Abstract Interface class for Elementary Shape Representation. More...
#include <BasicShapes.hpp>
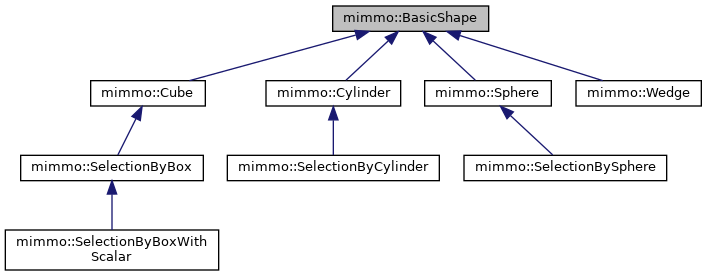
Protected Member Functions | |
darray3E | checkNearestPointToAABBox (const darray3E &point, const darray3E &bMin, const darray3E &bMax) |
uint32_t | intersectShapePlane (int level, const darray3E &target) |
void | swap (BasicShape &) noexcept |
Static Protected Member Functions | |
static dmatrix33E | inverse (const dmatrix33E &mat) |
static darray3E | matmul (const darray3E &vec, const dmatrix33E &mat) |
static darray3E | matmul (const dmatrix33E &mat, const darray3E &vec) |
static dmatrix33E | transpose (const dmatrix33E &mat) |
Protected Attributes | |
dvecarr3E | m_bbox |
darray3E | m_infLimits |
darray3E | m_origin |
darray3E | m_scaling |
dmatrix33E | m_sdr |
dmatrix33E | m_sdr_inverse |
ShapeType | m_shape |
darray3E | m_span |
std::array< CoordType, 3 > | m_typeCoord |
Detailed Description
Abstract Interface class for Elementary Shape Representation.
Interface class for Volumetric Core Element, suitable for interaction with Data Structure stored in a MimmoObject class. Object orientation in 3D space can be externally manipulated with dedicated transformation blocks. Class internally implements transformation to/from local sdr to/from world sdr, that can be used in derived objects from it.
Class works with three reference systems:
- Global Absolute SDR: is the external World reference system
- Local Relative SDR: is the local reference system, not affected by Rigid Transformations as RotoTranslations or Scalings
- basic SDR: local system remapping to unitary cube, not accounting of the shape type.
Definition at line 91 of file BasicShapes.hpp.
Constructor & Destructor Documentation
◆ BasicShape()
mimmo::BasicShape::BasicShape | ( | ) |
Basic Constructor
Definition at line 91 of file BasicShapes.cpp.
◆ ~BasicShape()
|
virtual |
Basic Destructor
Definition at line 105 of file BasicShapes.cpp.
Member Function Documentation
◆ checkNearestPointToAABBox()
|
protected |
- Returns
- the nearest point belonging to an Axis Aligned Bounding Box, given a target vertex. If the target is internal to or on surface of the AABB, return the target itself.
- Parameters
-
[in] point target vertex [in] bMin inferior extremal point of the AABB [in] bMax superior extremal point of the AABB
Definition at line 666 of file BasicShapes.cpp.
◆ excludeCloudPoints() [1/3]
livector1D mimmo::BasicShape::excludeCloudPoints | ( | bitpit::PatchKernel * | tri | ) |
Given a bitpit class bitpit::PatchKernel point cloud, return identifiers of those points outside the volume of the BasicShape object.The method force a one-by-one vertex search.
- Parameters
-
[in] tri pointer to bitpit::PatchKernel object retaining the cloud point
- Returns
- list-by-ids of vertices outside the volumetric patch
Definition at line 525 of file BasicShapes.cpp.
◆ excludeCloudPoints() [2/3]
livector1D mimmo::BasicShape::excludeCloudPoints | ( | const dvecarr3E & | list | ) |
Given a list of vertices of a point cloud, return indices of those vertices outside the volume of BasicShape object
- Parameters
-
[in] list list of cloud points
- Returns
- list-by-indices of vertices outside the volumetric patch
Definition at line 479 of file BasicShapes.cpp.
◆ excludeCloudPoints() [3/3]
livector1D mimmo::BasicShape::excludeCloudPoints | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Given a geometry by MimmoObject class, return vertex identifiers of those vertices outside the volume of the BasicShape object. The methods implicitly use search algorithms based on the bitpit::KdTree of the class MimmoObject.
- Parameters
-
[in] geo target geometry
- Returns
- list-by-ids of vertices outside the volumetric patch
Definition at line 572 of file BasicShapes.cpp.
◆ excludeGeometry() [1/2]
livector1D mimmo::BasicShape::excludeGeometry | ( | bitpit::PatchKernel * | tri | ) |
Given a bitpit class bitpit::PatchKernel tessellation, return cell identifiers of those simplex outside the volume of the BasicShape object.The method searches simplex one-by-one on the whole tesselation.
- Parameters
-
[in] tri target tesselation
- Returns
- list-by-ids of simplicies outside the volumetric patch
Definition at line 432 of file BasicShapes.cpp.
