portManager.hpp
99 long addPort(const std::string name, const std::string container_name, const std::string datatype_name, const std::string fileregistration)
108 std::cerr<<"Failed Registration of Port "<<name<<" - "<<container_name<<" - "<<datatype_name<<" in file :"<<fileregistration<<std::endl;
109 std::cerr<<"It already exists as: "<<name<<" - "<<orcont<<" - "<<ordata<<" in file :"<<fileregistration<<std::endl;
213 __attribute__((unused)) static long port_##Name##_##Container##_##Datatype##_##ManipBlock = mimmo::PortManager::instance().addPort(Name, Container, Datatype, __FILE__);\
std::unordered_map< std::string, long > mapRegisteredContainers()
Definition: portManager.hpp:168
std::unordered_map< std::string, InfoPort > mapRegisteredPorts()
Definition: portManager.hpp:162
std::unordered_map< std::string, long > mapRegisteredDataTypes()
Definition: portManager.hpp:174
long addPort(const std::string name, const std::string container_name, const std::string datatype_name, const std::string fileregistration)
Definition: portManager.hpp:99
InfoPort getPortData(const std::string name)
Definition: portManager.hpp:183
Basic singleton for managing Ports declaration in mimmo.
Definition: portManager.hpp:71
bool containsContainer(const std::string name)
Definition: portManager.hpp:148
bool containsDatatype(const std::string name)
Definition: portManager.hpp:156
bool containsPort(const std::string name)
Definition: portManager.hpp:140
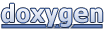