SelectionByElementList.cpp
83 built = (built && createPortIn<MimmoPiercedVector<long> *, SelectionByElementList>(this, &SelectionByElementList::addAnnotatedCellList, M_LONGFIELD2,true,2 ));
84 built = (built && createPortIn<MimmoPiercedVector<long> *, SelectionByElementList>(this, &SelectionByElementList::addAnnotatedVertexList, M_LONGFIELD,true,2 ));
85 built = (built && createPortIn<std::vector<long>, SelectionByElementList>(this, &SelectionByElementList::addRawCellList, M_VECTORLI3,true,2 ));
86 built = (built && createPortIn<std::vector<long>, SelectionByElementList>(this, &SelectionByElementList::addRawVertexList, M_VECTORLI2,true,2 ));
229 SelectionByElementList::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByElementList.cpp:253
void addRawVertexList(std::vector< long > vertexdata)
Definition: SelectionByElementList.cpp:134
livector1D extractSelection()
Definition: SelectionByElementList.cpp:161
Selection through list of cells/vertices of the target mesh.
Definition: MeshSelection.hpp:769
@ CELL
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
std::vector< MimmoPiercedVector< long > * > m_annotatedvertices
Definition: MeshSelection.hpp:790
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
std::vector< MimmoPiercedVector< long > * > m_annotatedcells
Definition: MeshSelection.hpp:789
void swap(SelectionByElementList &) noexcept
Definition: SelectionByElementList.cpp:65
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
void addAnnotatedVertexList(MimmoPiercedVector< long > *vertexdata)
Definition: SelectionByElementList.cpp:110
@ POINT
void addAnnotatedCellList(MimmoPiercedVector< long > *celldata)
Definition: SelectionByElementList.cpp:97
std::vector< std::vector< long > > m_rawvertices
Definition: MeshSelection.hpp:792
std::vector< std::vector< long > > m_rawcells
Definition: MeshSelection.hpp:791
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByElementList.cpp:229
SelectionByElementList()
Definition: SelectionByElementList.cpp:32
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
virtual ~SelectionByElementList()
Definition: SelectionByElementList.cpp:57
void addRawCellList(std::vector< long > celldata)
Definition: SelectionByElementList.cpp:122
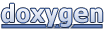