BaseManipulation.tpp
42 (*m_log)<<"Port name was not regularly registered or a port with the same name was already instantiated in the current class."<<std::endl;
71 (*m_log)<<"Port name was not regularly registered or a port with the same name was already instantiated in the current class."<<std::endl;
101 (*m_log)<<"Port name was not regularly registered or a port with the same name was already instantiated in the current class."<<std::endl;
125 BaseManipulation::createPortIn(O* obj_, void (O::*setVar_)(T), PortID portR, bool mandatory, int family ){
131 (*m_log)<<"Port name was not regularly registered or a port with the same name was already instantiated in the current class."<<std::endl;
153 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, MimmoPiercedVector<mpv_t> & data)
158 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
177 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
185 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
199 (*m_log) << " Warning: data type to write not allowed in " << m_name << "; skip data" << std::endl;
226 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, MimmoPiercedVector<mpv_t> & data, Args ... args)
230 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
248 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
256 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
270 (*m_log) << " Warning: data type to write not allowed in " << m_name << "; skip data" << std::endl;
297 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>> & vdata)
322 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
339 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
347 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
384 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>> & vdata, Args ... args)
398 (*m_log) << " Warning: data type to write not allowed in " << m_name << "; skip vector of data fields" << std::endl;
410 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
427 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
435 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
470 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>*> & vdata)
495 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
512 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
520 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
554 BaseManipulation::write(MimmoSharedPointer<MimmoObject> geometry, std::vector<MimmoPiercedVector<mpv_t>*> & vdata, Args ... args)
568 (*m_log) << " Warning: data type to write not allowed in " << m_name << "; skip vector of data fields" << std::endl;
580 (*m_log) << " Warning: data to write not consistent with geometry in " << m_name << "; skip data" << std::endl;
597 (*m_log)<<" Warning: Undefined Reference Location in plotOptionalResults of "<<m_name<<std::endl;
605 (*m_log) << " Warning: error during complete missing data to write in " << m_name << "; skip data" << std::endl;
Class DataType defines the container and the type of data communicated by ports.
Definition: InOut.hpp:53
@ CELL
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
bool createPortIn(T *var_, PortID portR, bool mandatory=false, int family=0)
Definition: BaseManipulation.tpp:94
MimmoPiercedVector is the basic data container for mimmo library.
Definition: MimmoPiercedVector.hpp:60
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
PortOutT is the PIN class to exchange output data from an object to others.
Definition: InOut.hpp:155
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
InfoPort getPortData(const std::string name)
Definition: portManager.hpp:183
bool createPortOut(O *obj, T(O::*getVar_)(), PortID portS)
Definition: BaseManipulation.tpp:64
std::unordered_map< PortID, PortOut * > m_portOut
Definition: BaseManipulation.hpp:153
@ POINT
std::unordered_map< PortID, PortIn * > m_portIn
Definition: BaseManipulation.hpp:152
std::vector< mpv_t > getDataAsVector(bool ordered=false)
Definition: MimmoPiercedVector.tpp:214
PortInT is the PIN class to get input data arriving to an object from other objects.
Definition: InOut.hpp:264
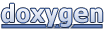