MimmoGeometry.cpp
139 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, MimmoGeometry>(this, &mimmo::BaseManipulation::setGeometry, M_GEOM, mandatory_input));
140 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, MimmoGeometry>(this, &mimmo::BaseManipulation::getGeometry, M_GEOM));
540 dynamic_cast<bitpit::SurfUnstructured*>(getGeometry()->getPatch())->exportSTL(name, m_codex, m_multiSolidSTL, &mpp);
614 nastran.write(m_winfo.fdir,namefile,points, pointsID, connectivity,elementsID, &pids, &pidsset);
633 // bitpit::VTKUnstructuredGrid vtk(m_winfo.fdir, m_winfo.fname, bitpit::VTKElementType::VERTEX);
711 dynamic_cast<bitpit::SurfUnstructured*>(getGeometry()->getPatch())->importSTL(name, binary, 0, false, &mapPIDSolid);
751 VTUGridReader input(m_rinfo.fdir, m_rinfo.fname, vtustreamer, *(getGeometry()->getPatch()), masterRankOnly);
782 VTUGridReader input(m_rinfo.fdir, m_rinfo.fname, vtustreamer, *(getGeometry()->getPatch()), masterRankOnly);
867 VTUGridReader input(m_rinfo.fdir, m_rinfo.fname, vtustreamer, *(getGeometry()->getPatch()), masterRankOnly);
897 VTUGridReader input(m_rinfo.fdir, m_rinfo.fname, vtustreamer, *(getGeometry()->getPatch()), masterRankOnly);
1391 void NastranInterface::writeFace(std::string faceType, const livector1D& facePts, const long& nFace, std::ofstream& os,long PID){
1465 bool NastranInterface::writeGeometry(dvecarr3E& points, livector1D& pointsID, livector2D& faces, livector1D & facesID,
1561 void NastranInterface::write(std::string& outputDir, std::string& surfaceName, dvecarr3E& points, livector1D & pointsID,
1570 throw std::runtime_error ("Error in NastranInterface::write : geometry mesh cannot be written");
1587 void NastranInterface::read(std::string& inputDir, std::string& surfaceName, dvecarr3E& points, livector1D & pointsID,
1822 NastranInterface::appendLineRecords(std::string & sread, std::size_t recordlength, std::vector<std::string> & records)
1829 // Do not append empty records or blank spaces or new line tag (ANSA format, starting with character '+')
1846 NastranInterface::absorbRBE3(std::vector<std::string> & records, long & ID, long & PID, std::vector<long> & connectivity, std::string & components)
1895 NastranInterface::absorbRBE2(std::vector<std::string> & records, long & ID, long & PID, std::vector<long> & connectivity, std::string & components)
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: MimmoGeometry.cpp:1004
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setBuildSkdTree(bool build)
Definition: MimmoGeometry.cpp:466
Custom reader of unstructured grids from external files *.vtu.
Definition: VTUGridReader.hpp:102
void write(std::string &outputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D *PIDS=nullptr, std::unordered_set< long > *PIDSSET=nullptr)
Definition: MimmoGeometry.cpp:1561
void setReferencePID(long pid)
Definition: MimmoGeometry.cpp:456
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void setBuildKdTree(bool build)
Definition: MimmoGeometry.cpp:475
void writeFace(std::string faceType, const livector1D &facePts, const long &nFace, std::ofstream &os, long PID)
Definition: MimmoGeometry.cpp:1391
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void writeKeyword(std::string key, std::ofstream &os)
Definition: MimmoGeometry.cpp:1306
bitpit::PiercedVector< bitpit::Vertex > * getVertices()
Definition: MimmoGeometry.cpp:418
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: MimmoGeometry.cpp:1209
void writeValue(Type &value, std::ofstream &os)
Definition: MimmoGeometry.hpp:298
void appendLineRecords(std::string &sread, std::size_t recordlength, std::vector< std::string > &records)
Definition: MimmoGeometry.cpp:1822
Custom writer of ASCII unstructured grids to external files *.vtu.
Definition: VTUGridWriterASCII.hpp:63
bool fileExist(const std::string &filename)
Definition: MimmoGeometry.cpp:957
bool isInteger(std::string &str)
Definition: MimmoGeometry.cpp:1918
void writeFooter(std::ofstream &os, std::unordered_set< long > *PIDSSET=nullptr)
Definition: MimmoGeometry.cpp:1502
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setMultiSolidSTL(bool multi=true)
Definition: MimmoGeometry.cpp:366
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void writeCoord(const darray3E &p, const long &pointI, std::ofstream &os)
Definition: MimmoGeometry.cpp:1330
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
std::string convertVertex(std::string in)
Definition: MimmoGeometry.cpp:1801
void absorbRBE3(std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components)
Definition: MimmoGeometry.cpp:1846
bool isEnabled(NastranElementType type)
Definition: MimmoGeometry.cpp:1932
void read(std::string &inputDir, std::string &surfaceName, dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, livector1D &PIDS)
Definition: MimmoGeometry.cpp:1587
void setParallelRestore(bool parallelRestore=0)
Definition: MimmoGeometry.cpp:395
Abstract class for custom ASCII writer/flusher of *.vtu mesh external files.
Definition: VTUGridWriterASCII.hpp:41
void setFilename(std::string filename)
Definition: MimmoGeometry.cpp:324
MimmoGeometry(IOMode mode=IOMode::READ)
Definition: MimmoGeometry.cpp:40
NastranInterface is an interface class for I/O handling of BDF bulk nastran format *....
Definition: MimmoGeometry.hpp:258
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
void enable(NastranElementType type)
Definition: MimmoGeometry.cpp:1945
void absorbRBE2(std::vector< std::string > &records, long &ID, long &PID, std::vector< long > &connectivity, std::string &components)
Definition: MimmoGeometry.cpp:1895
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
bitpit::PiercedVector< bitpit::Cell > * getCells()
Definition: MimmoGeometry.cpp:427
void disable(NastranElementType type)
Definition: MimmoGeometry.cpp:1954
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
MimmoSharedPointer< MimmoObject > & getGeometryReference()
Definition: BaseManipulation.cpp:245
void write(const std::string &dir, const std::string &file, bitpit::VTKWriteMode mode=bitpit::VTKWriteMode::DEFAULT)
Definition: VTUGridWriterASCII.cpp:320
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
bool writeGeometry(dvecarr3E &points, livector1D &pointsID, livector2D &faces, livector1D &facesID, std::ofstream &os, livector1D *PIDS=nullptr)
Definition: MimmoGeometry.cpp:1465
MimmoGeometry & operator=(MimmoGeometry other)
Definition: MimmoGeometry.cpp:105
std::map< NastranElementType, bool > m_enabled
Definition: MimmoGeometry.hpp:264
Custom mesh/data absorber for unstructured grids given by external files *.vtu.
Definition: VTUGridReader.hpp:63
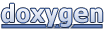