MimmoObject is the basic geometry container for mimmo library. More...
#include <MimmoObject.hpp>
Public Member Functions | |
MimmoObject (int type, bitpit::PatchKernel *geometry) | |
MimmoObject (int type, dvecarr3E &vertex, livector2D *connectivity=nullptr, bool isParallel=0) | |
MimmoObject (int type, std::unique_ptr< bitpit::PatchKernel > &geometry) | |
MimmoObject (int type=1, bool isParallel=0) | |
~MimmoObject () | |
long | addCell (bitpit::Cell &cell, int rank=-1) |
long | addCell (bitpit::Cell &cell, long idtag, int rank=-1) |
long | addConnectedCell (const livector1D &locConn, bitpit::ElementType type, int rank=-1) |
long | addConnectedCell (const livector1D &locConn, bitpit::ElementType type, long idtag, int rank=-1) |
long | addConnectedCell (const livector1D &locConn, bitpit::ElementType type, long PID, long idtag, int rank=-1) |
long | addVertex (const bitpit::Vertex &vertex, const long idtag=bitpit::Vertex::NULL_ID) |
long | addVertex (const darray3E &vertex, const long idtag=bitpit::Vertex::NULL_ID) |
void | buildKdTree () |
void | buildPatchInfo () |
void | buildPointConnectivity () |
void | buildSkdTree (std::size_t value=1) |
void | cleanBoundingBox () |
bool | cleanGeometry () |
void | cleanKdTree () |
void | cleanPatchInfo () |
void | cleanPointConnectivity () |
void | cleanSkdTree () |
MimmoSharedPointer< MimmoObject > | clone () const |
std::vector< std::vector< long > > | decomposeLoop () |
void | degradeDegenerateElements (bitpit::PiercedVector< bitpit::Cell > *degradedDeletedCells=nullptr, bitpit::PiercedVector< bitpit::Vertex > *collapsedVertices=nullptr) |
void | destroyAdjacencies () |
void | destroyInterfaces () |
bitpit::ElementType | desumeElement (const livector1D &) |
void | dump (std::ostream &stream) |
void | evalCellAspectRatio (bitpit::PiercedVector< double > &) |
double | evalCellAspectRatio (const long &id) |
std::array< double, 3 > | evalCellCentroid (const long &id) |
double | evalCellVolume (const long &id) |
void | evalCellVolumes (bitpit::PiercedVector< double > &) |
double | evalInterfaceArea (const long &id) |
std::array< double, 3 > | evalInterfaceCentroid (const long &id) |
std::array< double, 3 > | evalInterfaceNormal (const long &id) |
livector1D | extractBoundaryCellID (bool ghost=false) |
livector1D | extractBoundaryCellID (std::unordered_map< long, std::set< int > > &map) |
std::unordered_map< long, std::set< int > > | extractBoundaryFaceCellID (bool ghost=false) |
livector1D | extractBoundaryInterfaceID (bool ghost=false) |
livector1D | extractBoundaryInterfaceID (std::unordered_map< long, std::set< int > > &map) |
MimmoSharedPointer< MimmoObject > | extractBoundaryMesh () |
livector1D | extractBoundaryVertexID (bool ghost=false) |
livector1D | extractBoundaryVertexID (std::unordered_map< long, std::set< int > > &map) |
livector1D | extractPIDCells (livector1D, bool squeeze=true) |
livector1D | extractPIDCells (long, bool squeeze=true) |
std::map< long, livector1D > | extractPIDSubdivision () |
std::set< long > | findVertexVertexOneRing (const long &, const long &) |
SyncStatus | getAdjacenciesSyncStatus () |
livector1D | getBorderCells () |
livector1D | getBorderCells (std::unordered_map< long, std::set< int > > &map) |
std::unordered_map< long, std::set< int > > | getBorderFaceCells () |
livector1D | getBorderVertices () |
livector1D | getBorderVertices (std::unordered_map< long, std::set< int > > &map) |
void | getBoundingBox (std::array< double, 3 > &pmin, std::array< double, 3 > &pmax, bool global=true) |
SyncStatus | getBoundingBoxSyncStatus () |
livector1D | getCellConnectivity (long id) const |
livector1D | getCellFromVertexList (const livector1D &vertList, bool strict=true) |
bitpit::PiercedVector< bitpit::Cell > & | getCells () |
const bitpit::PiercedVector< bitpit::Cell > & | getCells () const |
livector1D | getCellsIds (bool internalsOnly=false) |
livector1D | getCellsNarrowBandToExtSurface (MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr) |
bitpit::PiercedVector< double > | getCellsNarrowBandToExtSurfaceWDist (MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr) |
livector2D | getCompactConnectivity (lilimap &mapDataInv) |
livector1D | getCompactPID () |
livector2D | getConnectivity () |
SyncStatus | getInfoSyncStatus () |
livector1D | getInterfaceFromCellList (const livector1D &cellList, bool all=true) |
livector1D | getInterfaceFromVertexList (const livector1D &vertList, bool strict, bool border) |
bitpit::PiercedVector< bitpit::Interface > & | getInterfaces () |
const bitpit::PiercedVector< bitpit::Interface > & | getInterfaces () const |
SyncStatus | getInterfacesSyncStatus () |
std::unordered_map< long, long > | getInverseConnectivity () |
bitpit::KdTree< 3, bitpit::Vertex, long > * | getKdTree () |
SyncStatus | getKdTreeSyncStatus () |
bitpit::Logger & | getLog () |
lilimap | getMapCell (bool withghosts=true) |
lilimap | getMapCellInv (bool withghosts=true) |
lilimap | getMapData (bool withghosts=false) |
lilimap | getMapDataInv (bool withghosts=true) |
long | getNCells () |
long | getNCells () const |
long | getNInternals () const |
long | getNInternalVertices () |
long | getNVertices () |
long | getNVertices () const |
bitpit::PatchKernel * | getPatch () |
const bitpit::PatchKernel * | getPatch () const |
bitpit::PatchNumberingInfo * | getPatchInfo () |
std::unordered_map< long, long > | getPID () |
std::unordered_set< long > & | getPIDTypeList () |
const std::unordered_set< long > & | getPIDTypeList () const |
std::unordered_map< long, std::string > & | getPIDTypeListWNames () |
const std::unordered_map< long, std::string > & | getPIDTypeListWNames () const |
std::unordered_set< long > & | getPointConnectivity (const long &id) |
SyncStatus | getPointConnectivitySyncStatus () |
int | getProcessorCount () const |
int | getRank () const |
bitpit::PatchSkdTree * | getSkdTree () |
SyncStatus | getSkdTreeSyncStatus () |
double | getTolerance () |
int | getType () |
const darray3E & | getVertexCoords (long i) const |
livector1D | getVertexFromCellList (const livector1D &cellList) |
bitpit::PiercedVector< bitpit::Vertex > & | getVertices () |
const bitpit::PiercedVector< bitpit::Vertex > & | getVertices () const |
dvecarr3E | getVerticesCoords (lilimap *mapDataInv=nullptr) |
livector1D | getVerticesIds (bool internalsOnly=false) |
livector1D | getVerticesNarrowBandToExtSurface (MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr) |
bitpit::PiercedVector< double > | getVerticesNarrowBandToExtSurfaceWDist (MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr) |
bool | isClosedLoop () |
bool | isEmpty () |
bool | isParallel () |
bool | isPointInterior (long id) |
bool | isSkdTreeSupported () |
bool | modifyVertex (const darray3E &vertex, const long &id) |
void | resetPatch () |
void | restore (std::istream &stream) |
void | resyncPID () |
void | setPID (livector1D) |
void | setPID (std::unordered_map< long, long >) |
void | setPIDCell (long, long) |
bool | setPIDName (long, const std::string &) |
void | setTolerance (double tol) |
void | setUnsyncAll () |
void | swap (MimmoObject &) noexcept |
void | triangulate () |
void | update () |
void | updateAdjacencies () |
void | updateInterfaces () |
Protected Member Functions | |
std::unordered_set< int > | elementsMap (bitpit::PatchKernel &obj) |
void | initializeLogger () |
void | reset (int type, bool isParallel=0) |
Protected Attributes | |
SyncStatus | m_AdjSync |
SyncStatus | m_boundingBoxSync |
SyncStatus | m_infoSync |
SyncStatus | m_IntSync |
bool | m_isParallel |
std::unique_ptr< bitpit::KdTree< 3, bitpit::Vertex, long > > | m_kdTree |
SyncStatus | m_kdTreeSync |
bitpit::Logger * | m_log |
int | m_nprocs |
bitpit::PatchNumberingInfo | m_patchInfo |
std::unordered_set< long > | m_pidsType |
std::unordered_map< long, std::string > | m_pidsTypeWNames |
std::unordered_map< long, std::unordered_set< long > > | m_pointConnectivity |
SyncStatus | m_pointConnectivitySync |
int | m_rank |
std::unique_ptr< bitpit::PatchSkdTree > | m_skdTree |
SyncStatus | m_skdTreeSync |
int | m_type |
Detailed Description
MimmoObject is the basic geometry container for mimmo library.
