VTUGridReader.hpp
50 virtual void absorbData(std::fstream &stream, const std::string & name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype);
87 virtual void absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype);
89 void readIntegerPod(std::fstream & stream, bitpit::VTKFormat& format, bitpit::VTKDataType& datatype, long &target);
90 void readDoublePod(std::fstream & stream, bitpit::VTKFormat& format, bitpit::VTKDataType& datatype, double&target);
virtual void decodeRawData(bitpit::PatchKernel &)=0
Custom reader of unstructured grids from external files *.vtu.
Definition: VTUGridReader.hpp:102
Abstract class for custom reader/absorber of *.vtu mesh external files.
Definition: VTUGridReader.hpp:43
void readDoublePod(std::fstream &stream, bitpit::VTKFormat &format, bitpit::VTKDataType &datatype, double &target)
Definition: VTUGridReader.cpp:424
std::vector< bitpit::ElementType > types
Definition: VTUGridReader.hpp:72
virtual void absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype)
Definition: VTUGridReader.cpp:74
VTUGridReader(std::string dir, std::string name, VTUAbsorbStreamer &streamer, bitpit::PatchKernel &patch, bool masterRankOnly=true, bitpit::VTKElementType eltype=bitpit::VTKElementType::UNDEFINED)
Definition: VTUGridReader.cpp:466
virtual void absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype)
Definition: VTUGridReader.cpp:48
virtual ~VTUAbsorbStreamer()
Definition: VTUGridReader.cpp:37
void readIntegerPod(std::fstream &stream, bitpit::VTKFormat &format, bitpit::VTKDataType &datatype, long &target)
Definition: VTUGridReader.cpp:351
void decodeRawData(bitpit::PatchKernel &patch)
Definition: VTUGridReader.cpp:208
Custom mesh/data absorber for unstructured grids given by external files *.vtu.
Definition: VTUGridReader.hpp:63
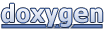