Selection mapping external surfaces/volume/3D curves on a target mesh of the same topology. More...
#include <MeshSelection.hpp>
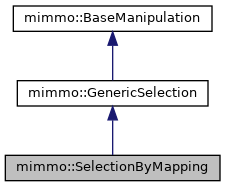
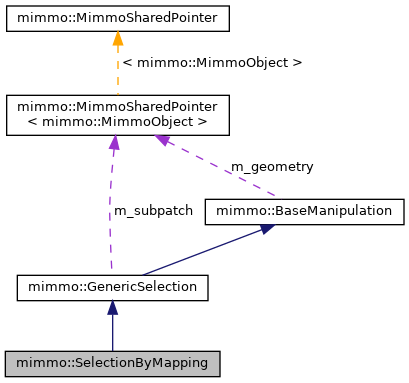
Public Member Functions | |
SelectionByMapping (const bitpit::Config::Section &rootXML) | |
SelectionByMapping (const SelectionByMapping &other) | |
SelectionByMapping (int topo=1) | |
SelectionByMapping (std::unordered_map< std::string, int > &geolist, mimmo::MimmoSharedPointer< MimmoObject > target, double tolerance) | |
virtual | ~SelectionByMapping () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | addFile (std::pair< std::string, int >) |
void | addMappingGeometry (mimmo::MimmoSharedPointer< MimmoObject > obj) |
void | buildPorts () |
void | clear () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
const std::unordered_map< std::string, int > & | getFiles () const |
double | getTolerance () |
SelectionByMapping & | operator= (SelectionByMapping other) |
void | removeFile (std::string) |
void | removeFiles () |
void | removeMappingGeometries () |
void | setFiles (std::unordered_map< std::string, int >) |
void | setGeometry (mimmo::MimmoSharedPointer< MimmoObject > geometry) |
void | setTolerance (double tol=1.e-8) |
![]() | |
GenericSelection () | |
GenericSelection (const GenericSelection &other) | |
virtual | ~GenericSelection () |
livector1D | constrainedBoundary () |
void | execute () |
mimmo::MimmoSharedPointer< MimmoObject > | getPatch () |
const mimmo::MimmoSharedPointer< MimmoObject > | getPatch () const |
bool | isDual () |
GenericSelection & | operator= (const GenericSelection &other) |
virtual void | plotOptionalResults () |
void | setDual (bool flag=false) |
SelectionType | whichMethod () |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
livector1D | extractSelection () |
void | swap (SelectionByMapping &) noexcept |
![]() | |
void | swap (GenericSelection &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_dual |
mimmo::MimmoSharedPointer< MimmoObject > | m_subpatch |
int | m_topo |
SelectionType | m_type |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Selection mapping external surfaces/volume/3D curves on a target mesh of the same topology.
Selection Object managing selection of sub-patches of a MimmoObject unstructured surface mesh, unstructured volume mesh or 3D Curve mesh. Extract portion of mesh in common between a target geometry and a second one of the same topology, provided externally. Extraction criterium is based on euclidean nearness, within a prescribed tolerance. Point clouds are not suitable for this selection method.
Ports available in SelectionByMapping Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM2 | addMappingGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
Inherited from GenericSelection
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | setDual | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORLI | constrainedBoundary | (MC_VECTOR, MD_LONG) |
M_GEOM | getPatch | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.SelectionByMapping
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Topology: number indentifying topology of tesselated mesh. 1-surfaces, 2-voume. no other types are supported;
- Dual: boolean to get straight what given by selection method or its exact dual;
- Tolerance: proximity threshold to activate mapping;
- Files: list of external files to map on the target surface:
<Files>
<file0>
<fullpath> absolute path to your file with extension <fullpath>
<tag> tag extension as supported format STL,NAS,STVTU,etc...<tag>
<file0>
<file1>
<fullpath> absolute path to your file with extension <fullpath>
<tag> tag extension as supported format STL,NAS,STVTU,etc...<tag>
<file1>
...
</Files>
Geometry has to be mandatorily passed through port.
- Examples
- geohandlers_example_00003.cpp.
Definition at line 458 of file MeshSelection.hpp.
Constructor & Destructor Documentation
◆ SelectionByMapping() [1/4]
mimmo::SelectionByMapping::SelectionByMapping | ( | int | topo = 1 | ) |
Basic Constructor. Need to know kind of topology chosen 1-3D surface, 2-VolumeMesh, 4-3DCurve. Other options are not available, if forced trigger default value of 1.
Definition at line 36 of file SelectionByMapping.cpp.
◆ SelectionByMapping() [2/4]
mimmo::SelectionByMapping::SelectionByMapping | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 72 of file SelectionByMapping.cpp.