MimmoObject is the basic container for 3D unustructured mesh data structure, based on bitpit::PatchKernel containers. MimmoObject can handle unustructured surface meshes, unustructured volume meshes, 3D point clouds and 3D tessellated curves. It supports interface methods to explore and handle the geometrical structure. It supports PID convention to mark subparts of geometry as well as building the search-trees KdTree (3D point spatial ordering) and skdTree(Cell-AABB spatial ordering) to quickly retrieve vertices and cells in the data structure.
- Examples
- geohandlers_example_00004.cpp, test_propagators_00001.cpp, test_propagators_00002.cpp, test_propagators_00003.cpp, and utils_example_00003.cpp.
Definition at line 143 of file MimmoObject.hpp.
Constructor & Destructor Documentation
◆ MimmoObject() [1/4]
mimmo::MimmoObject::MimmoObject | ( | int | type = 1 , |
bool | isParallel = 0 |
||
) |
Default constructor of MimmoObject. It requires a int flag identifying the type of mesh meant to be created:
- surface unstructured mesh = 1
- volume unstructured mesh = 2
- 3D Cloud Point = 3
- 3D tessellated Curve = 4
- Parameters
-
[in] type type of mesh [in] isParallel if true construct a parallel MimmoObject (if MPI not enabled forced to false)
Definition at line 235 of file MimmoObject.cpp.
◆ MimmoObject() [2/4]
mimmo::MimmoObject::MimmoObject | ( | int | type, |
dvecarr3E & | vertex, | ||
livector2D * | connectivity = nullptr , |
||
bool | isParallel = 0 |
||
) |
Custom constructor of MimmoObject. This constructor builds a generic mesh from given vertex list and its related local connectivity. Mesh-element details are desumed by local cell-vertices connectivity size. Connectivities supported can be of any kind. Mixed elements are allowed. Cell element types supported are all those of bitpit::ElementType list. In particular:
- any 2D cell for surface meshes
- any 3D cell for volume meshes
- VERTEX only for 3D point clouds
- LINE cells only for 3D curves
Cloud points are always supported (no connectivity field must be provided) Type of meshes supported are described in the default MimmoObject constructor documentation. Please note, despite type argument, if null connectivity structure is provided, MimmoObject will be builtas a standard cloud point of vertices provided by vertex. Connectivity for each standard cell is simply defined as a list of vertex indices which compose it (indices are meant as positions in the provided vertex list argument). Polygonal and Polyhedral cells requires a special convention to define their connectivity:
- polygons: require on top of the list the total number of vertices which compose it,followed by the indices in the vertex list (ex. polygon with 5 vertices: 5 i1 i2 i3 i4 i5).
- polyhedra: require on top the total number of faces, followed by the number of vertices which composes the local face + the vertex indices which compose the faces, and so on for all the faces which compose the polyhedron (ex. polyhedron with 3 faces, first face is a triangle, second is a quad etc... 3 3 i1 i2 i3 4 i2 i3 i4 i5 ...)
- Parameters
-
[in] type type of meshes. [in] vertex Coordinates of geometry vertices. [in] connectivity pointer to mesh connectivity list (optional). [in] isParallel if true construct a parallel MimmoObject (if MPI not enabled forced to false)
Definition at line 351 of file MimmoObject.cpp.
◆ MimmoObject() [3/4]
mimmo::MimmoObject::MimmoObject | ( | int | type, |
bitpit::PatchKernel * | geometry | ||
) |
Custom constructor of MimmoObject. This constructor builds a geometry data structure soft linking to an external bitpit::PatchKernel object, i.e. it does not own the geometry data structure, but simple access it, while it is instantiated elsewhere. If a null or empty or not coherent geometry patch is linked, throw an error exception.
- Parameters
-
[in] type type of mesh [in] geometry pointer to a geometry of class PatchKernel to be linked.
Definition at line 469 of file MimmoObject.cpp.
◆ MimmoObject() [4/4]
mimmo::MimmoObject::MimmoObject | ( | int | type, |
std::unique_ptr< bitpit::PatchKernel > & | geometry | ||
) |
Custom constructor of MimmoObject. This constructor builds a geometry data structure owning an external bitpit::PatchKernel object, i.e.it takes the ownership of the geometry data structure treating it as an internal structure, and it will be responsible of its own destruction. If a null or empty or not coherent geometry patch is linked, throw an error exception.
- Parameters
-
[in] type type of mesh [in] geometry unique pointer to a geometry of class PatchKernel to be linked.
Definition at line 625 of file MimmoObject.cpp.
◆ ~MimmoObject()
mimmo::MimmoObject::~MimmoObject | ( | ) |
Default destructor
Definition at line 772 of file MimmoObject.cpp.
Member Function Documentation
◆ addCell() [1/2]
long mimmo::MimmoObject::addCell | ( | bitpit::Cell & | cell, |
int | rank = -1 |
||
) |
Same as MimmoObject::addCell(bitpit::Cell & cell, const long idtag, int rank), but using id automatic assignment.
- Returns
- id of the inserted cell. return bitpit::Cell::NULL_ID if failed.
Definition at line 2291 of file MimmoObject.cpp.
◆ addCell() [2/2]
long mimmo::MimmoObject::addCell | ( | bitpit::Cell & | cell, |
long | idtag, | ||
int | rank = -1 |
||
) |
It adds one cell to the mesh. If unique-id is specified for the cell, assign it, otherwise provide itself to get a unique-id for the added cell. The latter option is the default. If the unique-id is already assigned, return with unsuccessful insertion. PAY ATTENTION if the cell have adjacencies and interfaces built, they are not copied to not mess with local PatchKernel and MimmoObject Adjacencies and Interfaces syncronization.
FOR MPI version: the method add cell with the custom rank provided. If rank < 0, use local m_rank of MimmoObject.
- Parameters
-
[in] cell cell to be added [in] idtag unique id associated to the cell [in] rank belonging proc rank of the Cell (for MPI version only)
- Returns
- id of the inserted cell. return bitpit::Cell::NULL_ID if failed.
Definition at line 2311 of file MimmoObject.cpp.
◆ addConnectedCell() [1/3]
long mimmo::MimmoObject::addConnectedCell | ( | const livector1D & | conn, |
bitpit::ElementType | type, | ||
int | rank = -1 |
||
) |
See method addConnectedCell(const livector1D & conn, bitpit::ElementType type, long PID, long idtag, int rank) doxy. The only difference is the automatic assignment to PID= 0 for the current element and the automatic assignment of ID.
- Returns
- id of the inserted cell. return bitpit::Cell::NULL_ID if failed.
Definition at line 2209 of file MimmoObject.cpp.
◆ addConnectedCell() [2/3]
long mimmo::MimmoObject::addConnectedCell | ( | const livector1D & | conn, |
bitpit::ElementType | type, | ||
long | idtag, | ||
int | rank = -1 |
||
) |
See method addConnectedCell(const livector1D & conn, bitpit::ElementType type, long PID, long idtag, int rank) doxy. The only difference is the automatic assignment to PID= 0 for the current element.
- Returns
- id of the inserted cell. return bitpit::Cell::NULL_ID if failed.
Definition at line 2219 of file MimmoObject.cpp.
◆ addConnectedCell() [3/3]
long mimmo::MimmoObject::addConnectedCell | ( | const livector1D & | conn, |
bitpit::ElementType | type, | ||
long | PID, | ||
long | idtag, | ||
int | rank = -1 |
||
) |
It adds one cell with its vertex-connectivity (vertex in bitpit::PatchKernel unique id's), the type of cell to be added and its own unique id. If no id is specified, teh method assigns it automatically. Cell type and connectivity dimension of the cell are cross-checked with the mesh type of the class: if mismatch, the method does not add the cell and return false. If class type is a pointcloud one (type 3) the method creates cell of type bitpit::Vertex A part identifier PID mark can be associated to the cell.
As a reminder for connectivity conn argument:
- Connectivity of polygons must be defined as (nV,V1,V2,V3,V4,...) where nV is the number of vertices defining the polygon and V1,V2,V3... are indices of vertices.
