MeshSelection.hpp
277 SelectionByCylinder(darray3E origin, darray3E span, double infLimTheta, darray3E mainAxis, mimmo::MimmoSharedPointer<MimmoObject> target);
368 SelectionBySphere(darray3E origin, darray3E span, double infLimTheta, double infLimPhi, mimmo::MimmoSharedPointer<MimmoObject> target);
470 SelectionByMapping(std::unordered_map<std::string, int> & geolist, mimmo::MimmoSharedPointer<MimmoObject> target, double tolerance);
687 SelectionByBoxWithScalar(darray3E origin, darray3E span, mimmo::MimmoSharedPointer<MimmoObject> target);
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByElementList.cpp:253
void setTolerance(double tol=1.e-8)
Definition: SelectionByMapping.cpp:233
livector1D extractSelection()
Definition: SelectionByPID.cpp:229
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByCylinder.cpp:159
void addRawVertexList(std::vector< long > vertexdata)
Definition: SelectionByElementList.cpp:134
livector1D extractSelection()
Definition: SelectionByElementList.cpp:161
livector1D extractSelection()
Definition: SelectionByCylinder.cpp:138
void setField(dmpvector1D *)
Definition: SelectionByBoxWithScalar.cpp:129
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject > geometry)
Definition: SelectionByMapping.cpp:246
void addFile(std::pair< std::string, int >)
Definition: SelectionByMapping.cpp:291
SelectionByPID & operator=(SelectionByPID other)
Definition: SelectionByPID.cpp:81
@ MAPPING
void removeFile(std::string)
Definition: SelectionByMapping.cpp:314
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBoxWithScalar.cpp:204
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBoxWithScalar.cpp:192
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByPID.cpp:306
@ UNDEFINED
void swap(SelectionByMapping &) noexcept
Definition: SelectionByMapping.cpp:192
Selection through list of cells/vertices of the target mesh.
Definition: MeshSelection.hpp:769
const std::unordered_map< std::string, int > & getFiles() const
Definition: SelectionByMapping.cpp:271
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBox.cpp:241
virtual ~SelectionByBoxWithScalar()
Definition: SelectionByBoxWithScalar.cpp:72
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByMapping.cpp:480
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
void buildPorts()
Definition: SelectionByBoxWithScalar.cpp:105
Elementary Shape Representation of a Sphere or portion of it.
Definition: BasicShapes.hpp:322
std::vector< MimmoPiercedVector< long > * > m_annotatedvertices
Definition: MeshSelection.hpp:790
Elementary Shape Representation of a Cylinder or portion of it.
Definition: BasicShapes.hpp:288
SelectionType
Enum class for choiche of method to select sub-patch of a MimmoObject tessellated mesh.
Definition: MeshSelection.hpp:43
Selection mapping external surfaces/volume/3D curves on a target mesh of the same topology.
Definition: MeshSelection.hpp:458
std::vector< MimmoPiercedVector< long > * > m_annotatedcells
Definition: MeshSelection.hpp:789
SelectionByMapping(int topo=1)
Definition: SelectionByMapping.cpp:36
livector1D extractSelection()
Definition: SelectionBySphere.cpp:137
virtual ~SelectionBySphere()
Definition: SelectionBySphere.cpp:79
@ BOXwSCALAR
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
@ SPHERE
@ CYLINDER
livector1D extractSelection()
Definition: SelectionByMapping.cpp:350
virtual ~GenericSelection()
Definition: GenericSelection.cpp:41
livector1D getActivePID(bool active=true)
Definition: SelectionByPID.cpp:135
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByMapping.cpp:561
SelectionByMapping & operator=(SelectionByMapping other)
Definition: SelectionByMapping.cpp:182
void swap(SelectionByElementList &) noexcept
Definition: SelectionByElementList.cpp:65
void setFiles(std::unordered_map< std::string, int >)
Definition: SelectionByMapping.cpp:280
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBox.cpp:153
virtual ~SelectionByCylinder()
Definition: SelectionByCylinder.cpp:78
void swap(SelectionByCylinder &) noexcept
Definition: SelectionByCylinder.cpp:99
void addMappingGeometry(mimmo::MimmoSharedPointer< MimmoObject > obj)
Definition: SelectionByMapping.cpp:303
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
Selection through volume box primitive.
Definition: MeshSelection.hpp:678
void addAnnotatedVertexList(MimmoPiercedVector< long > *vertexdata)
Definition: SelectionByElementList.cpp:110
const mimmo::MimmoSharedPointer< MimmoObject > getPatch() const
Definition: GenericSelection.cpp:111
virtual void plotOptionalResults()
Definition: GenericSelection.cpp:282
virtual ~SelectionByMapping()
Definition: SelectionByMapping.cpp:166
void addAnnotatedCellList(MimmoPiercedVector< long > *celldata)
Definition: SelectionByElementList.cpp:97
SelectionBySphere & operator=(SelectionBySphere other)
Definition: SelectionBySphere.cpp:90
SelectionByBoxWithScalar()
Definition: SelectionByBoxWithScalar.cpp:33
std::vector< std::vector< long > > m_rawvertices
Definition: MeshSelection.hpp:792
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionBySphere.cpp:258
SelectionByCylinder & operator=(SelectionByCylinder other)
Definition: SelectionByCylinder.cpp:89
void plotOptionalResults()
Definition: SelectionByBoxWithScalar.cpp:179
std::vector< std::vector< long > > m_rawcells
Definition: MeshSelection.hpp:791
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionBySphere.cpp:156
SelectionByBox & operator=(SelectionByBox other)
Definition: SelectionByBox.cpp:84
void swap(SelectionByBoxWithScalar &) noexcept
Definition: SelectionByBoxWithScalar.cpp:94
SelectionByBoxWithScalar & operator=(SelectionByBoxWithScalar other)
Definition: SelectionByBoxWithScalar.cpp:84
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: SelectionByPID.cpp:149
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByElementList.cpp:229
virtual void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: GenericSelection.cpp:130
dmpvector1D * getField()
Definition: SelectionByBoxWithScalar.cpp:138
GenericSelection & operator=(const GenericSelection &other)
Definition: GenericSelection.cpp:57
SelectionByElementList()
Definition: SelectionByElementList.cpp:32
virtual livector1D extractSelection()=0
void swap(SelectionBySphere &) noexcept
Definition: SelectionBySphere.cpp:100
virtual ~SelectionByElementList()
Definition: SelectionByElementList.cpp:57
livector1D extractSelection()
Definition: SelectionByBox.cpp:133
void addRawCellList(std::vector< long > celldata)
Definition: SelectionByElementList.cpp:122
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByPID.cpp:357
livector1D constrainedBoundary()
Definition: GenericSelection.cpp:176
SelectionByCylinder()
Definition: SelectionByCylinder.cpp:33
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByCylinder.cpp:261
void removeMappingGeometries()
Definition: SelectionByMapping.cpp:330
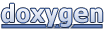