MimmoObject.cpp
255 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(2, getCommunicator())));
259 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
260 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
264 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoVolUnstructured(3, getCommunicator())));
268 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(m_patch.get()))));
269 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
273 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoPointCloud(getCommunicator())));
277 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
281 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(1, getCommunicator())));
285 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
286 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
289 (*m_log)<<"Error MimmoObject: unrecognized data structure type in class construction."<<std::endl;
351 MimmoObject::MimmoObject(int type, dvecarr3E & vertex, livector2D * connectivity, bool isParallel):m_patch(nullptr),m_extpatch(nullptr){
369 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(2, getCommunicator())));
373 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
374 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
378 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoVolUnstructured(3, getCommunicator())));
382 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(m_patch.get()))));
383 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
387 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoPointCloud(getCommunicator())));
391 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
395 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(1, getCommunicator())));
399 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
400 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
403 (*m_log)<<"Error MimmoObject: unrecognized data structure type in class construction."<<std::endl;
438 addConnectedCell(cc, eltype); //if MPI, is adding cell with rank =-1, that is is using local m_rank.
440 (*m_log)<<"warning: in MimmoObject custom constructor. Undefined cell type detected and skipped."<<std::endl;
469 MimmoObject::MimmoObject(int type, bitpit::PatchKernel* geometry):m_patch(nullptr),m_extpatch(nullptr){
473 throw std::runtime_error ("MimmoObject : unrecognized mesh type or nullptr argument in class construction");
506 (*m_log)<<"Error MimmoObject: unsupported Elements for required type in linked mesh."<<std::endl;
507 throw std::runtime_error ("MimmoObject :unsupported Elements for required type in linked mesh.");
509 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(geometry))));
510 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
521 (*m_log)<<"Error MimmoObject: unsupported Elements for required type in linked mesh."<<std::endl;
522 throw std::runtime_error ("MimmoObject :unsupported Elements for required type in linked mesh.");
524 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(geometry))));
525 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
541 (*m_log)<<"Error MimmoObject: unsupported elements for required type in linked mesh."<<std::endl;
542 throw std::runtime_error ("MimmoObject :unsupported elements for required type in linked mesh.");
544 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
560 (*m_log)<<"Error MimmoObject: unsupported elements for required type in linked mesh."<<std::endl;
561 throw std::runtime_error ("MimmoObject :unsupported elements for required type in linked mesh.");
563 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(geometry))));
564 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
580 // Check if adjacencies and interfaces are built.(Patch called it BuildStrategy -- NONE is unbuilt)
581 if (geometry->getAdjacenciesBuildStrategy() == bitpit::PatchKernel::AdjacenciesBuildStrategy::ADJACENCIES_NONE){
589 if (geometry->getInterfacesBuildStrategy() == bitpit::PatchKernel::InterfacesBuildStrategy::INTERFACES_NONE){
625 MimmoObject::MimmoObject(int type, std::unique_ptr<bitpit::PatchKernel> & geometry):m_patch(nullptr),m_extpatch(nullptr){
660 (*m_log)<<"Error MimmoObject: unsupported Elements for required type in linked mesh."<<std::endl;
661 throw std::runtime_error ("MimmoObject :unsupported Elements for required type in linked mesh.");
663 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(getPatch()))));
664 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
