MimmoObject.hpp
190 MimmoObject(int type, dvecarr3E & vertex, livector2D * connectivity = nullptr, bool isParallel = MIMMO_ENABLE_MPI);
278 long addConnectedCell(const livector1D & locConn, bitpit::ElementType type, long idtag, int rank = -1);
279 long addConnectedCell(const livector1D & locConn, bitpit::ElementType type, long PID, long idtag, int rank = -1);
325 void getBoundingBox(std::array<double,3> & pmin, std::array<double,3> & pmax, bool global = true);
388 void degradeDegenerateElements(bitpit::PiercedVector<bitpit::Cell>* degradedDeletedCells = nullptr, bitpit::PiercedVector<bitpit::Vertex>* collapsedVertices = nullptr);
403 //make copy constructor and assignment private and not accessible (use properly clone method for copy)
livector1D extractBoundaryVertexID(std::unordered_map< long, std::set< int > > &map)
Definition: MimmoObject.cpp:2849
std::unordered_map< long, std::set< int > > getBorderFaceCells()
Definition: MimmoObject.cpp:3011
std::vector< std::vector< long > > decomposeLoop()
Definition: MimmoObject.cpp:3508
@ SYNC
std::set< long > findVertexVertexOneRing(const long &, const long &)
Definition: MimmoObject.cpp:4556
double evalInterfaceArea(const long &id)
Definition: MimmoObject.cpp:4653
MimmoVolUnstructured()
Definition: MimmoObject.cpp:146
SyncStatus
Define status of mimmo object structures like adjacencies, interfaces, etc.
Definition: MimmoObject.hpp:123
bitpit::PiercedVector< bitpit::Vertex > & getVertices()
Definition: MimmoObject.cpp:1177
bitpit::PiercedVector< double > getCellsNarrowBandToExtSurfaceWDist(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4131
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
Custom derivation of bitpit::VolUnstructured class.
Definition: MimmoObject.hpp:73
SyncStatus m_pointConnectivitySync
Definition: MimmoObject.hpp:186
bitpit::PiercedVector< bitpit::Interface > & getInterfaces()
Definition: MimmoObject.cpp:1322
SyncStatus getKdTreeSyncStatus()
Definition: MimmoObject.cpp:1626
dvecarr3E getVerticesCoords(lilimap *mapDataInv=nullptr)
Definition: MimmoObject.cpp:1136
bool setPIDName(long, const std::string &)
Definition: MimmoObject.cpp:2425
livector1D getVertexFromCellList(const livector1D &cellList)
Definition: MimmoObject.cpp:2557
MimmoObject(int type=1, bool isParallel=0)
Definition: MimmoObject.cpp:235
lilimap getMapCell(bool withghosts=true)
Definition: MimmoObject.cpp:1473
long addConnectedCell(const livector1D &locConn, bitpit::ElementType type, int rank=-1)
Definition: MimmoObject.cpp:2209
livector1D getCellsIds(bool internalsOnly=false)
Definition: MimmoObject.cpp:1345
livector1D getCellFromVertexList(const livector1D &vertList, bool strict=true)
Definition: MimmoObject.cpp:2633
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:219
long addVertex(const darray3E &vertex, const long idtag=bitpit::Vertex::NULL_ID)
Definition: MimmoObject.cpp:2120
bitpit::PatchNumberingInfo * getPatchInfo()
Definition: MimmoObject.cpp:1617
livector1D extractBoundaryInterfaceID(std::unordered_map< long, std::set< int > > &map)
Definition: MimmoObject.cpp:2890
bitpit::PiercedVector< bitpit::Cell > & getCells()
Definition: MimmoObject.cpp:1301
bool modifyVertex(const darray3E &vertex, const long &id)
Definition: MimmoObject.cpp:2188
lilimap getMapCellInv(bool withghosts=true)
Definition: MimmoObject.cpp:1494
std::unique_ptr< bitpit::PatchSkdTree > m_skdTree
Definition: MimmoObject.hpp:157
livector2D getCompactConnectivity(lilimap &mapDataInv)
Definition: MimmoObject.cpp:1204
void evalCellAspectRatio(bitpit::PiercedVector< double > &)
Definition: MimmoObject.cpp:3966
std::unique_ptr< bitpit::KdTree< 3, bitpit::Vertex, long > > m_kdTree
Definition: MimmoObject.hpp:158
livector1D getCellsNarrowBandToExtSurface(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4116
std::unordered_map< long, long > getInverseConnectivity()
Definition: MimmoObject.cpp:4532
void cleanPointConnectivity()
Definition: MimmoObject.cpp:4742
bitpit::PatchNumberingInfo m_patchInfo
Definition: MimmoObject.hpp:180
SyncStatus getAdjacenciesSyncStatus()
Definition: MimmoObject.cpp:3446
livector1D extractPIDCells(long, bool squeeze=true)
Definition: MimmoObject.cpp:3122
bitpit::KdTree< 3, bitpit::Vertex, long > * getKdTree()
Definition: MimmoObject.cpp:1634
livector1D getInterfaceFromVertexList(const livector1D &vertList, bool strict, bool border)
Definition: MimmoObject.cpp:2676
bitpit::ElementType desumeElement(const livector1D &)
Definition: MimmoObject.cpp:3676
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:110
std::array< double, 3 > evalInterfaceNormal(const long &id)
Definition: MimmoObject.cpp:4615
std::unordered_map< long, std::string > & getPIDTypeListWNames()
Definition: MimmoObject.cpp:1524
std::unordered_map< long, std::set< int > > extractBoundaryFaceCellID(bool ghost=false)
Definition: MimmoObject.cpp:2719
Custom derivation of bitpit::SurfUnstructured class, for Point Cloud handling only.
