MimmoPiercedVector is the basic data container for mimmo library. More...
#include <MimmoPiercedVector.hpp>
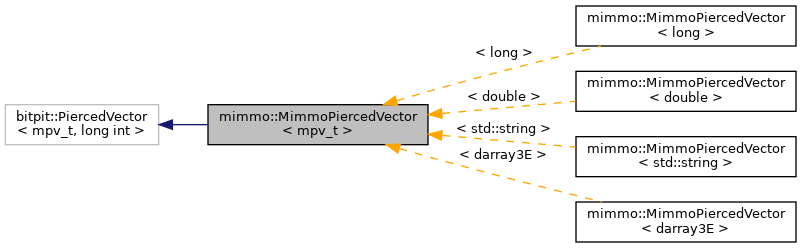
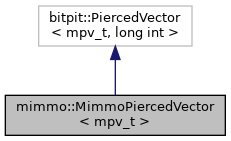
Public Member Functions | |
MimmoPiercedVector (const MimmoPiercedVector< mpv_t > &other) | |
MimmoPiercedVector (MimmoSharedPointer< MimmoObject > geo=nullptr, MPVLocation loc=MPVLocation::UNDEFINED) | |
virtual | ~MimmoPiercedVector () |
MimmoPiercedVector | cellDataToPointData (const MimmoPiercedVector< mpv_t > &cellGradientsX, const MimmoPiercedVector< mpv_t > &cellGradientsY, const MimmoPiercedVector< mpv_t > &cellGradientsZ, bool maximum=false) |
MimmoPiercedVector | cellDataToPointData (double p=0.) |
bool | checkDataIdsCoherence () |
bool | checkDataSizeCoherence () |
void | clear () |
bool | completeMissingData (const mpv_t &defValue) |
MPVLocation | getConstDataLocation () const |
std::vector< mpv_t > | getDataAsVector (bool ordered=false) |
std::size_t | getDataFrom (const MimmoPiercedVector< mpv_t > &other, bool strict=false) |
MPVLocation | getDataLocation () |
MimmoSharedPointer< MimmoObject > | getGeometry () const |
std::vector< mpv_t > | getInternalDataAsVector (bool ordered=false, bool squeeze=true) |
std::string | getName () const |
std::vector< mpv_t > | getRawDataAsVector (bool ordered=false) |
void | initialize (MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &) |
bool | intIsValidLocation (int &) |
bool | isEmpty () |
MimmoPiercedVector & | operator= (bitpit::PiercedVector< mpv_t, long int > other) |
MimmoPiercedVector & | operator= (MimmoPiercedVector< mpv_t > other) |
MimmoPiercedVector | pointDataToBoundaryInterfaceData (double p=0.) |
MimmoPiercedVector | pointDataToCellData (double p=0.) |
MimmoPiercedVector< mpv_t > | resizeToCoherentDataIds () |
void | setData (std::vector< mpv_t > &rawdata) |
void | setDataLocation (int loc) |
void | setDataLocation (MPVLocation loc) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geo) |
void | setName (std::string name) |
void | squeezeOutExcept (const std::unordered_set< long int > &list, bool keepOrder=false) |
void | squeezeOutExcept (const std::vector< long int > &list, bool keepOrder=false) |
void | swap (MimmoPiercedVector< mpv_t > &x) noexcept |
Detailed Description
template<typename mpv_t>
class mimmo::MimmoPiercedVector< mpv_t >
MimmoPiercedVector is the basic data container for mimmo library.
MimmoPiercedVector is the basic container for data attached to a geometry defined as a MimmoObject. It is based on bitpit::PiercedVector container. It supports interface methods to recover the related geometric object. It supports a string name attribute to mark the field as well as a location enum to understand to which structures of geometry refers the data (UNDEFINED no-info, POINT-vertices, CELL-cells, INTERFACE-interfaces).
- Examples
- test_propagators_00001.cpp, and test_propagators_00003.cpp.
Definition at line 60 of file MimmoPiercedVector.hpp.
Constructor & Destructor Documentation
◆ MimmoPiercedVector() [1/2]
mimmo::MimmoPiercedVector< mpv_t >::MimmoPiercedVector | ( | MimmoSharedPointer< MimmoObject > | geo = nullptr , |
MPVLocation | loc = MPVLocation::UNDEFINED |
||
) |
Default constructor of MimmoPiercedVector.
- Parameters
-
[in] geo mimmo shared pointer to related geometry [in] loc reference location of field. see MPVLocation.
Definition at line 91 of file MimmoPiercedVector.tpp.
