimmoGeometry.hpp
- src/iogeneric/MimmoGeometry.cpp
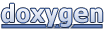