Abstract Interface for selection classes. More...
#include <MeshSelection.hpp>
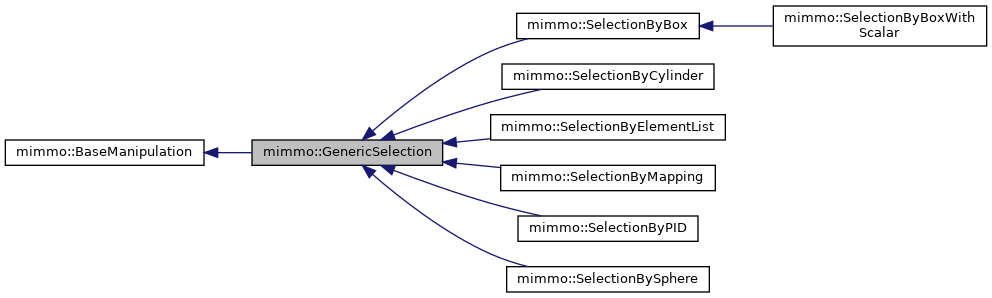
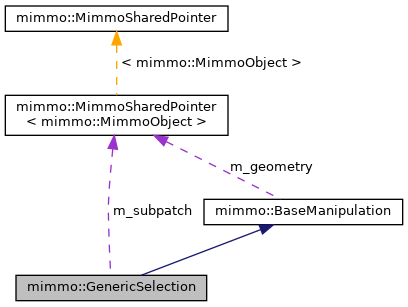
Protected Member Functions | |
virtual livector1D | extractSelection ()=0 |
void | swap (GenericSelection &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_dual |
mimmo::MimmoSharedPointer< MimmoObject > | m_subpatch |
int | m_topo |
SelectionType | m_type |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Abstract Interface for selection classes.
Class/BaseManipulation Object managing selection of sub-patches of MimmoObject Data structure.
Ports available in GenericSelection Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | setDual | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORLI | constrainedBoundary | (MC_VECTOR, MD_LONG) |
M_GEOM | getPatch | (MC_SCALAR, MD_MIMMO_) |
Definition at line 80 of file MeshSelection.hpp.
Constructor & Destructor Documentation
◆ GenericSelection() [1/2]
mimmo::GenericSelection::GenericSelection | ( | ) |
Basic Constructor
Definition at line 32 of file GenericSelection.cpp.
◆ ~GenericSelection()
|
virtual |
Basic Destructor
Definition at line 41 of file GenericSelection.cpp.
◆ GenericSelection() [2/2]
mimmo::GenericSelection::GenericSelection | ( | const GenericSelection & | other | ) |
Copy Constructor, any already calculated selection is not copied.
Definition at line 48 of file GenericSelection.cpp.
Member Function Documentation
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::SelectionByElementList, mimmo::SelectionByBoxWithScalar, mimmo::SelectionByPID, mimmo::SelectionByMapping, mimmo::SelectionBySphere, mimmo::SelectionByCylinder, and mimmo::SelectionByBox.
Definition at line 83 of file GenericSelection.cpp.
◆ constrainedBoundary()
livector1D mimmo::GenericSelection::constrainedBoundary | ( | ) |
Return list of constrained boundary nodes (all those boundary nodes of the subpatch extracted which are not part of the boundary of the mother geometry) MPI version return a list of all subpatch physical border vertices not belonging to the physical border of the mother, ghost included (internal rank border faces are not accounted). This list is computed locally, and vary from rank to rank, according to mesh distribution among ranks.
- Returns
- list of physical boundary vertex ID
Definition at line 176 of file GenericSelection.cpp.
◆ execute()
|
virtual |
Execute your object. A selection is extracted and trasferred in an indipendent MimmoObject structure pointed by m_subpatch member
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::SelectionByBoxWithScalar.
Definition at line 222 of file GenericSelection.cpp.
◆ extractSelection()
|
protectedpure virtual |
Extract selection from target geometry
Implemented in mimmo::SelectionByElementList, mimmo::SelectionByPID, mimmo::SelectionByMapping, mimmo::SelectionBySphere, mimmo::SelectionByCylinder, and mimmo::SelectionByBox.
◆ getPatch()
const mimmo::MimmoSharedPointer< MimmoObject > mimmo::GenericSelection::getPatch | ( | ) | const |
Return pointer by copy to sub-patch extracted by the class
- Returns
- pointer to MimmoObject extracted sub-patch
Return pointer by copy to subpatch extracted by the class [Const overloading].
- Returns
- pointer to MimmoObject extracted sub-patch
Definition at line 111 of file GenericSelection.cpp.
◆ isDual()
bool mimmo::GenericSelection::isDual | ( | ) |
Return actual status of "dual" feature of the class. See setDual method.
- Returns
- true/false for "dual" feature activated or not
Definition at line 161 of file GenericSelection.cpp.
◆ operator=()
GenericSelection & mimmo::GenericSelection::operator= | ( | const GenericSelection & | other | ) |
Copy operator, any already calculated selection is not copied.
Definition at line 57 of file GenericSelection.cpp.
◆ plotOptionalResults()
|
virtual |
Plot optional result of the class in execution. It plots the selected patch as standard vtk unstructured grid.
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SelectionByBoxWithScalar.
Definition at line 282 of file GenericSelection.cpp.
◆ setDual()
void mimmo::GenericSelection::setDual | ( | bool | flag = false | ) |
Set your class behavior selecting a portion of a target geoemetry. Given a initial set up, gets the dual result (its negative) of current selection. For instance, in a class extracting geometry inside the volume of an elemental shape, gets all other parts of it not included in the shape. For a class extracting portions of geometry matching an external tessellation, gets all the other parts not matching it. For a class extracting portion of geometry identified by a PID list, get all other parts not marked by such list.
- Parameters
-
[in] flag Active/Inactive "dual" feature true/false.
- Examples
- utils_example_00003.cpp.
Definition at line 152 of file GenericSelection.cpp.
◆ setGeometry()
|
virtual |
Set link to target geometry for your selection. Reimplementation of mimmo::BaseManipulation::setGeometry();
- Parameters
-
[in] target Pointer to MimmoObject with target geometry.
Reimplemented in mimmo::SelectionByPID, and mimmo::SelectionByMapping.
Definition at line 130 of file GenericSelection.cpp.
◆ swap()
|
protectednoexcept |
Swap function. Assign data of this class to another of the same type and vice-versa. Resulting patch of selection is not swapped, ever.
- Parameters
-
[in] x GenericSelection object
Definition at line 71 of file GenericSelection.cpp.
◆ whichMethod()
SelectionType mimmo::GenericSelection::whichMethod | ( | ) |
Return type of method for selection. See SelectionType enum for available methods.
- Returns
- type of selection method
Definition at line 102 of file GenericSelection.cpp.
Member Data Documentation
◆ m_dual
|
protected |
False selects w/ current set up, true gets its "negative". False is default.
Definition at line 87 of file MeshSelection.hpp.
◆ m_subpatch
|
protected |
Pointer to result sub-patch
Definition at line 85 of file MeshSelection.hpp.
◆ m_topo
|
protected |
1 = surface (default value), 2 = volume, 3 = points cloud, 4 = 3D-Curve
Definition at line 86 of file MeshSelection.hpp.
◆ m_type
|
protected |
Type of enum class SelectionType for selection method
Definition at line 84 of file MeshSelection.hpp.
The documentation for this class was generated from the following files:
- src/geohandlers/MeshSelection.hpp
- src/geohandlers/GenericSelection.cpp
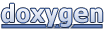