675 (*m_log)<<"Error MimmoObject: unsupported Elements for required type in linked mesh."<<std::endl;
676 throw std::runtime_error ("MimmoObject :unsupported Elements for required type in linked mesh.");
678 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(getPatch()))));
679 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
695 (*m_log)<<"Error MimmoObject: unsupported elements for required type in linked mesh."<<std::endl;
696 throw std::runtime_error ("MimmoObject :unsupported elements for required type in linked mesh.");
697 } m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
713 (*m_log)<<"Error MimmoObject: unsupported elements for required type in linked mesh."<<std::endl;
714 throw std::runtime_error ("MimmoObject :unsupported elements for required type in linked mesh.");
716 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(getPatch()))));
717 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
735 // Check if adjacencies and interfaces are built.(Patch called it BuildStrategy -- NONE is unbuilt)
736 if (getPatch()->getAdjacenciesBuildStrategy() == bitpit::PatchKernel::AdjacenciesBuildStrategy::ADJACENCIES_NONE){
744 if (getPatch()->getInterfacesBuildStrategy() == bitpit::PatchKernel::InterfacesBuildStrategy::INTERFACES_NONE){
810 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_extpatch))));
811 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
814 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(m_extpatch))));
815 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
818 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
821 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_extpatch))));
822 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
912 bitpit::log::manager().initialize(bitpit::log::Mode::COMBINED, MIMMO_LOG_FILE, true, MIMMO_LOG_DIR, m_nprocs, m_rank);
914 bitpit::log::manager().initialize(bitpit::log::Mode::COMBINED, MIMMO_LOG_FILE, true, MIMMO_LOG_DIR);
1353 for(bitpit::PatchKernel::CellIterator it = getPatch()->internalCellBegin(); it!= getPatch()->internalCellEnd(); ++it){
1702 const std::unordered_map<int, std::vector<long>> & MimmoObject::getPointGhostExchangeTargets() const
1713 const std::unordered_map<int, std::vector<long>> & MimmoObject::getPointGhostExchangeSources() const
1727 MPI_Allreduce(MPI_IN_PLACE, &m_pointGhostExchangeInfoSync, 1, MPI_LONG, MPI_MIN, m_communicator);
1755 MPI_Allgather(&rankinteriorvertices, 1, MPI_LONG, m_rankinteriorvertices.data(), 1, MPI_LONG, m_communicator);
1783 dataCommunicator = std::unique_ptr<bitpit::DataCommunicator>(new bitpit::DataCommunicator(getCommunicator()));
1994 MPI_Allreduce(MPI_IN_PLACE, &m_pointGhostExchangeInfoSync, 1, MPI_LONG, MPI_MIN, m_communicator);
2122 if(idtag != bitpit::Vertex::NULL_ID && getVertices().exists(idtag)) return bitpit::Vertex::NULL_ID;
2158 if(idtag != bitpit::Vertex::NULL_ID && getVertices().exists(idtag)) return bitpit::Vertex::NULL_ID;
2219 MimmoObject::addConnectedCell(const livector1D & conn, bitpit::ElementType type, long idtag, int rank){
2249 MimmoObject::addConnectedCell(const livector1D & conn, bitpit::ElementType type, long PID, long idtag, int rank){
2319 //patchkernel methods addCell(const & Cell... ) copy everything from the target cell, adjacencies and interfaces included.
2321 // But pay attention these information are useless for you, since you considered interfaces and
2488 //rank, nprocs and communicator managed inside initializeParallel call of the MimmoObject constructor
2614 if (targetCells.count(idowner) && (targetCells.count(idneigh) || idneigh == bitpit::Element::NULL_ID)){
2676 livector1D MimmoObject::getInterfaceFromVertexList(const livector1D & vertexList, bool strict, bool border){
2727 if(getAdjacenciesSyncStatus() != SyncStatus::SYNC) updateAdjacencies(); //forcing adjacencies building
2732 for (bitpit::PatchKernel::CellIterator it = getPatch()->internalCellBegin(); it != getPatch()->internalCellEnd(); ++it){
2749 std::unique_ptr<bitpit::DataCommunicator> dataCommunicator(new bitpit::DataCommunicator(getCommunicator()));
2972 boundary->addConnectedCell(livector1D(conn, conn+bInt.getConnectSize()), bInt.getType(), PID, idI, rank);
3019 for (bitpit::PatchKernel::CellIterator it = getPatch()->cellBegin(); it != getPatch()->cellEnd(); ++it){
3170 int reserveSize = int(totCells*1.3)/int(m_pidsType.size()); //euristic to not overestimate the reserve on vectors.