- Connectivity of polyhedrons must be defined as (nF,nF1V, V1, V2, V3,..., nF2V, V2,V4,V5,V6,...,....) where nF is the total number of faces of polyhedros, nF1V is the number of vertices composing the face 1, followed by the indices of vertices which draws it. Face 2,3,..,n are defined in the same way.
- Any other standard cell element is uniquely defined by its list of vertex indices.
FOR MPI version: the method add cell with the custom rank provided. If rank < 0, use local m_rank of current MimmoObject.
- Parameters
-
[in] conn connectivity of target cell of geometry mesh. [in] type type of element to be added, according to bitpit ElementInfo enum. [in] PID part identifier [in] idtag id of the cell [in] rank belonging proc rank of the Cell (for MPI version only)
- Returns
- id of the inserted cell. return bitpit::Cell::NULL_ID if failed.
Definition at line 2249 of file MimmoObject.cpp.
◆ addVertex() [1/2]
long mimmo::MimmoObject::addVertex | ( | const bitpit::Vertex & | vertex, |
const long | idtag = bitpit::Vertex::NULL_ID |
||
) |
It adds one vertex to the mesh. If unique-id is specified for the vertex, assign it, otherwise provide itself to get a unique-id for the added vertex. The latter option is the default. If the unique-id is already assigned, return with unsuccessful insertion.
- Parameters
-
[in] vertex vertex to be added [in] idtag unique id associated to the vertex
- Returns
- id of the inserted vertex. return bitpit::Vertex::NULL_ID if failed.
Definition at line 2156 of file MimmoObject.cpp.
◆ addVertex() [2/2]
long mimmo::MimmoObject::addVertex | ( | const darray3E & | vertex, |
const long | idtag = bitpit::Vertex::NULL_ID |
||
) |
It adds one vertex to the mesh. If unique-id is specified for the vertex, assign it, otherwise provide itself to get a unique-id for the added vertex. The latter option is the default. If the unique-id is already assigned, return with unsuccessful insertion. Vertices of ghost cells have to be considered.
- Parameters
-
[in] vertex coordinates of the vertex to be added [in] idtag unique id associated to the vertex
- Returns
- id of the inserted vertex. return bitpit::Vertex::NULL_ID if failed.
Definition at line 2120 of file MimmoObject.cpp.
◆ buildKdTree()
void mimmo::MimmoObject::buildKdTree | ( | ) |
Reset and build again vertex kdTree of your geometry. Nodes fo ghost cells are insert in the tree.
Definition at line 3280 of file MimmoObject.cpp.
◆ buildPatchInfo()
void mimmo::MimmoObject::buildPatchInfo | ( | ) |
Build cell patch numbering info of your geometry.
Definition at line 3324 of file MimmoObject.cpp.
◆ buildPointConnectivity()
void mimmo::MimmoObject::buildPointConnectivity | ( | ) |
Build the Node-Node connectivity of the tessellated mesh,(nodes connected by edges) and store it internally.
Definition at line 4681 of file MimmoObject.cpp.
◆ buildSkdTree()
void mimmo::MimmoObject::buildSkdTree | ( | std::size_t | value = 1 | ) |
Reset and build again cell skdTree of your geometry (if supports connectivity elements). Ghost cells are insert in the tree.
- Parameters
-
[in] value build the minimum leaf of the tree as a bounding box containing value elements at most.
Definition at line 3265 of file MimmoObject.cpp.
◆ cleanBoundingBox()
void mimmo::MimmoObject::cleanBoundingBox | ( | ) |
Clean bounding box of your geometry (i.e. currently set to SyncStatus::NONE the synchronization status).
Definition at line 3348 of file MimmoObject.cpp.
◆ cleanGeometry()
bool mimmo::MimmoObject::cleanGeometry | ( | ) |
It finds and cleans duplicated vertices and, in case of connected tessellations, all orphan/isolated vertices. In case of active cleaning, unsync all MimmoObject structures. In MPI version this call is collective among all ranks.
- Returns
- false if no activity cleaning is performed, true otherwise.
Definition at line 2507 of file MimmoObject.cpp.
◆ cleanKdTree()
void mimmo::MimmoObject::cleanKdTree | ( | ) |
Clean the KdTree of the class
Definition at line 3301 of file MimmoObject.cpp.
◆ cleanPatchInfo()
void mimmo::MimmoObject::cleanPatchInfo | ( | ) |
Reset cell patch numbering info of your geometry.
Definition at line 3336 of file MimmoObject.cpp.
◆ cleanPointConnectivity()
void mimmo::MimmoObject::cleanPointConnectivity | ( | ) |
Clean the point connectivity structure.
Definition at line 4742 of file MimmoObject.cpp.
◆ cleanSkdTree()
void mimmo::MimmoObject::cleanSkdTree | ( | ) |
Clean the SkdTree of the class
Definition at line 3312 of file MimmoObject.cpp.
◆ clone()
MimmoSharedPointer< MimmoObject > mimmo::MimmoObject::clone | ( | ) | const |
Clone your MimmoObject in a new indipendent MimmoObject. All data of the current class will be "hard" copied in a new MimmoObject class. Hard means that the geometry data structure will be allocated internally into the new object as an exact and stand-alone copy of the geometry data structure of the current class, indipendently on how the current class owns or links it.
- Returns
- MimmoSharedPointer to cloned MimmoObject.
Definition at line 2463 of file MimmoObject.cpp.
◆ decomposeLoop()
livector2D mimmo::MimmoObject::decomposeLoop | ( | ) |
If the mesh is composed by multiple unconnected patches or triage patches, the method returns the cellID list belonging to each subpatches
- Returns
- list of cellIDs set for each subpatch
Definition at line 3508 of file MimmoObject.cpp.
◆ degradeDegenerateElements()
void mimmo::MimmoObject::degradeDegenerateElements | ( | bitpit::PiercedVector< bitpit::Cell > * | degradedDeletedCells = nullptr , |
bitpit::PiercedVector< bitpit::Vertex > * | collapsedVertices = nullptr |
||
) |
Check the correctness elements of the geometry. Find degenerate elements in terms of vertices, i.e. an element is considered degenerate if at least two vertices are equal. If a degenerate element is found, it is degraded to a valid inferior element preserving the same cell id, e.g a degenerate QUAD is degraded to a TRIANGLE, if only one vertex is repeated. If a degraded element is invalid (e.g. a QUAD in a threedimensional mesh) the cell is deleted.
- Parameters
-
[out] degradedDeletedCells If not a null pointer the pointed vector is filled with the original deleted or degraded cells [out] collapsedVertices If not a null pointer the pointed vector is filled with the original collapsed (i.e. deleted) vertices
Definition at line 4899 of file MimmoObject.cpp.
◆ destroyAdjacencies()
void mimmo::MimmoObject::destroyAdjacencies | ( | ) |
Destroy cell-cell adjacency connectivity.
Definition at line 3630 of file MimmoObject.cpp.
◆ destroyInterfaces()
void mimmo::MimmoObject::destroyInterfaces | ( | ) |
Destroy Interfaces connectivity.
Definition at line 3638 of file MimmoObject.cpp.
◆ desumeElement()
bitpit::ElementType mimmo::MimmoObject::desumeElement | ( | const livector1D & | locConn | ) |
Desume Element given the vertex connectivity list associated. Polygons and Polyhedra require a special writing for their connectivity list. Please read doxy of MimmoObject(int type, dvecarr3E & vertex, livector2D * connectivity = nullptr) custom constructor for further information. Return undefined type for unexistent or unsupported element.
- Parameters
-
[in] locConn list of vertex indices composing the cell.
- Returns
- cell type hold by the current connectivity argument.
Definition at line 3676 of file MimmoObject.cpp.
◆ dump()
void mimmo::MimmoObject::dump | ( | std::ostream & | stream | ) |
Dump contents of your current MimmoObject to a stream. Dump all strict necessary information except for search tree structure, Point ghost exchange info and point connectivity are recreated when restores. Write all in a binary format.
- Parameters
-
[in,out] stream to write on.
Definition at line 3818 of file MimmoObject.cpp.
◆ elementsMap()
|
protected |
- Returns
- map of all bitpit::ElementType (casted as integers) hold by the current bitpit::PatchKernel obj. Ghost cells are considered.
- Parameters
-
[in] obj target PatchKernel object.
Definition at line 4096 of file MimmoObject.cpp.
◆ evalCellAspectRatio() [1/2]
void mimmo::MimmoObject::evalCellAspectRatio | ( | bitpit::PiercedVector< double > & | ARs | ) |
Evaluate Aspect Ratio of each cell in the current local mesh, according to its topology. VERTEX and LINE elements does not support a proper definition of Aspect Ratio and will return always 0.0 as value. PointCloud and 3DCurve MimmoObject return an empty map. If no patch or empty patch or pointcloud patch is present in the current MimmoObject return empty map as result. Ghost cells are considered.