◆ ~MimmoPiercedVector()
|
virtual |
Default destructor of MimmoPiercedVector.
Definition at line 102 of file MimmoPiercedVector.tpp.
◆ MimmoPiercedVector() [2/2]
mimmo::MimmoPiercedVector< mpv_t >::MimmoPiercedVector | ( | const MimmoPiercedVector< mpv_t > & | other | ) |
Copy Constructor
- Parameters
-
[in] other MimmoPiercedVector object
Definition at line 109 of file MimmoPiercedVector.tpp.
Member Function Documentation
◆ cellDataToPointData() [1/2]
MimmoPiercedVector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::cellDataToPointData | ( | const MimmoPiercedVector< mpv_t > & | cellGradientsX, |
const MimmoPiercedVector< mpv_t > & | cellGradientsY, | ||
const MimmoPiercedVector< mpv_t > & | cellGradientsZ, | ||
bool | maximum = false |
||
) |
Cell data to Point data interpolation by using gradients on cell centers. A inverse cell volume weighted extrapolation of reconstructed values is placed on points. The current object is a MimmoPiercedVector object with data located on MPVLocation::CELL
- Parameters
-
[in] cellGradientsX x-gradient comp on cell centers [in] cellGradientsY y-gradient comp on cell centers [in] cellGradientsZ z-gradient comp on cell centers [in] maximum boolean true, use simple averaging, false cubic-distance weighted averaging.
- Returns
- point data MimmoPiercedVector object located on MPVLocation::POINT
Note. In parallel data on ghost points are not correctly interpolated; external communication to fix the values is needed.
Definition at line 728 of file MimmoPiercedVector.tpp.
◆ cellDataToPointData() [2/2]
MimmoPiercedVector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::cellDataToPointData | ( | double | p = 0. | ) |
Cell data to Point data interpolation. Average of cell center data is set on point. The current object is a MimmoPiercedVector object with data located on MPVLocation::CELL
- Parameters
-
[in] p exponent value of inverse distance exponential weight w = (1/d^p)
- Returns
- point data MimmoPiercedVector object located on MPVLocation::POINT
Note. In parallel data on ghost points are not correctly interpolated; external communication to fix the values is needed.
Definition at line 682 of file MimmoPiercedVector.tpp.
◆ checkDataIdsCoherence()
bool mimmo::MimmoPiercedVector< mpv_t >::checkDataIdsCoherence |
Check data coherence with the geometry linked. Return a coherence boolean flag which is false if:
- UNDEFINED location is set for the current data.
- all internal m_data ids does not match those available in the relative geometry reference structure: vertex, cell or interfaces
- no geometry is linked
- Returns
- boolean coherence flag
Definition at line 412 of file MimmoPiercedVector.tpp.
◆ checkDataSizeCoherence()
bool mimmo::MimmoPiercedVector< mpv_t >::checkDataSizeCoherence |
Check data coherence with the geometry linked. Return a coherence boolean flag which is false if:
- UNDEFINED location is set to the current data
- internal m_data does not match the size of the relative geometry reference structure: vertex, cell or interfaces
- no geometry is linked
- Returns
- boolean coherence flag
Definition at line 378 of file MimmoPiercedVector.tpp.
◆ clear()
void mimmo::MimmoPiercedVector< mpv_t >::clear |
Clear the whole MimmoPiercedVector.
Definition at line 157 of file MimmoPiercedVector.tpp.
◆ completeMissingData()
bool mimmo::MimmoPiercedVector< mpv_t >::completeMissingData | ( | const mpv_t & | defValue | ) |
Check if container current data are coherent with the geometry linked. If it is and current data size does not match the size of the reference geometry container, attempt to complete all values in the missing ids of reference location geometry structure with a User-assigned reference value.
- Parameters
-
[in] defValue User-assigned reference value
- Returns
- true if the vector is coherent and full values aligned with geoemtry reference structure.
Definition at line 567 of file MimmoPiercedVector.tpp.
◆ getConstDataLocation()
MPVLocation mimmo::MimmoPiercedVector< mpv_t >::getConstDataLocation |
Get data location w.r.t geometry inner structures.
- Returns
- MPVLocation enum
Definition at line 180 of file MimmoPiercedVector.tpp.
◆ getDataAsVector()
std::vector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::getDataAsVector | ( | bool | ordered = false | ) |
Return data contained in inner pierced vector. Sequence follows that of reference location in geometry(vertices, cells or interfaces). If no geometry is provided, return empty result.