3175 for(bitpit::PatchKernel::CellIterator it = getPatch()->cellBegin(); it!= getPatch()->cellEnd(); ++it){
3192 bool MimmoObject::checkCellConnCoherence(const bitpit::ElementType & type, const livector1D & list){
3256 void MimmoObject::getBoundingBox(std::array<double,3> & pmin, std::array<double,3> & pmax, bool global){
3449 if (getPatch()->getAdjacenciesBuildStrategy() == bitpit::PatchKernel::AdjacenciesBuildStrategy::ADJACENCIES_NONE){
3463 if (getPatch()->getInterfacesBuildStrategy() == bitpit::PatchKernel::InterfacesBuildStrategy::INTERFACES_NONE){
3675 //TODO To review in order to implement new derived classes MimmoSurfUnstructured and MimmoVolUnstructured
3684 if(sizeConn > 4 && sizeConn == std::size_t(locConn[0]+1)) result= bitpit::ElementType::POLYGON;
3752 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(2, getCommunicator())));
3756 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
3757 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
3761 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoVolUnstructured(3, getCommunicator())));
3765 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::VolumeSkdTree(dynamic_cast<bitpit::VolumeKernel*>(m_patch.get()))));
3766 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
3770 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoPointCloud(getCommunicator())));
3774 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
3778 m_patch = std::move(std::unique_ptr<bitpit::PatchKernel>(new MimmoSurfUnstructured(1, getCommunicator())));
3782 m_skdTree = std::move(std::unique_ptr<bitpit::PatchSkdTree>(new bitpit::SurfaceSkdTree(dynamic_cast<bitpit::SurfaceKernel*>(m_patch.get()))));
3783 m_kdTree = std::move(std::unique_ptr<bitpit::KdTree<3,bitpit::Vertex,long> >(new bitpit::KdTree<3,bitpit::Vertex, long>()));
3865 throw std::runtime_error("Error during MimmoObject::restore : uncoherent procs number between contents and container");
4116 MimmoObject::getCellsNarrowBandToExtSurface(MimmoObject & surface, const double & maxdist, livector1D * seedlist){
4117 bitpit::PiercedVector<double> res = getCellsNarrowBandToExtSurfaceWDist(surface, maxdist, seedlist);
4131 MimmoObject::getCellsNarrowBandToExtSurfaceWDist(MimmoObject & surface, const double & maxdist, livector1D * seedlist){
4188 candidates = skdTreeUtils::selectByGlobalPatch(surface.getSkdTree(), getSkdTree(), maxdistance);
4211 skdTreeUtils::globalDistance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), surface_ranks.data(), distances.data(), maxdistance);
4215 skdTreeUtils::distance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), distances.data(), maxdistance);
4273 skdTreeUtils::globalDistance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), surface_ranks.data(), distances.data(), maxdistance);
4277 skdTreeUtils::distance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), distances.data(), maxdistance);
4323 MimmoObject::getVerticesNarrowBandToExtSurface(MimmoObject & surface, const double & maxdist, livector1D * seedlist){
4324 bitpit::PiercedVector<double> res = getVerticesNarrowBandToExtSurfaceWDist(surface, maxdist, seedlist);
4339 MimmoObject::getVerticesNarrowBandToExtSurfaceWDist(MimmoObject & surface, const double & maxdist, livector1D * seedlist){
4399 cell_candidates = skdTreeUtils::selectByGlobalPatch(surface.getSkdTree(), getSkdTree(), maxdistance);
4403 cell_candidates = skdTreeUtils::selectByPatch(surface.getSkdTree(), getSkdTree(), maxdistance);
4420 skdTreeUtils::globalDistance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), surface_ranks.data(), distances.data(), maxdistance);
4424 skdTreeUtils::distance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), distances.data(), maxdistance);
4441 std::unordered_set<long> neighs = getPointConnectivity(it.getId()); //only edge connected neighbours.
4482 skdTreeUtils::globalDistance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), surface_ranks.data(), distances.data(), maxdistance);
4486 skdTreeUtils::distance(npoints, points.data(), surface.getSkdTree(), surface_ids.data(), distances.data(), maxdistance);
4556 std::set<long> MimmoObject::findVertexVertexOneRing(const long & cellId, const long & vertexId){
4623 std::array<double,3> enormal = static_cast<bitpit::SurfaceKernel*>(getPatch())->evalEdgeNormal(interf.getOwner(), interf.getOwnerFace());
4661 result = static_cast<bitpit::SurfaceKernel*>(getPatch())->evalEdgeLength(interf.getOwner(), interf.getOwnerFace());
4759 assert(m_pointConnectivity.count(id) > 0 && "MimmoObject::not valid id in getPointConnectivity call");
4779 (*m_log)<<"Error MimmoObject: data structure type different from Surface in triangulate method"<<std::endl;
4780 throw std::runtime_error ("MimmoObject: data structure type different from Surface in triangulate method");
4788 // The ghosts structures are updated at the end of the methods, by calling setPartitioned method.
