geohandlers_example_00004.cpp
Extract and manipulate topology of a geometry mesh subportion using Pid Selection, Clipping and a Surface Triangulator in a chainUsing: SelectionByPID, ClipGeometry, SurfaceTriangulator, Chain, Partition(MPI version).
To run : ./geohandlers_example_00004
To run (MPI version): mpirun -np X geohandlers_example_00004
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_geohandlers.hpp"
#if MIMMO_ENABLE_MPI
#include "mimmo_parallel.hpp"
#endif
// =================================================================================== //
// =================================================================================== //
/*
* Create a surface polygonal mesh and return it in a MimmoObject.
* Pidding the "M I O" mesh subpart with PID = 1;
*
* \return pointer to the filled polygonal surface mesh
*/
mimmo::MimmoSharedPointer<mimmo::MimmoObject> createMIOMesh(){
int rank = -1;
#if MIMMO_ENABLE_MPI
rank = mesh->getRank();
if ( rank == 0)
#endif
{
//create the vertices set.
mesh->addVertex({{0.0,0.0,0.0}}, 0);
mesh->addVertex({{0.0,1.0,0.0}}, 1);
mesh->addVertex({{0.3,0.0,0.0}}, 2);
mesh->addVertex({{0.3,1.0,0.0}}, 3);
mesh->addVertex({{0.4,0.0,0.0}}, 4);
mesh->addVertex({{0.4,0.75,0.0}}, 5);
mesh->addVertex({{0.4,1.0,0.0}}, 6);
mesh->addVertex({{0.65,0.25,0.0}}, 7);
mesh->addVertex({{0.65,0.5,0.0}}, 8);
mesh->addVertex({{0.9,0.0,0.0}}, 9);
mesh->addVertex({{0.9,0.75,0.0}}, 10);
mesh->addVertex({{0.9,1.0,0.0}}, 11);
mesh->addVertex({{1.0,0.0,0.0}}, 12);
mesh->addVertex({{1.0,1.0,0.0}}, 13);
mesh->addVertex({{1.3,0.0,0.0}}, 14);
mesh->addVertex({{1.3,1.0,0.0}}, 15);
mesh->addVertex({{1.4,0.0,0.0}}, 16);
mesh->addVertex({{1.4,1.0,0.0}}, 17);
mesh->addVertex({{1.7,0.0,0.0}}, 20);
mesh->addVertex({{1.7,1.0,0.0}}, 21);
mesh->addVertex({{1.8,0.0,0.0}}, 22);
mesh->addVertex({{1.8,0.1,0.0}}, 23);
mesh->addVertex({{1.8,0.9,0.0}}, 24);
mesh->addVertex({{1.8,1.0,0.0}}, 25);
mesh->addVertex({{2.3,0.0,0.0}}, 26);
mesh->addVertex({{2.3,0.1,0.0}}, 27);
mesh->addVertex({{2.3,0.9,0.0}}, 28);
mesh->addVertex({{2.3,1.0,0.0}}, 29);
mesh->addVertex({{2.4,0.0,0.0}}, 30);
mesh->addVertex({{2.4,1.0,0.0}}, 31);
mesh->addVertex({{2.7,0.0,0.0}}, 32);
mesh->addVertex({{2.7,1.0,0.0}}, 33);
//add and create polygonal cells
mesh->addConnectedCell(livector1D({{5,2,4,5,6,3}}), bitpit::ElementType::POLYGON, long(1), long(1), rank);
mesh->addConnectedCell(livector1D({{5,4,9,10,7,5}}), bitpit::ElementType::POLYGON, long(0), long(2), rank);
mesh->addConnectedCell(livector1D({{7,10,11,8}}), bitpit::ElementType::QUAD, long(1), long(4), rank);
mesh->addConnectedCell(livector1D({{6,8,11}}), bitpit::ElementType::TRIANGLE, long(0), long(5), rank);
mesh->addConnectedCell(livector1D({{5,9,12,13,11,10}}), bitpit::ElementType::POLYGON, long(1), long(6), rank);
mesh->addConnectedCell(livector1D({{12,14,15,13}}), bitpit::ElementType::QUAD, long(0), long(7), rank);
mesh->addConnectedCell(livector1D({{14,16,17,15}}), bitpit::ElementType::QUAD, long(1), long(8), rank);
mesh->addConnectedCell(livector1D({{16,20,21,17}}), bitpit::ElementType::QUAD, long(0), long(9), rank);
