BaseManipulation.hpp
107 friend bool pin::addPin(BaseManipulation* objSend, BaseManipulation* objRec, PortID portS, PortID portR, bool forced);
111 friend void pin::removePin(BaseManipulation* objSend, BaseManipulation* objRec, PortID portS, PortID portR);
119 friend bool pin::checkCompatibility(BaseManipulation* objSend, BaseManipulation* objRec, PortID portS, PortID portR);
260 bool createPortIn(O* obj, void (O::*setVar_)(T), PortID portR, bool mandatory = false, int family = 0);
295 void write(MimmoSharedPointer<MimmoObject> geometry, MimmoPiercedVector<mpv_t> & data, Args ... args);
301 void write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>> & data, Args ... args);
304 void write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>> & data);
307 void write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>*> & data, Args ... args);
310 void write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>*> & data);
PortID findPinOut(PortOut &pin)
Definition: BaseManipulation.cpp:809
void addPinOut(BaseManipulation *objOut, PortID portS, PortID portR)
Definition: BaseManipulation.cpp:833
void removePin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR)
Definition: MimmoNamespace.cpp:147
BaseManipulation * getChild(int i=0)
Definition: BaseManipulation.cpp:301
virtual void plotOptionalResults()
Definition: BaseManipulation.cpp:908
ConnectionType getConnectionType()
Definition: BaseManipulation.cpp:328
bool checkCompatibility(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR)
Definition: MimmoNamespace.cpp:165
void addParent(BaseManipulation *parent)
Definition: BaseManipulation.cpp:735
virtual void execute()=0
void removePinIn(BaseManipulation *objIn, PortID portR)
Definition: BaseManipulation.cpp:847
std::unordered_map< PortID, PortOut * > getPortsOut()
Definition: BaseManipulation.cpp:364
void setBufferIn(PortID port, mimmo::IBinaryStream &input)
Definition: BaseManipulation.cpp:707
void setOutputPlot(std::string path)
Definition: BaseManipulation.cpp:453
bool createPortIn(T *var_, PortID portR, bool mandatory=false, int family=0)
Definition: BaseManipulation.tpp:94
virtual ~BaseManipulation()
Definition: BaseManipulation.cpp:74
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void addPinIn(BaseManipulation *objIn, PortID portR)
Definition: BaseManipulation.cpp:821
BaseManipulation & operator=(const BaseManipulation &other)
Definition: BaseManipulation.cpp:113
void unsetChild(BaseManipulation *child)
Definition: BaseManipulation.cpp:780
BaseManipulation * getParent(int i=0)
Definition: BaseManipulation.cpp:264
ConnectionType
Type of allowed connections of the object: bidirectional, only input or only output.
Definition: MimmoNamespace.hpp:83
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
PortIn is the abstract PIN base class dedicated to carry data to a target class from other ones (inpu...
Definition: InOut.hpp:201
PortOut is the abstract PIN base class dedicated to exchange data from a target class to other ones (...
Definition: InOut.hpp:96
void addChild(BaseManipulation *child)
Definition: BaseManipulation.cpp:749
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
static int sm_baseManipulationCounter
Definition: BaseManipulation.hpp:164
bool isPlotInExecution()
Definition: BaseManipulation.cpp:372
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void buildPorts()=0
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
bool isParent(BaseManipulation *, int &)
Definition: BaseManipulation.cpp:276
void initializeLogger(bool logexists)
Definition: BaseManipulation.cpp:164
bool createPortOut(O *obj, T(O::*getVar_)(), PortID portS)
Definition: BaseManipulation.tpp:64
std::unordered_map< PortID, PortOut * > m_portOut
Definition: BaseManipulation.hpp:153
void cleanBufferIn(PortID port)
Definition: BaseManipulation.cpp:726
void readBufferIn(PortID port)
Definition: BaseManipulation.cpp:717
pin::ConnectionType ConnectionType
Definition: BaseManipulation.hpp:137
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void removePinOut(BaseManipulation *objOut, PortID portS)
Definition: BaseManipulation.cpp:863
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
std::unordered_map< PortID, PortIn * > m_portIn
Definition: BaseManipulation.hpp:152
bool isChild(BaseManipulation *, int &)
Definition: BaseManipulation.cpp:313
std::unordered_map< PortID, PortIn * > getPortsIn()
Definition: BaseManipulation.cpp:355
void setPriority(uint priority)
Definition: BaseManipulation.cpp:416
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void removeAllPins(BaseManipulation *objSend, BaseManipulation *objRec)
Definition: MimmoNamespace.cpp:108
MimmoSharedPointer< MimmoObject > & getGeometryReference()
Definition: BaseManipulation.cpp:245
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
std::unordered_map< BaseManipulation *, int > bmumap
Definition: BaseManipulation.hpp:136
virtual std::vector< BaseManipulation * > getSubBlocksEmbedded()
Definition: BaseManipulation.cpp:927
void unsetParent(BaseManipulation *parent)
Definition: BaseManipulation.cpp:764
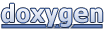