4792 // TODO INTRODUCE ADAPTING INFORMATION ON VERTICES AND CELLS WHEN USER INTERFACE IS READY IN BITPIT
4899 MimmoObject::degradeDegenerateElements(bitpit::PiercedVector<bitpit::Cell>* degradedDeletedCells,
4940 std::vector<bitpit::Vertex>::iterator it = std::find(cellVertices.begin(), cellVertices.end(), candidateVertex);
livector1D extractBoundaryVertexID(std::unordered_map< long, std::set< int > > &map)
Definition: MimmoObject.cpp:2849
std::unordered_map< long, std::set< int > > getBorderFaceCells()
Definition: MimmoObject.cpp:3011
std::vector< std::vector< long > > decomposeLoop()
Definition: MimmoObject.cpp:3508
@ SYNC
std::set< long > findVertexVertexOneRing(const long &, const long &)
Definition: MimmoObject.cpp:4556
double evalInterfaceArea(const long &id)
Definition: MimmoObject.cpp:4653
MimmoVolUnstructured()
Definition: MimmoObject.cpp:146
SyncStatus
Define status of mimmo object structures like adjacencies, interfaces, etc.
Definition: MimmoObject.hpp:123
bitpit::PiercedVector< bitpit::Vertex > & getVertices()
Definition: MimmoObject.cpp:1177
bitpit::PiercedVector< double > getCellsNarrowBandToExtSurfaceWDist(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4131
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
Custom derivation of bitpit::VolUnstructured class.
Definition: MimmoObject.hpp:73
SyncStatus m_pointConnectivitySync
Definition: MimmoObject.hpp:186
bitpit::PiercedVector< bitpit::Interface > & getInterfaces()
Definition: MimmoObject.cpp:1322
SyncStatus getKdTreeSyncStatus()
Definition: MimmoObject.cpp:1626
dvecarr3E getVerticesCoords(lilimap *mapDataInv=nullptr)
Definition: MimmoObject.cpp:1136
bool setPIDName(long, const std::string &)
Definition: MimmoObject.cpp:2425
livector1D getVertexFromCellList(const livector1D &cellList)
Definition: MimmoObject.cpp:2557
MimmoObject(int type=1, bool isParallel=0)
Definition: MimmoObject.cpp:235
lilimap getMapCell(bool withghosts=true)
Definition: MimmoObject.cpp:1473
long addConnectedCell(const livector1D &locConn, bitpit::ElementType type, int rank=-1)
Definition: MimmoObject.cpp:2209
livector1D getCellsIds(bool internalsOnly=false)
Definition: MimmoObject.cpp:1345
livector1D getCellFromVertexList(const livector1D &vertList, bool strict=true)
Definition: MimmoObject.cpp:2633
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:219
long addVertex(const darray3E &vertex, const long idtag=bitpit::Vertex::NULL_ID)
Definition: MimmoObject.cpp:2120
bitpit::PatchNumberingInfo * getPatchInfo()
Definition: MimmoObject.cpp:1617
livector1D extractBoundaryInterfaceID(std::unordered_map< long, std::set< int > > &map)
Definition: MimmoObject.cpp:2890
bitpit::PiercedVector< bitpit::Cell > & getCells()
Definition: MimmoObject.cpp:1301
bool modifyVertex(const darray3E &vertex, const long &id)
Definition: MimmoObject.cpp:2188
lilimap getMapCellInv(bool withghosts=true)
Definition: MimmoObject.cpp:1494
std::unique_ptr< bitpit::PatchSkdTree > m_skdTree
Definition: MimmoObject.hpp:157
livector2D getCompactConnectivity(lilimap &mapDataInv)
Definition: MimmoObject.cpp:1204
void evalCellAspectRatio(bitpit::PiercedVector< double > &)
Definition: MimmoObject.cpp:3966
std::unique_ptr< bitpit::KdTree< 3, bitpit::Vertex, long > > m_kdTree
Definition: MimmoObject.hpp:158
livector1D getCellsNarrowBandToExtSurface(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4116
std::unordered_map< long, long > getInverseConnectivity()
Definition: MimmoObject.cpp:4532
void cleanPointConnectivity()
Definition: MimmoObject.cpp:4742
bitpit::PatchNumberingInfo m_patchInfo
Definition: MimmoObject.hpp:180
SyncStatus getAdjacenciesSyncStatus()
Definition: MimmoObject.cpp:3446
livector1D extractPIDCells(long, bool squeeze=true)
Definition: MimmoObject.cpp:3122
bitpit::KdTree< 3, bitpit::Vertex, long > * getKdTree()
Definition: MimmoObject.cpp:1634
livector1D getInterfaceFromVertexList(const livector1D &vertList, bool strict, bool border)
Definition: MimmoObject.cpp:2676
bitpit::ElementType desumeElement(const livector1D &)
Definition: MimmoObject.cpp:3676
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:110
std::array< double, 3 > evalInterfaceNormal(const long &id)
Definition: MimmoObject.cpp:4615
std::unordered_map< long, std::string > & getPIDTypeListWNames()
Definition: MimmoObject.cpp:1524
std::unordered_map< long, std::set< int > > extractBoundaryFaceCellID(bool ghost=false)
Definition: MimmoObject.cpp:2719
Custom derivation of bitpit::SurfUnstructured class, for Point Cloud handling only.