- Parameters
-
[in] ordered if true data will be returned in ids ascending order, otherwise they will be returned as you get iterating the internal location reference geometry PiercedVector from the beginning.
- Returns
- list of data. Data on ghost cells are retained.
Definition at line 214 of file MimmoPiercedVector.tpp.
◆ getDataFrom()
std::size_t mimmo::MimmoPiercedVector< mpv_t >::getDataFrom | ( | const MimmoPiercedVector< mpv_t > & | other, |
bool | strict = false |
||
) |
Copy data from another target MimmoPiercedVector of the same type.
- strict true: data transfer is made only on the shared ids of the structure.
- strict false: ids of target not present in the current MPV are copied also.
- Parameters
-
[in] other target structure to copy from. [in] strict boolean controlling the data transfer type
- Returns
- number of updates or brand new insertions done.
Definition at line 840 of file MimmoPiercedVector.tpp.
◆ getDataLocation()
MPVLocation mimmo::MimmoPiercedVector< mpv_t >::getDataLocation |
Get data location w.r.t geometry inner structures.
- Returns
- MPVLocation enum
Definition at line 190 of file MimmoPiercedVector.tpp.
◆ getGeometry()
MimmoSharedPointer< MimmoObject > mimmo::MimmoPiercedVector< mpv_t >::getGeometry |
Get the linked MimmoObject.
- Returns
- mimmo shared pointer to linked geometry.
Definition at line 170 of file MimmoPiercedVector.tpp.
◆ getInternalDataAsVector()
std::vector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::getInternalDataAsVector | ( | bool | ordered = false , |
bool | squeeze = true |
||
) |
Return data contained in inner pierced vector located on internal cells. Sequence follows that of reference location in geometry(vertices, cells, interfaces). If no geometry is provided, return empty result.
- Parameters
-
[in] ordered if true data will be returned in ids ascending order, otherwise they will be returned as you get iterating the internal location reference geometry PiercedVector from the beginning.
- Returns
- list of data. Only data on structures of internal cells are retained.
- Parameters
-
[in] squeeze if true the result container is squeezed once full
Definition at line 238 of file MimmoPiercedVector.tpp.
◆ getName()
std::string mimmo::MimmoPiercedVector< mpv_t >::getName |
◆ getRawDataAsVector()
std::vector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::getRawDataAsVector | ( | bool | ordered = false | ) |
Return only raw data contained in inner pierced vector. Sequence follows the internal pierced vector id-ing, without any reference to geometry structure ordering.
- Parameters
-
[in] ordered if true data will be returned in ids ascending order, otherwise they will be returned as you get iterating the class object itself from the beginning.
- Returns
- list of data
Definition at line 297 of file MimmoPiercedVector.tpp.
◆ initialize()
void mimmo::MimmoPiercedVector< mpv_t >::initialize | ( | MimmoSharedPointer< MimmoObject > | geo, |
MPVLocation | loc, | ||
const mpv_t & | data | ||
) |
Initialize your container with reference data.
- Parameters
-
[in] geo target geometry [in] loc data location [in] data reference data
if a valid geometry and coherent location are specified, create a container on all elements of specified location with constant reference data attached. Any pre-existent data will be destroyed.
Definition at line 593 of file MimmoPiercedVector.tpp.
◆ intIsValidLocation()
bool mimmo::MimmoPiercedVector< mpv_t >::intIsValidLocation | ( | int & | value | ) |
Check if a random integer number is a valid MPVLocation for the current class.
- Returns
- true if valid.
Definition at line 518 of file MimmoPiercedVector.tpp.
◆ isEmpty()
bool mimmo::MimmoPiercedVector< mpv_t >::isEmpty |
- Returns
- true if current pierced container has no element in it.
Definition at line 553 of file MimmoPiercedVector.tpp.
◆ operator=() [1/2]
MimmoPiercedVector< mpv_t > & mimmo::MimmoPiercedVector< mpv_t >::operator= | ( | bitpit::PiercedVector< mpv_t, long int > | other | ) |
Copy Operator for pierced data only. Values will be stored as is in the inner PiercedVector of the class.
- Parameters
-
[in] other PiercedVector object
Definition at line 131 of file MimmoPiercedVector.tpp.
◆ operator=() [2/2]
MimmoPiercedVector< mpv_t > & mimmo::MimmoPiercedVector< mpv_t >::operator= | ( | MimmoPiercedVector< mpv_t > | other | ) |
Assignment Operator.
- Parameters
-
[in] other MimmoPiercedVector object
Definition at line 121 of file MimmoPiercedVector.tpp.