Definition: MimmoObject.hpp:99
SyncStatus getInterfacesSyncStatus()
Definition: MimmoObject.cpp:3460
std::unordered_set< int > elementsMap(bitpit::PatchKernel &obj)
Definition: MimmoObject.cpp:4096
void getBoundingBox(std::array< double, 3 > &pmin, std::array< double, 3 > &pmax, bool global=true)
Definition: MimmoObject.cpp:3256
MimmoSurfUnstructured()
Definition: MimmoObject.cpp:73
std::array< double, 3 > evalCellCentroid(const long &id)
Definition: MimmoObject.cpp:4584
livector1D getInterfaceFromCellList(const livector1D &cellList, bool all=true)
Definition: MimmoObject.cpp:2585
void degradeDegenerateElements(bitpit::PiercedVector< bitpit::Cell > *degradedDeletedCells=nullptr, bitpit::PiercedVector< bitpit::Vertex > *collapsedVertices=nullptr)
Definition: MimmoObject.cpp:4899
std::array< double, 3 > evalInterfaceCentroid(const long &id)
Definition: MimmoObject.cpp:4597
MimmoSharedPointer< MimmoObject > clone() const
Definition: MimmoObject.cpp:2463
std::unordered_map< long, std::string > m_pidsTypeWNames
Definition: MimmoObject.hpp:156
@ NONE
void buildSkdTree(std::size_t value=1)
Definition: MimmoObject.cpp:3265
SyncStatus getBoundingBoxSyncStatus()
Definition: MimmoObject.cpp:1601
std::unordered_set< long > & getPIDTypeList()
Definition: MimmoObject.cpp:1515
std::map< long, livector1D > extractPIDSubdivision()
Definition: MimmoObject.cpp:3163
livector1D getCellConnectivity(long id) const
Definition: MimmoObject.cpp:1281
SyncStatus getSkdTreeSyncStatus()
Definition: MimmoObject.cpp:1583
std::unordered_set< long > & getPointConnectivity(const long &id)
Definition: MimmoObject.cpp:4757
long addCell(bitpit::Cell &cell, int rank=-1)
Definition: MimmoObject.cpp:2291
livector1D getVerticesIds(bool internalsOnly=false)
Definition: MimmoObject.cpp:1376
void evalCellVolumes(bitpit::PiercedVector< double > &)
Definition: MimmoObject.cpp:3926
@ UNSYNC
SyncStatus getPointConnectivitySyncStatus()
Definition: MimmoObject.cpp:4767
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:175
livector1D getVerticesNarrowBandToExtSurface(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4323
lilimap getMapData(bool withghosts=false)
Definition: MimmoObject.cpp:1423
livector1D extractBoundaryCellID(bool ghost=false)
Definition: MimmoObject.cpp:2837
Custom derivation of bitpit::SurfUnstructured class.
Definition: MimmoObject.hpp:45
std::unordered_map< long, std::unordered_set< long > > m_pointConnectivity
Definition: MimmoObject.hpp:185
virtual ~MimmoSurfUnstructured()
Definition: MimmoObject.cpp:103
MimmoSharedPointer< MimmoObject > extractBoundaryMesh()
Definition: MimmoObject.cpp:2934
bitpit::PiercedVector< double > getVerticesNarrowBandToExtSurfaceWDist(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4339
void buildPointConnectivity()
Definition: MimmoObject.cpp:4681
double evalCellVolume(const long &id)
Definition: MimmoObject.cpp:4032
lilimap getMapDataInv(bool withghosts=true)
Definition: MimmoObject.cpp:1441
const darray3E & getVertexCoords(long i) const
Definition: MimmoObject.cpp:1165
@ NOTSUPPORTED
virtual ~MimmoVolUnstructured()
Definition: MimmoObject.cpp:168
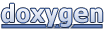