VTUGridReader.cpp
48 void VTUAbsorbStreamer::absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format,
74 void VTUGridStreamer::absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format,
216 throw std::runtime_error("Error VTUGridStreamer : no valid connectivity info detected while reading *.vtu file.");
219 //check for undefined cell types, if found throw a runtime error. Impossible to absorb completely the mesh.
222 std::vector<bitpit::ElementType>::iterator it = std::find(types.begin(), types.end(), bitpit::ElementType::UNDEFINED);
226 throw std::runtime_error("Error VTUGridStreamer : found unsupported cell elements. Impossible to absorb mesh");
232 // If for some reason you need them or bitpit change its behaviour, abilitate their reading in VTUGridReader constructor
290 throw std::runtime_error("Error VTUGridStreamer : trying to acquire POLYHEDRON info without faces and faceoffsets data");
300 int posfbegin = 1, posfend; //begin from 1- value. 0 value of conn contains the total number fo faces
351 VTUGridStreamer::readIntegerPod(std::fstream & stream, bitpit::VTKFormat& format, bitpit::VTKDataType& datatype, long &target){
412 throw std::runtime_error("VTUGridStreamer::absorbData :integer pod binary absorption, VTKDataType format unavailable");
424 VTUGridStreamer::readDoublePod(std::fstream & stream, bitpit::VTKFormat& format, bitpit::VTKDataType& datatype, double&target){
448 throw std::runtime_error("VTUGridStreamer::absorbData : double pod binary absorption, VTKDataType format unavailable");
466 VTUGridReader::VTUGridReader( std::string dir, std::string name, VTUAbsorbStreamer & streamer, bitpit::PatchKernel & patch, bool masterRankOnly, bitpit::VTKElementType eltype) :
474 addData<long>("vertexIndex", bitpit::VTKFieldType::SCALAR, bitpit::VTKLocation::POINT, &streamer);
482 // the reading of these 2 fields and provide a compliant implentation of VTUGridStreamer::decodeRawData
485 // addData<int>("vertexRank", bitpit::VTKFieldType::SCALAR, bitpit::VTKLocation::POINT, &streamer);
virtual void decodeRawData(bitpit::PatchKernel &)=0
Abstract class for custom reader/absorber of *.vtu mesh external files.
Definition: VTUGridReader.hpp:43
void readDoublePod(std::fstream &stream, bitpit::VTKFormat &format, bitpit::VTKDataType &datatype, double &target)
Definition: VTUGridReader.cpp:424
std::vector< bitpit::ElementType > types
Definition: VTUGridReader.hpp:72
virtual void absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype)
Definition: VTUGridReader.cpp:74
VTUGridReader(std::string dir, std::string name, VTUAbsorbStreamer &streamer, bitpit::PatchKernel &patch, bool masterRankOnly=true, bitpit::VTKElementType eltype=bitpit::VTKElementType::UNDEFINED)
Definition: VTUGridReader.cpp:466
virtual void absorbData(std::fstream &stream, const std::string &name, bitpit::VTKFormat format, uint64_t entries, uint8_t components, bitpit::VTKDataType datatype)
Definition: VTUGridReader.cpp:48
virtual ~VTUAbsorbStreamer()
Definition: VTUGridReader.cpp:37
void readIntegerPod(std::fstream &stream, bitpit::VTKFormat &format, bitpit::VTKDataType &datatype, long &target)
Definition: VTUGridReader.cpp:351
void decodeRawData(bitpit::PatchKernel &patch)
Definition: VTUGridReader.cpp:208
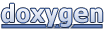