- Parameters
-
[out] ARs aspect ratio values referred to cell id
Definition at line 3966 of file MimmoObject.cpp.
◆ evalCellAspectRatio() [2/2]
double mimmo::MimmoObject::evalCellAspectRatio | ( | const long & | id | ) |
Evaluate Aspect Ratio of a target local cell. VERTEX and LINE elements does not support a proper definition of Aspect Ratio and will return always 0.0 as value.
- Parameters
-
[in] id of the cell
- Returns
- value of cell AR.
Definition at line 4055 of file MimmoObject.cpp.
◆ evalCellCentroid()
std::array< double, 3 > mimmo::MimmoObject::evalCellCentroid | ( | const long & | id | ) |
Evaluate the centroid of the target cell.
- Parameters
-
[in] id of cell, no control if cell exists is done
- Returns
- cell centroid coordinate
Definition at line 4584 of file MimmoObject.cpp.
◆ evalCellVolume()
double mimmo::MimmoObject::evalCellVolume | ( | const long & | id | ) |
Evaluate volume of a target cell in the current local mesh.
- Parameters
-
[in] id cell id.
- Returns
- cell volume
Definition at line 4032 of file MimmoObject.cpp.
◆ evalCellVolumes()
void mimmo::MimmoObject::evalCellVolumes | ( | bitpit::PiercedVector< double > & | volumes | ) |
Evaluate general volume of each cell in the current local mesh, according to its topology. If no patch or empty patch or pointcloud patch is present in the current MimmoObject return empty map as result. Ghost cells are considered.
- Parameters
-
[out] volumes volume values referred to cell ids
Definition at line 3926 of file MimmoObject.cpp.
◆ evalInterfaceArea()
double mimmo::MimmoObject::evalInterfaceArea | ( | const long & | id | ) |
Evaluate the area/lenght of the target interface. Need to have interface built. The method return zero area for Point Cloud, 3D-Curve and Undefined mimmoObject meshes. If volume mesh return the area of the the 2D Element face. if surface mesh return the lenght of the edge in common between owner and neighbour cells.
- Parameters
-
[in] id of interface,no control if interface exists is done
- Returns
- value of interface area/length.
Definition at line 4653 of file MimmoObject.cpp.
◆ evalInterfaceCentroid()
std::array< double, 3 > mimmo::MimmoObject::evalInterfaceCentroid | ( | const long & | id | ) |
Evaluate the centroid of the target interface. Need to have interface built.
- Parameters
-
[in] id of interface, no control if interface exists is done
- Returns
- interface centroid coordinate
Definition at line 4597 of file MimmoObject.cpp.
◆ evalInterfaceNormal()
std::array< double, 3 > mimmo::MimmoObject::evalInterfaceNormal | ( | const long & | id | ) |
Evaluate the normal of the target interface, in the sense of owner-neighbour cell. Need to have interface built. The method return zero normal for Point Cloud, 3D-Curve and Undefined mimmoObject meshes. If volume mesh return the normal to the face in common between owner and neighbour cells and directed from the owner to the neighbor. if surface mesh return the normal to the edge in common between owner and neighbour cells and directed from the owner to the neighbor.
- Parameters
-
[in] id of interface,no control if interface exists is done
- Returns
- normal to interface
Definition at line 4615 of file MimmoObject.cpp.
◆ extractBoundaryCellID() [1/2]
livector1D mimmo::MimmoObject::extractBoundaryCellID | ( | bool | ghost = false | ) |
Extract cells who have one face at the mesh boundaries at least, if any. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] ghost true if the ghosts must be accounted into the search, false otherwise
- Returns
- list of cell ids.
Definition at line 2837 of file MimmoObject.cpp.
◆ extractBoundaryCellID() [2/2]
livector1D mimmo::MimmoObject::extractBoundaryCellID | ( | std::unordered_map< long, std::set< int > > & | map | ) |
Extract boundary cells who have one face at the mesh boundaries at least, if any. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] map of border faces previously calculated with extractBoundaryFaceCellID
- Returns
- list of cell ids.
Definition at line 2821 of file MimmoObject.cpp.
◆ extractBoundaryFaceCellID()
std::unordered_map< long, std::set< int > > mimmo::MimmoObject::extractBoundaryFaceCellID | ( | bool | ghost = false | ) |
Extract cells who have one face at the mesh boundaries at least, if any. Return the list of the local faces per cell, which lie exactly on the boundary. The method is meant for connected mesh only, return empty list otherwise. In MPI versions, if mesh is distributed and ghost search is activated, returns all cells (internal and ghost) that have a face properly lying on a physical boundary: faces lying on a rank border are excluded through an internal communication.
- Parameters
-
[in] ghost true if the ghosts must be accounted into the search, false otherwise
- Returns
- map of boundary cell unique-ids, with local boundary faces list.
Definition at line 2719 of file MimmoObject.cpp.
◆ extractBoundaryInterfaceID() [1/2]
livector1D mimmo::MimmoObject::extractBoundaryInterfaceID | ( | bool | ghost = false | ) |
Extract interfaces who lies on the mesh boundaries. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] ghost true if the ghost interfaces must be accounted into the search, false otherwise
- Returns
- list of interface ids.
Definition at line 2918 of file MimmoObject.cpp.
◆ extractBoundaryInterfaceID() [2/2]
livector1D mimmo::MimmoObject::extractBoundaryInterfaceID | ( | std::unordered_map< long, std::set< int > > & | map | ) |
Extract interfaces who lies on the mesh boundaries. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] map of border faces previously calculated with extractBoundaryFaceCellID
- Returns
- list of interface ids.
Definition at line 2890 of file MimmoObject.cpp.
◆ extractBoundaryMesh()
MimmoSharedPointer< MimmoObject > mimmo::MimmoObject::extractBoundaryMesh | ( | ) |
Extract boundary mesh if the current MimmoObject mesh. Boundaries will be stored in an indipendent MimmoObject container, whose topology is:
- Surface Mesh (type 1 ) if the starting mesh is a Volume(2);
- 3DCurve Mesh (type 4 ) if the starting mesh is a Surface(1);
- Point Cloud (type 3) if the starting mesh is a 3DCurve(4); Bulk interfaces are used to create boundary tessellations. Bulk PIDs will be inherited into the boundary mesh. In MPI versions, boundaries will inherit the distributed status of the original mesh
Definition at line 2934 of file MimmoObject.cpp.
◆ extractBoundaryVertexID() [1/2]
livector1D mimmo::MimmoObject::extractBoundaryVertexID | ( | bool | ghost = false | ) |
Extract vertices at the mesh boundaries, if any. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] ghost true if the ghosts must be accounted into the search, false otherwise
- Returns
- list of vertex ids.
Definition at line 2878 of file MimmoObject.cpp.
◆ extractBoundaryVertexID() [2/2]
livector1D mimmo::MimmoObject::extractBoundaryVertexID | ( | std::unordered_map< long, std::set< int > > & | map | ) |
Extract vertices at the mesh boundaries. The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] map of border faces previously calculated with extractBoundaryFaceCellID
- Returns
- list of vertex ids.
Definition at line 2849 of file MimmoObject.cpp.
◆ extractPIDCells() [1/2]
livector1D mimmo::MimmoObject::extractPIDCells | ( | livector1D | flag, |
bool | squeeze = true |
||
) |
Extract all cells marked with a series of target PIDs.
- Parameters
-
[in] flag list of PID for extraction [in] squeeze if true the result container is squeezed once full
- Returns
- list of cells as unique-ids
Definition at line 3146 of file MimmoObject.cpp.
◆ extractPIDCells() [2/2]
livector1D mimmo::MimmoObject::extractPIDCells | ( | long | flag, |
bool | squeeze = true |
||
) |
Extract all cells marked with a target PID flag.
- Parameters
-
[in] flag PID for extraction [in] squeeze if true the result container is squeezed once full*
- Returns
- list of cells as unique-ids
Definition at line 3122 of file MimmoObject.cpp.
◆ extractPIDSubdivision()
std::map< long, livector1D > mimmo::MimmoObject::extractPIDSubdivision | ( | ) |
Extract all cells ids rearranged according to PID subdivision.
- Returns
- map with PID key and list of cells belonging to PID as argument
Definition at line 3163 of file MimmoObject.cpp.
