Selection through sphere primitive. More...
#include <MeshSelection.hpp>
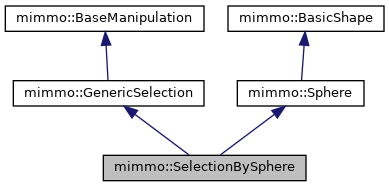

Protected Member Functions | |
livector1D | extractSelection () |
void | swap (SelectionBySphere &) noexcept |
![]() | |
void | swap (GenericSelection &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
![]() | |
darray3E | checkNearestPointToAABBox (const darray3E &point, const darray3E &bMin, const darray3E &bMax) |
uint32_t | intersectShapePlane (int level, const darray3E &target) |
void | swap (BasicShape &) noexcept |
Detailed Description
Selection through sphere primitive.
Selection Object managing selection of sub-patches of a MimmoObject Data structure. Select all elements contained in a sphere.
Ports available in SelectionBySphere Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXES | setRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_SPAN | setSpan | (MC_ARRAY3, MD_FLOAT) |
M_INFLIMITS | setInfLimits | (MC_ARRAY3, MD_FLOAT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
Inherited from GenericSelection
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | setDual | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORLI | constrainedBoundary | (MC_VECTOR, MD_LONG) |
M_GEOM | getPatch | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.SelectionBySphere
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Dual: boolean to get straight what given by selection method or its exact dual;
- Origin: array of 3 doubles identifying origin of sphere (space separated);
- Span: span of the sphere (radius angular_azimuthal_width angular_polar_width);
- RefSystem: reference system of the sphere:
<RefSystem>
<axis0> 1.0 0.0 0.0 </axis0>
<axis1> 0.0 1.0 0.0 </axis1>
<axis2> 0.0 0.0 1.0 </axis2>
</RefSystem>
- InfLimits: set starting point for each spherical coordinate. Useful to assign different starting angular coordinate to azimuthal width/ polar width.
Geometry has to be mandatorily passed through port.
- Examples
- geohandlers_example_00001.cpp.
Definition at line 363 of file MeshSelection.hpp.
Constructor & Destructor Documentation
◆ SelectionBySphere() [1/4]
mimmo::SelectionBySphere::SelectionBySphere | ( | ) |
Basic Constructor
Definition at line 33 of file SelectionBySphere.cpp.
◆ SelectionBySphere() [2/4]
mimmo::SelectionBySphere::SelectionBySphere | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 42 of file SelectionBySphere.cpp.
◆ SelectionBySphere() [3/4]
mimmo::SelectionBySphere::SelectionBySphere | ( | darray3E | origin, |
darray3E | span, | ||
double | infLimTheta, | ||
double | infLimPhi, | ||
mimmo::MimmoSharedPointer< MimmoObject > | target | ||
) |
Custom Constructor. Pay attention span of angular and polar coords are at most 2*pi and pi respectively. Inf limits of polar coordinate must be > 0 and < pi.
- Parameters
-
[in] origin Origin of the sphere->baricenter [in] span Span of the cylinder, main radius/ span of angular coord in radians/span of the polar coord in radians [in] infLimTheta Starting origin of the angular coordinate. default is 0 radians. [in] infLimPhi Starting origin of the polar coordinate. default is 0 radians. [in] target Pointer to a target geometry
Definition at line 66 of file SelectionBySphere.cpp.
◆ ~SelectionBySphere()
|
virtual |
Destructor
Definition at line 79 of file SelectionBySphere.cpp.
◆ SelectionBySphere() [4/4]
mimmo::SelectionBySphere::SelectionBySphere | ( | const SelectionBySphere & | other | ) |
Copy Constructor
Definition at line 84 of file SelectionBySphere.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 156 of file SelectionBySphere.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Reimplemented from mimmo::GenericSelection.
Definition at line 110 of file SelectionBySphere.cpp.
◆ clear()
void mimmo::SelectionBySphere::clear | ( | ) |
Clear content of the class
Definition at line 127 of file SelectionBySphere.cpp.
◆ extractSelection()
|
protectedvirtual |
Extract portion of target geometry which are enough near to the external geometry provided
- Returns
- ids of cell of target tesselation extracted
Implements mimmo::GenericSelection.
Definition at line 137 of file SelectionBySphere.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 258 of file SelectionBySphere.cpp.
◆ operator=()
SelectionBySphere & mimmo::SelectionBySphere::operator= | ( | SelectionBySphere | other | ) |
Copy operator
Definition at line 90 of file SelectionBySphere.cpp.
◆ swap()
|
protectednoexcept |
Swap function. Assign data of this class to another of the same type and vice-versa. Resulting patch of selection is not swapped, ever.
- Parameters
-
[in] x SelectionBySphere object
Definition at line 100 of file SelectionBySphere.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/MeshSelection.hpp
- src/geohandlers/SelectionBySphere.cpp
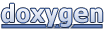