◆ SelectionByMapping() [3/4]
mimmo::SelectionByMapping::SelectionByMapping | ( | std::unordered_map< std::string, int > & | geolist, |
mimmo::MimmoSharedPointer< MimmoObject > | target, | ||
double | tolerance | ||
) |
Custom Constructor. Not empty geometry set also the topology allowed by the class. Point Clouds are skipped to default topology (3D surface mesh).
- Parameters
-
[in] geolist List of external files to read from, for comparison purposes [in] target Pointer to target geometry [in] tolerance Proximity criterium tolerance
Definition at line 126 of file SelectionByMapping.cpp.
◆ ~SelectionByMapping()
|
virtual |
Destructor
Definition at line 166 of file SelectionByMapping.cpp.
◆ SelectionByMapping() [4/4]
mimmo::SelectionByMapping::SelectionByMapping | ( | const SelectionByMapping & | other | ) |
copy Constructor
Definition at line 171 of file SelectionByMapping.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 480 of file SelectionByMapping.cpp.
◆ addFile()
void mimmo::SelectionByMapping::addFile | ( | std::pair< std::string, int > | file | ) |
Add a new file to a list of external geometry files
- Parameters
-
[in] file External file to be read
Definition at line 291 of file SelectionByMapping.cpp.
◆ addMappingGeometry()
void mimmo::SelectionByMapping::addMappingGeometry | ( | mimmo::MimmoSharedPointer< MimmoObject > | obj | ) |
Add a new mimmo object to a list of external geometries (only surface mesh allowed)
- Parameters
-
[in] obj Pointer to MimmoObject of external geometry
Definition at line 303 of file SelectionByMapping.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Reimplemented from mimmo::GenericSelection.
Definition at line 208 of file SelectionByMapping.cpp.
◆ clear()
void mimmo::SelectionByMapping::clear | ( | ) |
Clear your class
Definition at line 338 of file SelectionByMapping.cpp.
◆ extractSelection()
|
protectedvirtual |
Extract portion of target geometry which are enough near to the external geometry provided
- Returns
- ids of cell of target tessellation extracted
Implements mimmo::GenericSelection.
Definition at line 350 of file SelectionByMapping.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 561 of file SelectionByMapping.cpp.
◆ getFiles()
const std::unordered_map< std::string, int > & mimmo::SelectionByMapping::getFiles | ( | ) | const |
Return the actual list of external geometry files to read from and compare your target geometry for mapping purposes
- Returns
- the list of external files to read
Definition at line 271 of file SelectionByMapping.cpp.
◆ getTolerance()
double mimmo::SelectionByMapping::getTolerance | ( | ) |
Return tolerance actually set in your class
- Returns
- tolerance set in your class
Definition at line 223 of file SelectionByMapping.cpp.
◆ operator=()
SelectionByMapping & mimmo::SelectionByMapping::operator= | ( | SelectionByMapping | other | ) |
Copy Operator
Definition at line 182 of file SelectionByMapping.cpp.
◆ removeFile()
void mimmo::SelectionByMapping::removeFile | ( | std::string | file | ) |
Remove an existent file to a list of external geometry files. If not in the list, do nothing
- Parameters
-
[in] file Name of the file to be removed from the list
Definition at line 314 of file SelectionByMapping.cpp.
◆ removeFiles()
void mimmo::SelectionByMapping::removeFiles | ( | ) |
Empty your list of file for mapping
Definition at line 322 of file SelectionByMapping.cpp.
◆ removeMappingGeometries()
void mimmo::SelectionByMapping::removeMappingGeometries | ( | ) |
Empty your list of geometries for mapping
Definition at line 330 of file SelectionByMapping.cpp.
◆ setFiles()
void mimmo::SelectionByMapping::setFiles | ( | std::unordered_map< std::string, int > | files | ) |
Set a list of external geometry files to read from and compare your target geometry for mapping purposes
- Parameters
-
[in] files List external geometries to be read.
Definition at line 280 of file SelectionByMapping.cpp.
◆ setGeometry()
|
virtual |
Set link to target geometry for your selection. Reimplementation of GenericSelection::setGeometry();
- Parameters
-
[in] target Pointer to target geometry.
Reimplemented from mimmo::GenericSelection.
Definition at line 246 of file SelectionByMapping.cpp.
◆ setTolerance()
void mimmo::SelectionByMapping::setTolerance | ( | double | tol = 1.e-8 | ) |
Set Proximity tolerance. Under this threshold compared geometries are considered coincident.
- Parameters
-
[in] tol Desired tolerance.
- Examples
- geohandlers_example_00003.cpp.
Definition at line 233 of file SelectionByMapping.cpp.
◆ swap()
|
protectednoexcept |
Swap function. Assign data of this class to another of the same type and vice-versa. Resulting patch of selection is not swapped, ever.
- Parameters
-
[in] x SelectionByMapping object
Definition at line 192 of file SelectionByMapping.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/MeshSelection.hpp
- src/geohandlers/SelectionByMapping.cpp
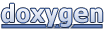