◆ findVertexVertexOneRing()
std::set< long > mimmo::MimmoObject::findVertexVertexOneRing | ( | const long & | cellId, |
const long & | vertexId | ||
) |
Return Vertex-Vertex One Ring of a specified target vertex. Does not work with unconnected meshes (point clouds). In case of distributed patches, the input cell must be an interior cell; in case of ghost cell passed, it returns an empty set.
- Parameters
-
[in] cellId bitpit::PatchKernel Id of a cell which target belongs to [in] vertexId bitpit::PatchKernel Id of the target vertex
- Returns
- list of all vertex in the One Ring of the target, by their bitpit::PatchKernel Ids
Definition at line 4556 of file MimmoObject.cpp.
◆ getAdjacenciesSyncStatus()
SyncStatus mimmo::MimmoObject::getAdjacenciesSyncStatus | ( | ) |
Return cell-cell adjacency synchronization status for your current mesh.
- Returns
- synchronization status of adjacencies.
Definition at line 3446 of file MimmoObject.cpp.
◆ getBorderCells() [1/2]
livector1D mimmo::MimmoObject::getBorderCells | ( | ) |
Get all border cells who have one face at the mesh boundaries at least(physical or rank border). In MPI versions, differently from extractBoundaryCellID, the method return all cells having border faces, those belonging to rank borders, plus those on physical borders, in non-collective way (operations on local rank, no communications). In serial version only is equivalent to method extractBoundaryCellID The method is meant for connected mesh only, return empty list otherwise.
- Returns
- list of cell ids.
Definition at line 3062 of file MimmoObject.cpp.
◆ getBorderCells() [2/2]
livector1D mimmo::MimmoObject::getBorderCells | ( | std::unordered_map< long, std::set< int > > & | map | ) |
Get all border cells who have one face at the mesh boundaries at least(physical or rank border). In MPI versions, differently from extractBoundaryCellID, the method return all cells having border faces, those belonging to rank borders, plus those on physical borders, in non-collective way (operations on local rank, no communications). In serial version only is equivalent to method extractBoundaryCellID The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] map of border faces previously calculated with getBorderFaceCells()
- Returns
- list of cell ids.
Definition at line 3043 of file MimmoObject.cpp.
◆ getBorderFaceCells()
std::unordered_map< long, std::set< int > > mimmo::MimmoObject::getBorderFaceCells | ( | ) |
Return all cells faces at the mesh border. The method is meant for connected mesh only, return empty list otherwise. In MPI versions, differently from extractBoundaryFaceCellID, the method return all faces, those belonging to rank borders, plus those on physical borders, in non-collective way (operations on local rank, no communications) In serial version the method is equivalent to extractBoundaryFaceCellID.
- Returns
- map of boundary cell unique-ids, with local boundary faces list.
Definition at line 3011 of file MimmoObject.cpp.
◆ getBorderVertices() [1/2]
livector1D mimmo::MimmoObject::getBorderVertices | ( | ) |
Get all border vertices belonging to border face at the mesh boundaries (physical or rank border). In MPI versions, differently from extractBoundaryVertexID, the method return all vertices belonging to border faces, those belonging to rank borders, plus those on physical borders, in non-collective way (operations on local rank, no communications). In serial version only is equivalent to method extractBoundaryVertexID The method is meant for connected mesh only, return empty list otherwise.
- Returns
- list of vertex ids.
Definition at line 3110 of file MimmoObject.cpp.
◆ getBorderVertices() [2/2]
livector1D mimmo::MimmoObject::getBorderVertices | ( | std::unordered_map< long, std::set< int > > & | map | ) |
Get all border vertices belonging to border face at the mesh boundaries (physical or rank border). In MPI versions, differently from extractBoundaryVertexID, the method return all vertices belonging to border faces, those belonging to rank borders, plus those on physical borders, in non-collective way (operations on local rank, no communications). In serial version only is equivalent to method extractBoundaryVertexID The method is meant for connected mesh only, return empty list otherwise.
- Parameters
-
[in] map of border faces previously calculated with getBorderFaceCells()
- Returns
- list of vertex ids.
Definition at line 3078 of file MimmoObject.cpp.
◆ getBoundingBox()
void mimmo::MimmoObject::getBoundingBox | ( | std::array< double, 3 > & | pmin, |
std::array< double, 3 > & | pmax, | ||
bool | global = true |
||
) |
Evaluate axis aligned bounding box of the current MimmoObject.
- Parameters
-
[out] pmin lowest bounding box point [out] pmax highest bounding box point [in] global true to ask for global bounding box over the processes
Definition at line 3256 of file MimmoObject.cpp.
◆ getBoundingBoxSyncStatus()
SyncStatus mimmo::MimmoObject::getBoundingBoxSyncStatus | ( | ) |
Return the synchronization status of the patch bounding box
- Returns
- synchronization status of the patch bounding box with your current geometry.
Definition at line 1601 of file MimmoObject.cpp.
◆ getCellConnectivity()
livector1D mimmo::MimmoObject::getCellConnectivity | ( | long | i | ) | const |
It gets the connectivity of a cell, with vertex id's in bitpit::PatchKernel unique indexing. Connectivity of polygons is returned as (nV,V1,V2,V3,V4,...) where nV is the number of vertices defining the polygon and V1,V2,V3... are indices of vertices. Connectivity of polyhedrons is returned as (nF,nF1V, V1, V2, V3,..., nF2V, V2,V4,V5,V6,...,....) where nF is the total number of faces of polyhedros, nF1V is the number of vertices composing the face 1, followed by the indices of vertices which draws it. Face 2,3,..,n are defined in the same way. Any other standard cell element is uniquely defined by its list of vertex indices. if i cell does not exists, return an empty list.
- Parameters
-
[in] i bitpit::PatchKernel ID of the cell.
- Returns
- i-th cell-vertices connectivity, in bitpit::PatchKernel vertex indexing.
Definition at line 1281 of file MimmoObject.cpp.
◆ getCellFromVertexList()
livector1D mimmo::MimmoObject::getCellFromVertexList | ( | const livector1D & | vertexList, |
bool | strict = true |
||
) |
Extract cell list from an ensamble of geometry vertices. Ids of all those cells whose vertex are defined inside the selection will be returned.
- Parameters
-
[in] vertexList list of bitpit::PatchKernel IDs identifying vertices. [in] strict boolean true to restrict search for cells having all vertices in the list, false to include all cells having at least 1 vertex in the list.
- Returns
- list of bitpit::PatchKernel IDs of involved 1-Ring cells.
Definition at line 2633 of file MimmoObject.cpp.
◆ getCells()
const bitpit::PiercedVector< bitpit::Cell > & mimmo::MimmoObject::getCells | ( | ) |
Return reference to the PiercedVector structure of local cells hold by bitpit::PatchKernel class member. Ghost cells are considered.
- Returns
- Pierced Vector structure of cells
Return const reference to the PiercedVector structure of local cells hold by bitpit::PatchKernel class member. Ghost cells are considered.
- Returns
- const Pierced Vector structure of cells
Definition at line 1301 of file MimmoObject.cpp.
◆ getCellsIds()
livector1D mimmo::MimmoObject::getCellsIds | ( | bool | internalsOnly = false | ) |
Return a compact vector with the Ids of local cells given by bitpit::PatchKernel unique-labeled indexing.
- Parameters
-
[in] internalsOnly FOR MPI ONLY: if true method accounts for internal cells only, if false it includes also ghosts. Serial comp ignore this parameter.
- Returns
- ids of cells
Definition at line 1345 of file MimmoObject.cpp.
◆ getCellsNarrowBandToExtSurface()
livector1D mimmo::MimmoObject::getCellsNarrowBandToExtSurface | ( | MimmoObject & | surface, |
const double & | maxdist, | ||
livector1D * | seedlist = nullptr |
||
) |
Get all the cells of the current mesh whose center is within a prescribed distance maxdist w.r.t to a target surface body. Ghost cells are considered. See twin method getCellsNarrowBandToExtSurfaceWDist.
- Parameters
-
[in] surface MimmoObject of type surface. [in] maxdist threshold distance. [in] seedlist (optional) list of cells to starting narrow band search
- Returns
- list of cells inside the narrow band at maxdist from target surface.
Definition at line 4116 of file MimmoObject.cpp.
◆ getCellsNarrowBandToExtSurfaceWDist()
bitpit::PiercedVector< double > mimmo::MimmoObject::getCellsNarrowBandToExtSurfaceWDist | ( | MimmoObject & | surface, |
const double & | maxdist, | ||
livector1D * | seedlist = nullptr |
||
) |
Get all the cells of the current mesh whose center is within a prescribed distance maxdist w.r.t to a target surface body. Ghost cells are considered.
- Parameters
-
[in] surface MimmoObject of type surface. [in] maxdist threshold distance. [in] seedlist (optional) list of cells to starting narrow band search.