mesh->addConnectedCell(livector1D({{6, 20,22, 23,24,25,21}}), bitpit::ElementType::POLYGON, long(1), long(10), rank);
mesh->addConnectedCell(livector1D({{22,26,27,23}}), bitpit::ElementType::QUAD, long(1), long(11), rank);
mesh->addConnectedCell(livector1D({{23,27,28,24}}), bitpit::ElementType::QUAD, long(0), long(12), rank);
mesh->addConnectedCell(livector1D({{24,28,29,25}}), bitpit::ElementType::QUAD, long(1), long(13), rank);
mesh->addConnectedCell(livector1D({{6,26,30,31,29,28,27}}), bitpit::ElementType::POLYGON, long(1), long(14), rank);
mesh->addConnectedCell(livector1D({{30,32,33,31}}), bitpit::ElementType::QUAD, long(0), long(15), rank);
}
mesh->update();
return mesh;
}
// core function
void test00001() {
/*
* Create a surface polygonal mesh with sub-part MIO pidded as PID=1
*/
mimmo::MimmoSharedPointer<mimmo::MimmoObject> geo = createMIOMesh();
#if MIMMO_ENABLE_MPI
/*
Distribute geo among the processes.
*/
std::unique_ptr<mimmo::Partition> partition(new mimmo::Partition());
partition->setPartitionMethod(mimmo::PartitionMethod::PARTGEOM);
partition->setPlotInExecution(true);
partition->setGeometry(geo);
#endif
/*
* extract subpart MIO with SelectionByPID
MPI version will absorb distributed geo from partition block input connection.
Serial case will require an explicit set of the target geometry
*/
sel->setName("PIDExtraction");
#if MIMMO_ENABLE_MPI==0
sel->setGeometry(geo);
#endif
sel->setPID(1);
sel->setDual(false);
sel->setPlotInExecution(true);
/*
* Isolate M from MIO sub part using a plane clipping.
The block will require the input of a point and normal to define the plane
InsideOut will control the emispace active for the clipping
*/
clip->setName("PlaneClipping");
clip->setOrigin({{1.1,0.0,0.0}});
clip->setNormal({{1.0,0.0,0.0}});
clip->setInsideOut(true);
clip->setPlotInExecution(true);
/*
* Triangulate the polygonal tessellation of the M subpart
*/
triang->setName("TriangulateSurface");
triang->setPlotInExecution(true);
/*
Define block pin connections.
*/
#if MIMMO_ENABLE_MPI
#endif
/*
Setup execution chain.
*/
mimmo::Chain ch0;
#if MIMMO_ENABLE_MPI
ch0.addObject(partition.get());
#endif
ch0.addObject(sel.get());
ch0.addObject(clip.get());
ch0.addObject(triang.get());
/*
Execute the chain.
Use debug flag true to to print out the execution steps.
*/
return;
}
// =================================================================================== //
int main( int argc, char *argv[] ) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI
MPI_Init(&argc, &argv);
#endif
try{
test00001();
}
catch(std::exception & e){
std::cout<<"geohandlers_example_00004 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI
MPI_Finalize();
#endif
return 0;
}
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
ClipGeometry is a class that clip a 3D geometry according to a plane intersecting it.
Definition: ClipGeometry.hpp:85
Triangulate a target MimmoObject non-homogeneous and/or non-triangular surface mesh.
Definition: SurfaceTriangulator.hpp:74
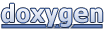