Elementary Shape Representation of a Sphere or portion of it. More...
#include <BasicShapes.hpp>
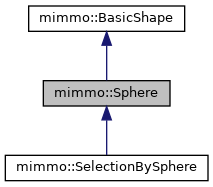
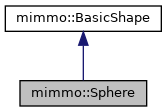
Additional Inherited Members | |
![]() | |
darray3E | checkNearestPointToAABBox (const darray3E &point, const darray3E &bMin, const darray3E &bMax) |
uint32_t | intersectShapePlane (int level, const darray3E &target) |
void | swap (BasicShape &) noexcept |
![]() | |
static dmatrix33E | inverse (const dmatrix33E &mat) |
static darray3E | matmul (const darray3E &vec, const dmatrix33E &mat) |
static darray3E | matmul (const dmatrix33E &mat, const darray3E &vec) |
static dmatrix33E | transpose (const dmatrix33E &mat) |
![]() | |
dvecarr3E | m_bbox |
darray3E | m_infLimits |
darray3E | m_origin |
darray3E | m_scaling |
dmatrix33E | m_sdr |
dmatrix33E | m_sdr_inverse |
ShapeType | m_shape |
darray3E | m_span |
std::array< CoordType, 3 > | m_typeCoord |
Detailed Description
Elementary Shape Representation of a Sphere or portion of it.
Volumetric Core Element, shaped as a sphere, directly derived from BasicShape class.
Definition at line 322 of file BasicShapes.hpp.
Constructor & Destructor Documentation
◆ Sphere() [1/3]
mimmo::Sphere::Sphere | ( | ) |
Basic Constructor
Definition at line 1387 of file BasicShapes.cpp.
◆ Sphere() [2/3]
Custom Constructor. Set shape originand its dimensions, ordered as overall radius, azimuthal/tangential coordinate, polar coordinate.
- Parameters
-
[in] origin point origin in global reference system [in] span characteristic dimension of your sphere/ portion of;
Definition at line 1400 of file BasicShapes.cpp.
◆ ~Sphere()
mimmo::Sphere::~Sphere | ( | ) |
Basic Destructor
Definition at line 1407 of file BasicShapes.cpp.
◆ Sphere() [3/3]
mimmo::Sphere::Sphere | ( | const Sphere & | other | ) |
Copy Constructor
- Parameters
-
[in] other Sphere object where copy from
Definition at line 1413 of file BasicShapes.cpp.
Member Function Documentation
◆ getLocalOrigin()
|
virtual |
- Returns
- local origin of your primitive shape
Implements mimmo::BasicShape.
Definition at line 1493 of file BasicShapes.cpp.
◆ intersectShapeAABBox()
Check if current shape intersects or is totally contained into the given Axis Aligned Bounding Box
- Parameters
-
[in] bMin inferior extremal point of the AABB [in] bMax superior extremal point of the AABB
- Returns
- true if intersects, false otherwise
Implements mimmo::BasicShape.
Definition at line 1635 of file BasicShapes.cpp.
◆ toLocalCoord()
Transform point from world coordinate system, to local reference system of the shape.
- Parameters
-
[in] point target
- Returns
- transformed point
Implements mimmo::BasicShape.
Definition at line 1450 of file BasicShapes.cpp.
◆ toWorldCoord()
Transform point from local reference system of the shape, to world reference system.
- Parameters
-
[in] point target
- Returns
- transformed point
Implements mimmo::BasicShape.
Definition at line 1421 of file BasicShapes.cpp.
The documentation for this class was generated from the following files:
- src/core/BasicShapes.hpp
- src/core/BasicShapes.cpp
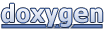