- Returns
- list of cells inside the narrow band at maxdist from target surface with their distance from it.
Definition at line 4131 of file MimmoObject.cpp.
◆ getCompactConnectivity()
livector2D mimmo::MimmoObject::getCompactConnectivity | ( | lilimap & | mapDataInv | ) |
Get the local geometry compact connectivity. "Compact" means that in the connectivity matrix the vertex indexing is referred to the local, compact and sequential numbering of vertices, as it gets them from the internal method getVertexCoords(). Special connectivity is returned for polygons and polyhedra support. See getCellConnectivity doxy for further info. Ghost cells are visited to fill the structure.
- Returns
- local connectivity list
- Parameters
-
[in] mapDataInv inverse of Map of vertex ids actually set, for aligning external vertex data to bitpit::Patch ordering
Definition at line 1204 of file MimmoObject.cpp.
◆ getCompactPID()
livector1D mimmo::MimmoObject::getCompactPID | ( | ) |
- Returns
- the list of PID associated to each cell of tessellation in compact sequential ordering. Ghost cells are considered. If empty list is returned, pidding is not supported for this geometry.
Definition at line 1552 of file MimmoObject.cpp.
◆ getConnectivity()
livector2D mimmo::MimmoObject::getConnectivity | ( | ) |
It gets the connectivity of the local cells of the linked geometry. Index of vertices in connectivity matrix are returned according to bitpit::PatchKernel unique indexing (ids). Special connectivity is returned for polygons and polyhedra support. See getCellConnectivity doxy for further info. Ghost cells are visited to fill the structure.
- Returns
- cell-vertices connectivity
Definition at line 1250 of file MimmoObject.cpp.
◆ getInfoSyncStatus()
SyncStatus mimmo::MimmoObject::getInfoSyncStatus | ( | ) |
Return the synchronization status of the patch numbering info structure for cells
- Returns
- synchronization status of the patch numbering info with your current geometry.
Definition at line 1592 of file MimmoObject.cpp.
◆ getInterfaceFromCellList()
livector1D mimmo::MimmoObject::getInterfaceFromCellList | ( | const livector1D & | cellList, |
bool | all = true |
||
) |
Extract interfaces list from an ensamble of geometry cells. Build Interfaces if the target mesh has none.
- Parameters
-
[in] cellList list of bitpit::PatchKernel ids identifying cells.
- Returns
- the list of bitpit::PatchKernel ids of involved interfaces.
- Parameters
-
[in] all extract all interfaces af listed cells. Otherwise (if false) extract only the interfaces shared by the listed cells.
Definition at line 2585 of file MimmoObject.cpp.
◆ getInterfaceFromVertexList()
livector1D mimmo::MimmoObject::getInterfaceFromVertexList | ( | const livector1D & | vertexList, |
bool | strict, | ||
bool | border | ||
) |
Extract interfaces list from an ensamble of geometry vertices. Ids of all those interfaces whose vertices are defined inside the selection will be returned. Interfaces are automatically built, if the mesh has none. "strict" boolean, if true, controls that interfaces have all connectivity points inside the vertex list, if false insert all interfaces having at least one point inside such list. "border" boolean, if true, limits the search to interface lying on the mesh border. Please, be aware, in case of MPI distributed mesh, all borders of partition are considered, physical and rank borders.
- Parameters
-
[in] vertexList list of bitpit::PatchKernel IDs identifying vertices. [in] strict boolean true to restrict search for cells having all vertices in the list, false to include all cells having at least 1 vertex in the list. [in] border boolean true to restrict the search to border interfaces, false to include all interfaces
- Returns
- list of bitpit::PatchKernel IDs of involved interfaces
Definition at line 2676 of file MimmoObject.cpp.
◆ getInterfaces()
const bitpit::PiercedVector< bitpit::Interface > & mimmo::MimmoObject::getInterfaces | ( | ) |
Return reference to the PiercedVector structure of local interfaces hold by bitpit::PatchKernel class member. Interface between internal and ghost cells are considered.
- Returns
- Pierced Vector structure of interfaces
Return const reference to the PiercedVector structure of local interfaces hold by bitpit::PatchKernel class member. Interfaces between internal and ghost cells are considered.
- Returns
- const Pierced Vector structure of interfaces
Definition at line 1322 of file MimmoObject.cpp.
◆ getInterfacesSyncStatus()
SyncStatus mimmo::MimmoObject::getInterfacesSyncStatus | ( | ) |
Return cell-cell interfaces synchronization status for your current mesh.
- Returns
- synchronization status of interfaces.
Definition at line 3460 of file MimmoObject.cpp.
◆ getInverseConnectivity()
std::unordered_map< long, long > mimmo::MimmoObject::getInverseConnectivity | ( | ) |
Get a minimal inverse connectivity of a target geometry mesh. Each vertex (passed by Id) is associated at list to one of the possible simplex (passed by Id) which it belongs. This is returned in an unordered_map having as key the vertex Id and as value the Cell id. Id is meant as the unique label identifier associated to bitpit::PatchKernel original geometry Ghost cells are considered.
- Returns
- unordered_map of vertex ids (key) vs cell-belonging-to ids(value)
Definition at line 4532 of file MimmoObject.cpp.
◆ getKdTree()
bitpit::KdTree< 3, bitpit::Vertex, long > * mimmo::MimmoObject::getKdTree | ( | ) |
- Returns
- pointer to geometry KdTree internal structure
Definition at line 1634 of file MimmoObject.cpp.
◆ getKdTreeSyncStatus()
SyncStatus mimmo::MimmoObject::getKdTreeSyncStatus | ( | ) |
Return the synchronization status of the kdTree ordering structure for cells
- Returns
- synchronization status of the kdTree with your current geometry.
Definition at line 1626 of file MimmoObject.cpp.
◆ getLog()
bitpit::Logger & mimmo::MimmoObject::getLog | ( | ) |
- Returns
- reference to internal logger
Definition at line 978 of file MimmoObject.cpp.
◆ getMapCell()
lilimap mimmo::MimmoObject::getMapCell | ( | bool | withghosts = true | ) |
Return the map of consecutive global indexing of the local cells; to pass from consecutive global index of a local cell to bitpit::PatchKernel unique-labeled indexing. Ghost cells are considered.
- Parameters
-
[in] withghosts If true the structure is filled with ghosts (meaningful only in parallel versions)
- Returns
- global consecutive / local unique id map
Definition at line 1473 of file MimmoObject.cpp.
◆ getMapCellInv()
lilimap mimmo::MimmoObject::getMapCellInv | ( | bool | withghosts = true | ) |
Return the map of consecutive global indexing of the local cells; to pass from bitpit::PatchKernel unique-labeled indexing of a local cell to consecutive global index. Ghost cells are considered.
- Parameters
-
[in] withghosts If true the structure is filled with ghosts (meaningful only in parallel versions)
- Returns
- local unique id / global consecutive map
Definition at line 1494 of file MimmoObject.cpp.
◆ getMapData()
lilimap mimmo::MimmoObject::getMapData | ( | bool | withghosts = false | ) |
Return the local indexing vertex map, to pass from local, compact indexing to bitpit::PatchKernel unique-labeled indexing.
- Parameters
-
[in] withghosts If true the structure is filled with ghosts (meaningful only in parallel versions)
- Returns
- local/unique-id map
Definition at line 1423 of file MimmoObject.cpp.
◆ getMapDataInv()
lilimap mimmo::MimmoObject::getMapDataInv | ( | bool | withghosts = true | ) |
Return the local indexing vertex map, to pass from bitpit::PatchKernel unique-labeled indexing to local, compact indexing. Note, ghost vertices are not considered in the consecutive map.
- Parameters
-
[in] withghosts If true the structure is filled with ghosts (meaningful only in parallel versions)
- Returns
- unique-id/local map
Definition at line 1441 of file MimmoObject.cpp.
◆ getNCells()
long mimmo::MimmoObject::getNCells | ( | ) |
Return the total number of local cells within the data structure. For parallel versions: ghost cells are considered in the count.
- Returns
- number of mesh cells.
Definition at line 1032 of file MimmoObject.cpp.
◆ getNInternals()
long mimmo::MimmoObject::getNInternals | ( | ) | const |
Return the total number of local internal cells (no ghosts) within the data structure
- Returns
- number of mesh cells.
Definition at line 1064 of file MimmoObject.cpp.
◆ getNInternalVertices()
long mimmo::MimmoObject::getNInternalVertices | ( | ) |
Return the number of interior vertices (no ghosts) within the data structure.
- Returns
- number of interior mesh vertices
Definition at line 1074 of file MimmoObject.cpp.
