InOut.tpp
206 PortInT<T,O>::PortInT(O* obj_, void (O::*setVar_)(T), DataType datatype, bool mandatory, int family){
Class DataType defines the container and the type of data communicated by ports.
Definition: InOut.hpp:53
PortOutT is the PIN class to exchange output data from an object to others.
Definition: InOut.hpp:155
PortIn is the abstract PIN base class dedicated to carry data to a target class from other ones (inpu...
Definition: InOut.hpp:201
PortOut is the abstract PIN base class dedicated to exchange data from a target class to other ones (...
Definition: InOut.hpp:96
PortInT is the PIN class to get input data arriving to an object from other objects.
Definition: InOut.hpp:264
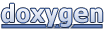