◆ pointDataToBoundaryInterfaceData()
MimmoPiercedVector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::pointDataToBoundaryInterfaceData | ( | double | p = 0. | ) |
Point data to boundary Interface data interpolation. Average of point data is set on interface center only for border interfaces. The current object is a MimmoPiercedVector object with data located on MPVLocation::POINT
- Parameters
-
[in] p exponent value of inverse distance exponential weight w = (1/d^p)
- Returns
- boundary interface data MimmoPiercedVector object located on MPVLocation::INTERFACE
Definition at line 785 of file MimmoPiercedVector.tpp.
◆ pointDataToCellData()
MimmoPiercedVector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::pointDataToCellData | ( | double | p = 0. | ) |
Point data to Cell data interpolation. Average of point data is set on cell center. The current object is a MimmoPiercedVector object with data located on MPVLocation::POINT
- Parameters
-
[in] p exponent value of inverse distance exponential weight w = (1/d^p)
- Returns
- cell data MimmoPiercedVector object located on MPVLocation::CELL
Definition at line 645 of file MimmoPiercedVector.tpp.
◆ resizeToCoherentDataIds()
MimmoPiercedVector< mpv_t > mimmo::MimmoPiercedVector< mpv_t >::resizeToCoherentDataIds |
Return a copy of the current MPV retaining data coherent with the geometry linked. If reference geometry is nullptr or none of the items are coherent with geometry return an empty structure;
- Returns
- coherent version of current MPV.
Definition at line 458 of file MimmoPiercedVector.tpp.
◆ setData()
void mimmo::MimmoPiercedVector< mpv_t >::setData | ( | std::vector< mpv_t > & | data | ) |
Set the data of the inner PiercedVector from a row data compound. Ids will be automatically assigned.
- Parameters
-
[in] data vector to copy from
Definition at line 348 of file MimmoPiercedVector.tpp.
◆ setDataLocation() [1/2]
void mimmo::MimmoPiercedVector< mpv_t >::setDataLocation | ( | int | loc | ) |
Set the data Location through integer. If out of MPVLocation enum, set MPVLocation::UNDEFINED by default.
- Parameters
-
[in] loc int 0 to 3 to identify location.
Definition at line 335 of file MimmoPiercedVector.tpp.
◆ setDataLocation() [2/2]
void mimmo::MimmoPiercedVector< mpv_t >::setDataLocation | ( | MPVLocation | loc | ) |
Set the data Location
- Parameters
-
[in] loc MPVLocation enum
Definition at line 324 of file MimmoPiercedVector.tpp.
◆ setGeometry()
void mimmo::MimmoPiercedVector< mpv_t >::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Set the linked MimmoObject.
- Parameters
-
[in] geo mimmo shared pointer to linked geometry.
- Examples
- test_propagators_00003.cpp.
Definition at line 314 of file MimmoPiercedVector.tpp.
◆ setName()
void mimmo::MimmoPiercedVector< mpv_t >::setName | ( | std::string | name | ) |
Set name of the field.
- Parameters
-
[in] name field name
Definition at line 364 of file MimmoPiercedVector.tpp.
◆ squeezeOutExcept() [1/2]
void mimmo::MimmoPiercedVector< mpv_t >::squeezeOutExcept | ( | const std::unordered_set< long int > & | list, |
bool | keepOrder = false |
||
) |
Erase all data not contained in the target list and squeeze the structure.
- Parameters
-
[in] list of ids to save from cleaning [in] keepOrder if true keep the survivors in the same order as they appear in the original list.
Definition at line 899 of file MimmoPiercedVector.tpp.
◆ squeezeOutExcept() [2/2]
void mimmo::MimmoPiercedVector< mpv_t >::squeezeOutExcept | ( | const std::vector< long int > & | list, |
bool | keepOrder = false |
||
) |
Erase all data not contained in the target list and squeeze the structure.
- Parameters
-
[in] list of ids to save from cleaning [in] keepOrder if true keep the survivors in the same order as they appear in the original list.
Definition at line 864 of file MimmoPiercedVector.tpp.
◆ swap()
|
noexcept |
Attibutes data of an external object to the current class and vice versa. Containts of both objects will be swapped. Size of the two containers may differ.
- Parameters
-
[in] x MimmoPiercedVector to be swapped. Data type of elements of the pierced vector must be of the same type of the current class.
Definition at line 143 of file MimmoPiercedVector.tpp.
The documentation for this class was generated from the following files:
- src/core/MimmoPiercedVector.hpp
- src/core/MimmoPiercedVector.tpp
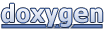