◆ getNVertices()
long mimmo::MimmoObject::getNVertices | ( | ) |
Return the total number of local vertices within the data structure. For parallel versions: ghost vertices are considered in the count.
- Returns
- number of mesh vertices
Definition at line 1021 of file MimmoObject.cpp.
◆ getPatch()
const bitpit::PatchKernel * mimmo::MimmoObject::getPatch | ( | ) |
- Returns
- pointer to bitpit::PatchKernel structure hold by the class.
Definition at line 1402 of file MimmoObject.cpp.
◆ getPatchInfo()
bitpit::PatchNumberingInfo * mimmo::MimmoObject::getPatchInfo | ( | ) |
- Returns
- pointer to geometry patch numbering info structure
Definition at line 1617 of file MimmoObject.cpp.
◆ getPID()
std::unordered_map< long, long > mimmo::MimmoObject::getPID | ( | ) |
- Returns
- the map of the PID (argument) associated to each cell local unique-id (key) in tessellation. If empty map is returned, pidding is not supported for this geometry. Ghost cells are considered.
Definition at line 1569 of file MimmoObject.cpp.
◆ getPIDTypeList()
const std::unordered_set< long > & mimmo::MimmoObject::getPIDTypeList | ( | ) |
- Returns
- the list of PID types actually present in your geometry. If empty list is returned, pidding is actually not supported for this geometry
Definition at line 1515 of file MimmoObject.cpp.
◆ getPIDTypeListWNames()
const std::unordered_map< long, std::string > & mimmo::MimmoObject::getPIDTypeListWNames | ( | ) |
- Returns
- the list of PID types actually present in your geometry with its custom names attached. If empty list is returned, pidding is actually not supported for this geometry
Definition at line 1524 of file MimmoObject.cpp.
◆ getPointConnectivity()
std::unordered_set< long > & mimmo::MimmoObject::getPointConnectivity | ( | const long & | id | ) |
Get the connectivity of a target node/vertex
- Parameters
-
[in] id of target node
- Returns
- connectivity nodes list of id-target.
Definition at line 4757 of file MimmoObject.cpp.
◆ getPointConnectivitySyncStatus()
SyncStatus mimmo::MimmoObject::getPointConnectivitySyncStatus | ( | ) |
- Returns
- the node-node connectivity sync status.
Definition at line 4767 of file MimmoObject.cpp.
◆ getProcessorCount()
int mimmo::MimmoObject::getProcessorCount | ( | ) | const |
Gets the MPI processors in the communicator associated to the object. For serial version return always 1
- Returns
- The MPI processors in the communicator associated to the object
Definition at line 1678 of file MimmoObject.cpp.
◆ getRank()
int mimmo::MimmoObject::getRank | ( | ) | const |
Gets the MPI rank associated to the object. For serial version return always 0.
- Returns
- The MPI rank associated to the object.
Definition at line 1668 of file MimmoObject.cpp.
◆ getSkdTree()
bitpit::PatchSkdTree * mimmo::MimmoObject::getSkdTree | ( | ) |
- Returns
- pointer to geometry skdTree search structure
Definition at line 1609 of file MimmoObject.cpp.
◆ getSkdTreeSyncStatus()
SyncStatus mimmo::MimmoObject::getSkdTreeSyncStatus | ( | ) |
Return the synchronization status of the skdTree ordering structure for cells
- Returns
- synchronization status of the skdTree with your current geometry.
Definition at line 1583 of file MimmoObject.cpp.
◆ getTolerance()
double mimmo::MimmoObject::getTolerance | ( | ) |
Get the geometric tolerance used to perform geometric operations on the geometry. param[out] Geometric tolerance
Definition at line 1659 of file MimmoObject.cpp.
◆ getType()
int mimmo::MimmoObject::getType | ( | ) |
Return the type of mesh currently hold by the class (for type of mesh allowed see MimmoObject(int type) documentation).
- Returns
- integer flag for mesh type
Definition at line 1011 of file MimmoObject.cpp.
◆ getVertexCoords()
const darray3E & mimmo::MimmoObject::getVertexCoords | ( | long | i | ) | const |
Gets the vertex coordinates marked with a unique label i (bitpit::PatchKernel id); If i is not found, return a default value (max double limit).
- Parameters
-
[in] i bitpit::PatchKernel id of the vertex in the mesh.
- Returns
- Coordinates of the i-th vertex of geometry mesh.
Definition at line 1165 of file MimmoObject.cpp.
◆ getVertexFromCellList()
livector1D mimmo::MimmoObject::getVertexFromCellList | ( | const livector1D & | cellList | ) |
Extract vertex list from an ensamble of geometry cells.
- Parameters
-
[in] cellList list of bitpit::PatchKernel ids identifying cells.
- Returns
- the list of bitpit::PatchKernel ids of involved vertices.
Definition at line 2557 of file MimmoObject.cpp.
◆ getVertices()
const bitpit::PiercedVector< bitpit::Vertex > & mimmo::MimmoObject::getVertices | ( | ) |
Return reference to the PiercedVector structure of local vertices hold by bitpit::PatchKernel class member. Ghost cells vertices are considered.
- Returns
- Pierced Vector structure of vertices
Return const reference to the PiercedVector structure of local vertices hold by bitpit::PatchKernel class member. Ghost cells vertices are considered.
- Returns
- const Pierced Vector structure of vertices
Definition at line 1177 of file MimmoObject.cpp.
◆ getVerticesCoords()
Return the compact list of local vertices hold by the class. Ghost cells are considered to fill the structure.
- Returns
- coordinates of mesh vertices
- Parameters
-
[out] mapDataInv pointer to inverse of Map of vertex ids, for aligning external vertex data to bitpit::Patch ordering.
Definition at line 1136 of file MimmoObject.cpp.
◆ getVerticesIds()
livector1D mimmo::MimmoObject::getVerticesIds | ( | bool | internalsOnly = false | ) |
Return a compact vector with the Ids of local vertices given by bitpit::PatchKernel unique-labeled indexing.
- Parameters
-
[in] internalsOnly if true method accounts for internal vertices only, if false it includes also ghosts.
- Returns
- ids of vertices
Definition at line 1376 of file MimmoObject.cpp.
◆ getVerticesNarrowBandToExtSurface()
livector1D mimmo::MimmoObject::getVerticesNarrowBandToExtSurface | ( | MimmoObject & | surface, |
const double & | maxdist, | ||
livector1D * | seedlist = nullptr |
||
) |
Get all the vertices of the current mesh within a prescribed distance maxdist w.r.t to a target surface body. Ghost vertices are considered. See twin method getCellsNarrowBandToExtSurfaceWDist.
- Parameters
-
[in] surface MimmoObject of type surface. [in] maxdist threshold distance. [in] seedlist (optional) list of vertices to starting narrow band search. In case the current class is a point cloud, a non empty seed list is mandatory.
- Returns
- list of vertices inside the narrow band at maxdist from target surface.
Definition at line 4323 of file MimmoObject.cpp.
◆ getVerticesNarrowBandToExtSurfaceWDist()
bitpit::PiercedVector< double > mimmo::MimmoObject::getVerticesNarrowBandToExtSurfaceWDist | ( | MimmoObject & | surface, |
const double & | maxdist, | ||
livector1D * | seedlist = nullptr |
||
) |
Get all the vertices of the current mesh within a prescribed distance maxdist w.r.t to a target surface body. Ghost vertices are considered.
- Parameters
-
[in] surface MimmoObject of type surface. [in] maxdist threshold distance. [in] seedlist (optional) list of vertices to starting narrow band search. In case the current class is a point cloud, a non empty seed list is mandatory.
- Returns
- list of vertices inside the narrow band at maxdist from target surface with their distance from it.
Definition at line 4339 of file MimmoObject.cpp.
◆ initializeLogger()
|
protected |
Initialize the logger.
Definition at line 905 of file MimmoObject.cpp.
◆ isClosedLoop()
bool mimmo::MimmoObject::isClosedLoop | ( | ) |
- Returns
- false if your local mesh has open edges/faces. True otherwise. Please note in distributed archs, the method cannot be called locally, but it needs to be called globally from each procs.
Definition at line 3475 of file MimmoObject.cpp.
◆ isEmpty()
bool mimmo::MimmoObject::isEmpty | ( | ) |
Is the object empty?
- Returns
- True/False if the local geometry data structure is empty.
Definition at line 987 of file MimmoObject.cpp.
◆ isParallel()
bool mimmo::MimmoObject::isParallel | ( | ) |
Give if the patch is a parallel patch, i.e. it has a communicator set and it can be distributed over the processes (see isDistributed() method).