Definition: MimmoObject.hpp:99
SyncStatus getInterfacesSyncStatus()
Definition: MimmoObject.cpp:3460
std::unordered_set< int > elementsMap(bitpit::PatchKernel &obj)
Definition: MimmoObject.cpp:4096
void getBoundingBox(std::array< double, 3 > &pmin, std::array< double, 3 > &pmax, bool global=true)
Definition: MimmoObject.cpp:3256
MimmoSurfUnstructured()
Definition: MimmoObject.cpp:73
std::array< double, 3 > evalCellCentroid(const long &id)
Definition: MimmoObject.cpp:4584
std::vector< long > selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol)
Definition: SkdTreeUtils.cpp:251
livector1D getInterfaceFromCellList(const livector1D &cellList, bool all=true)
Definition: MimmoObject.cpp:2585
void degradeDegenerateElements(bitpit::PiercedVector< bitpit::Cell > *degradedDeletedCells=nullptr, bitpit::PiercedVector< bitpit::Vertex > *collapsedVertices=nullptr)
Definition: MimmoObject.cpp:4899
std::array< double, 3 > evalInterfaceCentroid(const long &id)
Definition: MimmoObject.cpp:4597
MimmoSharedPointer< MimmoObject > clone() const
Definition: MimmoObject.cpp:2463
std::unordered_map< long, std::string > m_pidsTypeWNames
Definition: MimmoObject.hpp:156
@ NONE
void buildSkdTree(std::size_t value=1)
Definition: MimmoObject.cpp:3265
SyncStatus getBoundingBoxSyncStatus()
Definition: MimmoObject.cpp:1601
std::unordered_set< long > & getPIDTypeList()
Definition: MimmoObject.cpp:1515
std::map< long, livector1D > extractPIDSubdivision()
Definition: MimmoObject.cpp:3163
livector1D getCellConnectivity(long id) const
Definition: MimmoObject.cpp:1281
SyncStatus getSkdTreeSyncStatus()
Definition: MimmoObject.cpp:1583
std::unordered_set< long > & getPointConnectivity(const long &id)
Definition: MimmoObject.cpp:4757
long addCell(bitpit::Cell &cell, int rank=-1)
Definition: MimmoObject.cpp:2291
livector1D getVerticesIds(bool internalsOnly=false)
Definition: MimmoObject.cpp:1376
void evalCellVolumes(bitpit::PiercedVector< double > &)
Definition: MimmoObject.cpp:3926
@ UNSYNC
SyncStatus getPointConnectivitySyncStatus()
Definition: MimmoObject.cpp:4767
std::unique_ptr< bitpit::PatchKernel > clone() const override
Definition: MimmoObject.cpp:175
livector1D getVerticesNarrowBandToExtSurface(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4323
lilimap getMapData(bool withghosts=false)
Definition: MimmoObject.cpp:1423
livector1D extractBoundaryCellID(bool ghost=false)
Definition: MimmoObject.cpp:2837
Custom derivation of bitpit::SurfUnstructured class.
Definition: MimmoObject.hpp:45
std::unordered_map< long, std::unordered_set< long > > m_pointConnectivity
Definition: MimmoObject.hpp:185
virtual ~MimmoSurfUnstructured()
Definition: MimmoObject.cpp:103
MimmoSharedPointer< MimmoObject > extractBoundaryMesh()
Definition: MimmoObject.cpp:2934
bitpit::PiercedVector< double > getVerticesNarrowBandToExtSurfaceWDist(MimmoObject &surface, const double &maxdist, livector1D *seedList=nullptr)
Definition: MimmoObject.cpp:4339
void buildPointConnectivity()
Definition: MimmoObject.cpp:4681
double distance(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *tree, long &id, double r)
Definition: SkdTreeUtils.cpp:49
double evalCellVolume(const long &id)
Definition: MimmoObject.cpp:4032
lilimap getMapDataInv(bool withghosts=true)
Definition: MimmoObject.cpp:1441
const darray3E & getVertexCoords(long i) const
Definition: MimmoObject.cpp:1165
@ NOTSUPPORTED
virtual ~MimmoVolUnstructured()
Definition: MimmoObject.cpp:168
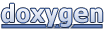