Selection using target mesh Part Identifiers. More...
#include <MeshSelection.hpp>
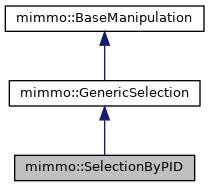
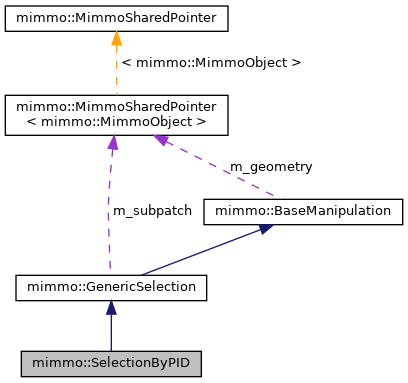
Protected Member Functions | |
livector1D | extractSelection () |
void | swap (SelectionByPID &) noexcept |
![]() | |
void | swap (GenericSelection &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_dual |
mimmo::MimmoSharedPointer< MimmoObject > | m_subpatch |
int | m_topo |
SelectionType | m_type |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Selection using target mesh Part Identifiers.
Selection Object managing selection of sub-patches of MimmoObject data structure supporting Part IDentifiers (PID). Extract portion of mesh getting its PID in target geometry. Point clouds are not suitable for this selection method.
Ports available in SelectionByPID Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORLI2 | setPID | (MC_VECTOR, MD_LONG) |
M_VALUELI | setPID | (MC_SCALAR, MD_LONG) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
Inherited from GenericSelection
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | setDual | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORLI | constrainedBoundary | (MC_VECTOR, MD_LONG) |
M_GEOM | getPatch | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.SelectionByMapping
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Dual: boolean to get straight what given by selection method or its exact dual;
- nPID: number of PID to be selected relative to target geometry;
- PID: list of PID (separated by blank spaces) to be selected relative to target geometry;
Geometry has to be mandatorily passed through port.
- Examples
- geohandlers_example_00004.cpp, and iocgns_example_00001.cpp.
Definition at line 563 of file MeshSelection.hpp.
Constructor & Destructor Documentation
◆ SelectionByPID() [1/4]
mimmo::SelectionByPID::SelectionByPID | ( | ) |
Basic Constructor
Definition at line 32 of file SelectionByPID.cpp.
◆ SelectionByPID() [2/4]
mimmo::SelectionByPID::SelectionByPID | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 40 of file SelectionByPID.cpp.
◆ SelectionByPID() [3/4]
mimmo::SelectionByPID::SelectionByPID | ( | livector1D & | pidlist, |
mimmo::MimmoSharedPointer< MimmoObject > | target | ||
) |
Custom Constructor
- Parameters
-
[in] pidlist List of pid to be included into selection [in] target Pointer to target geometry
Definition at line 59 of file SelectionByPID.cpp.
◆ ~SelectionByPID()
|
virtual |
Destructor
Definition at line 68 of file SelectionByPID.cpp.
◆ SelectionByPID() [4/4]
mimmo::SelectionByPID::SelectionByPID | ( | const SelectionByPID & | other | ) |
Copy constructor
Definition at line 73 of file SelectionByPID.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 306 of file SelectionByPID.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Reimplemented from mimmo::GenericSelection.
Definition at line 102 of file SelectionByPID.cpp.
◆ clear()
void mimmo::SelectionByPID::clear | ( | ) |
Clear your class
Definition at line 218 of file SelectionByPID.cpp.
◆ extractSelection()
|
protectedvirtual |
Extract portion of target geometry which are enough near to the external geometry provided
- Returns
- ids of cell of target tessellation extracted
Implements mimmo::GenericSelection.
Definition at line 229 of file SelectionByPID.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 357 of file SelectionByPID.cpp.
◆ getActivePID()
livector1D mimmo::SelectionByPID::getActivePID | ( | bool | active = true | ) |
Return active/inactive PIDs for current geometry linked.
- Parameters
-
[in] active boolean to get active-true, inactive false PID for selection
- Returns
- active/inactive PIDs for current geometry linked.
Definition at line 135 of file SelectionByPID.cpp.
◆ getPID()
livector1D mimmo::SelectionByPID::getPID | ( | ) |
Return all available PID for current geometry linked. Void list means not linked target geometry
- Returns
- vector with PIDs for current geometry
Definition at line 120 of file SelectionByPID.cpp.
◆ operator=()
SelectionByPID & mimmo::SelectionByPID::operator= | ( | SelectionByPID | other | ) |
Copy Operator
Definition at line 81 of file SelectionByPID.cpp.
◆ removePID() [1/2]
void mimmo::SelectionByPID::removePID | ( | livector1D | list | ) |
Deactivate a list of flagged PID i. SelectionByPID::removePID(long i = -1). Modifications are available after applying them with syncPIDList method;
- Parameters
-
[in] list list of PID to be deactivated
Definition at line 208 of file SelectionByPID.cpp.
◆ removePID() [2/2]
void mimmo::SelectionByPID::removePID | ( | long | i = -1 | ) |
Deactivate flagged PID i. If i<0, deactivates all PIDs available. if i > 0 but does not exist in PID available list, do nothing Modifications are available after applying them with syncPIDList method;
- Parameters
-
[in] i PID to be deactivated.
Definition at line 194 of file SelectionByPID.cpp.
◆ setGeometry()
|
virtual |
Set pointer to your target geometry. Reimplemented from mimmo::BaseManipulation::setGeometry()
- Parameters
-
[in] target Pointer to MimmoObject target geometry.
Reimplemented from mimmo::GenericSelection.
Definition at line 149 of file SelectionByPID.cpp.
◆ setPID() [1/2]
void mimmo::SelectionByPID::setPID | ( | livector1D | list | ) |
Activate a list of flagged PID i. SelectionByPID::setPID(long i). Modifications are available after applying them with syncPIDList method;
- Parameters
-
[in] list list of PID to be activated
Definition at line 181 of file SelectionByPID.cpp.
◆ setPID() [2/2]
void mimmo::SelectionByPID::setPID | ( | long | i = -1 | ) |
Activate flagged PID i. If i<0, activates all PIDs available. Modifications are available after applying them with syncPIDList method;
- Parameters
-
[in] i PID to be activated.
- Examples
- iocgns_example_00001.cpp.
Definition at line 169 of file SelectionByPID.cpp.
◆ swap()
|
protectednoexcept |
Swap function. Assign data of this class to another of the same type and vice-versa. Resulting patch of selection is not swapped, ever.
- Parameters
-
[in] x SelectionByPID object
Definition at line 91 of file SelectionByPID.cpp.
◆ syncPIDList()
void mimmo::SelectionByPID::syncPIDList | ( | ) |
Checks & synchronizes user given pid list w/ current pid list available by linked geometry. Activate positive matching PIDs and erase negative matches from user list. if geometry is not currently linked does nothing.
Definition at line 274 of file SelectionByPID.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/MeshSelection.hpp
- src/geohandlers/SelectionByPID.cpp
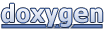