Definition at line 2086 of file MimmoObject.cpp.
◆ isPointInterior()
bool mimmo::MimmoObject::isPointInterior | ( | long | id | ) |
Get if a vertex is local or not. The structures have to be previously updated (not internal sync).
- Parameters
-
[in] id Vertex id Note: if not mpi support enabled or patch not partitioned return true (no check if node exists).
Definition at line 1644 of file MimmoObject.cpp.
◆ isSkdTreeSupported()
bool mimmo::MimmoObject::isSkdTreeSupported | ( | ) |
Is the skdTree ordering supported w/ the current geometry?
- Returns
- true for connectivity-based meshes, false por point clouds
Definition at line 1001 of file MimmoObject.cpp.
◆ modifyVertex()
bool mimmo::MimmoObject::modifyVertex | ( | const darray3E & | vertex, |
const long & | id | ||
) |
It modifies the coordinates of a pre-existent vertex.
- Parameters
-
[in] vertex new vertex coordinates [in] id unique-id of the vertex meant to be modified
- Returns
- false if no geometry is present or vertex id does not exist.
Definition at line 2188 of file MimmoObject.cpp.
◆ reset()
|
protected |
Reset class to default constructor set-up.
- Parameters
-
[in] type type of mesh from 1 to 4; See default constructor. [in] isParallel if true reset to parallel MimmoObject (if MPI not enabled forced to false)
Definition at line 3724 of file MimmoObject.cpp.
◆ resetPatch()
void mimmo::MimmoObject::resetPatch | ( | ) |
Force the class to reset all the patch structures, i.e. vertices, cells, interfaces.
Definition at line 3647 of file MimmoObject.cpp.
◆ restore()
void mimmo::MimmoObject::restore | ( | std::istream & | stream | ) |
Restore contents of a dumped MimmoObject from a stream in your current class. New restored data will be owned internally by the class. Every data previously stored will be lost. Tree are not restored by default. Read all in a binary format.
- Parameters
-
[in,out] stream to write on.
Definition at line 3857 of file MimmoObject.cpp.
◆ resyncPID()
void mimmo::MimmoObject::resyncPID | ( | ) |
Update class member map m_pidsType with PIDs effectively contained. Beware any label name previously stored for pid will be lost. in the internal/linked geometry patch.
Definition at line 2437 of file MimmoObject.cpp.
◆ setPID() [1/2]
void mimmo::MimmoObject::setPID | ( | livector1D | pids | ) |
Set PIDs for all geometry cells available. Any previous PID subdivion with label name associated will be erased. The PID list must be referred to the compact local/indexing of the cells in the class.
- Parameters
-
[in] pids PID list.
Definition at line 2359 of file MimmoObject.cpp.
◆ setPID() [2/2]
void mimmo::MimmoObject::setPID | ( | std::unordered_map< long, long > | pidsMap | ) |
Set PIDs for all geometry cells available. Any previous PID subdivion with label name associated will be erased. The PID must be provided as a map with cell unique-id as key and pid associated to it as argument.
- Parameters
-
[in] pidsMap PID amp.
Definition at line 2383 of file MimmoObject.cpp.
◆ setPIDCell()
void mimmo::MimmoObject::setPIDCell | ( | long | id, |
long | pid | ||
) |
Set the PID name, if PID exists
- Parameters
-
[in] id unique-id of the cell [in] pid PID to assign on cell
Definition at line 2408 of file MimmoObject.cpp.
◆ setPIDName()
bool mimmo::MimmoObject::setPIDName | ( | long | pid, |
const std::string & | name | ||
) |
Set the PID of a target cell
- Parameters
-
[in] pid PID to assign on cell [in] name name to be assigned to the pid
- Returns
- true if name is assigned, false if not.
Definition at line 2425 of file MimmoObject.cpp.
◆ setTolerance()
void mimmo::MimmoObject::setTolerance | ( | double | tol | ) |
Set geometric tolerance used to perform geometric operations on the patch. It set directly even the tolerance of the patch if not null. param[in] tol input geometric tolerance
Definition at line 2101 of file MimmoObject.cpp.
◆ setUnsyncAll()
void mimmo::MimmoObject::setUnsyncAll | ( | ) |
Unsynchronizes all MimmoObject patch structures, namely adjacencies, interfaces, skdtree, kdtree, internal patch numbering info, bounding box, and for MPI version ghost structures. If any of the structures has a SyncStatus flag lesser then UNSYNC, preserve it. This is a useful call when intense mesh modifications (insertion, deletion of vertices and cells) are done externally, and all structures needed to be re-synchronized as consequence. In that case use this method before calling the MimmoObject::update();
Definition at line 2539 of file MimmoObject.cpp.
◆ swap()
|
noexcept |
Swap function. Swap data between current object and one of the same type.
- Parameters
-
[in] x object to be swapped.
Definition at line 863 of file MimmoObject.cpp.
◆ triangulate()
void mimmo::MimmoObject::triangulate | ( | ) |
Triangulate the linked geometry. It works only for surface geometries (type = 1). After the method call the geometry (internal or linked) is forever modified.
Definition at line 4776 of file MimmoObject.cpp.
◆ update()
void mimmo::MimmoObject::update | ( | ) |
Update the MimmoObject after a manipulation, i.e. a mesh adaption, a cells/vertices insertion/delete or a generic manipulation. Note. To update parallel information the cell adjacencies have to be built.
Definition at line 3359 of file MimmoObject.cpp.
◆ updateAdjacencies()
void mimmo::MimmoObject::updateAdjacencies | ( | ) |
Update cell-cell adjacency connectivity.
Definition at line 3571 of file MimmoObject.cpp.
◆ updateInterfaces()
void mimmo::MimmoObject::updateInterfaces | ( | ) |
Update Interfaces connectivity. If needed the adjacencies are updated too. If MimmoObject does not support connectivity (as Point Clouds do) does nothing.
Definition at line 3600 of file MimmoObject.cpp.
Member Data Documentation
◆ m_AdjSync
|
protected |
Synchronization status of adjacencies along with geometry modifications
Definition at line 162 of file MimmoObject.hpp.
◆ m_boundingBoxSync
|
protected |
Synchronization status of patch bounding box along with geometry modifications
Definition at line 183 of file MimmoObject.hpp.
◆ m_infoSync
|
protected |
Synchronization status of patch info along with geometry modifications
Definition at line 181 of file MimmoObject.hpp.
◆ m_IntSync
|
protected |
Synchronization status of interfaces along with geometry modifications
Definition at line 163 of file MimmoObject.hpp.
◆ m_isParallel
|
protected |
True if the geometry is parallel.
Definition at line 166 of file MimmoObject.hpp.
◆ m_kdTree
|
protected |
ordered tree of geometry vertices for fast searching purposes
Definition at line 158 of file MimmoObject.hpp.
◆ m_kdTreeSync
|
protected |
Synchronization status of kdtree.
Definition at line 160 of file MimmoObject.hpp.
◆ m_log
|
protected |
Pointer to logger.
Definition at line 164 of file MimmoObject.hpp.
◆ m_nprocs
|
protected |
Total number of processors.
Definition at line 167 of file MimmoObject.hpp.
◆ m_patchInfo
|
protected |
Patch Numbering Info structure for cells.
Definition at line 180 of file MimmoObject.hpp.
◆ m_pidsType
|
protected |
pid type available for your geometry
Definition at line 155 of file MimmoObject.hpp.
◆ m_pidsTypeWNames
|
protected |
pid type available for your geometry, with name attached
Definition at line 156 of file MimmoObject.hpp.
◆ m_pointConnectivity
|
protected |
Point-Point connectivity. 1-Ring neighbours of each vertex.
Definition at line 185 of file MimmoObject.hpp.
◆ m_pointConnectivitySync
|
protected |
Track correct building of points connectivity along with geometry modifications
Definition at line 186 of file MimmoObject.hpp.
◆ m_rank
|
protected |
Current rank number.
Definition at line 168 of file MimmoObject.hpp.
◆ m_skdTree
|
protected |
ordered tree of geometry simplicies for fast searching purposes
Definition at line 157 of file MimmoObject.hpp.
◆ m_skdTreeSync
|
protected |
Synchronization status of bvtree.
Definition at line 159 of file MimmoObject.hpp.
◆ m_type
|
protected |
Type of geometry (0 = undefined, 1 = surface mesh, 2 = volume mesh, 3-point cloud mesh, 4-3DCurve).
Definition at line 154 of file MimmoObject.hpp.
The documentation for this class was generated from the following files:
- src/core/MimmoObject.hpp
- src/core/MimmoObject.cpp
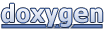