InOut.hpp
Class DataType defines the container and the type of data communicated by ports.
Definition: InOut.hpp:53
virtual void writeBuffer()=0
mimmo custom derivation of bitpit OBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:55
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
PortOutT is the PIN class to exchange output data from an object to others.
Definition: InOut.hpp:155
PortIn is the abstract PIN base class dedicated to carry data to a target class from other ones (inpu...
Definition: InOut.hpp:201
PortOut is the abstract PIN base class dedicated to exchange data from a target class to other ones (...
Definition: InOut.hpp:96
DataType & operator=(const DataType &)=default
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
virtual void readBuffer()=0
PortInT is the PIN class to get input data arriving to an object from other objects.
Definition: InOut.hpp:264
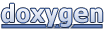