◆ excludeGeometry() [2/2]
livector1D mimmo::BasicShape::excludeGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Given a geometry by MimmoObject class, return cell identifiers of those simplex outside the volume of the BasicShape object. The methods implicitly use search algorithms based on the SkdTree of the class MimmoObject. Ghost elements are considered.
- Parameters
-
[in] geo target tesselation
- Returns
- list-by-ids of simplicies outside the volumetric patch
Definition at line 376 of file BasicShapes.cpp.
◆ getCoordinateType()
CoordType mimmo::BasicShape::getCoordinateType | ( | int | dir | ) |
- Returns
- type of your current shape coordinate "dir". See CoordType enum
- Parameters
-
[in] dir 0,1,2 int flag identifying coordinate
Definition at line 317 of file BasicShapes.cpp.
◆ getInfLimits()
darray3E mimmo::BasicShape::getInfLimits | ( | ) |
- Returns
- current inferior limits of your shape, in local coord reference system
Definition at line 302 of file BasicShapes.cpp.
◆ getLocalOrigin()
|
pure virtual |
Pure virtual method to get local Coordinate inferior limits of primitive shape
- Returns
- local origin
Implemented in mimmo::Wedge, mimmo::Sphere, mimmo::Cylinder, and mimmo::Cube.
◆ getLocalSpan()
darray3E mimmo::BasicShape::getLocalSpan | ( | ) |
- Returns
- span of your elementary shape, in local coord system
Definition at line 344 of file BasicShapes.cpp.
◆ getOrigin()
darray3E mimmo::BasicShape::getOrigin | ( | ) |
- Returns
- current origin of your shape
Definition at line 282 of file BasicShapes.cpp.
◆ getRefSystem()
dmatrix33E mimmo::BasicShape::getRefSystem | ( | ) |
- Returns
- actual axis of global relative sdr
Definition at line 309 of file BasicShapes.cpp.
◆ getScaling()
darray3E mimmo::BasicShape::getScaling | ( | ) |
- Returns
- current scaling w.r.t the local sdr elemental shape
Definition at line 337 of file BasicShapes.cpp.
◆ getShapeType()
ShapeType mimmo::BasicShape::getShapeType | ( | ) |
- Returns
- current type of shape instantiated
- current type of shape instantiated. Const method overloading
Definition at line 323 of file BasicShapes.cpp.
◆ getSpan()
darray3E mimmo::BasicShape::getSpan | ( | ) |
- Returns
- current span of your shape
Definition at line 290 of file BasicShapes.cpp.
◆ includeCloudPoints() [1/3]
livector1D mimmo::BasicShape::includeCloudPoints | ( | bitpit::PatchKernel * | tri | ) |
Given a bitpit class bitpit::PatchKernel point cloud, return identifiers of those points inside the volume of the BasicShape object. The method force a one-by-one vertex search.
- Parameters
-
[in] tri pointer to bitpit::PatchKernel object retaining the cloud point
- Returns
- list-by-ids of vertices included in the volumetric patch
Definition at line 502 of file BasicShapes.cpp.
◆ includeCloudPoints() [2/3]
livector1D mimmo::BasicShape::includeCloudPoints | ( | const dvecarr3E & | list | ) |
Given a list of vertices of a point cloud, return indices of those vertices included into the volume of the object. The method searches vertex one-by-one on the whole point cloud.
- Parameters
-
[in] list list of cloud points
- Returns
- list-by-indices of vertices included in the volumetric patch
Definition at line 456 of file BasicShapes.cpp.
◆ includeCloudPoints() [3/3]
livector1D mimmo::BasicShape::includeCloudPoints | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Given a geometry by MimmoObject class, return vertex identifiers of those vertices inside the volume of the BasicShape object. The methods implicitly use search algorithms based on the bitpit::KdTree of the class MimmoObject. Ghost vertices are included.
- Parameters
-
[in] geo target geometry
- Returns
- list-by-ids of vertices included in the volumetric patch
Definition at line 551 of file BasicShapes.cpp.
◆ includeGeometry() [1/2]
livector1D mimmo::BasicShape::includeGeometry | ( | bitpit::PatchKernel * | tri | ) |
Given a bitpit class bitpit::PatchKernel tessellation, return cell identifiers of those simplex inside the volume of the BasicShape object. The method searches simplex one-by-one on the whole tesselation.
- Parameters
-
[in] tri target tessellation
- Returns
- list-by-ids of simplicies included in the volumetric patch
Definition at line 408 of file BasicShapes.cpp.
◆ includeGeometry() [2/2]
livector1D mimmo::BasicShape::includeGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Given a geometry by MimmoObject class, return cell identifiers of those simplex inside the volume of the BasicShape object. The methods implicitly use search algorithms based on the SkdTree of the class MimmoObject.
- Parameters
-
[in] geo target tessellation
- Returns
- list-by-ids of simplicies included in the volumetric patch
Definition at line 355 of file BasicShapes.cpp.
◆ intersectShapeAABBox()
|
pure virtual |
Pure virtual method to get if the current shape an a given Axis Aligned Bounding Box intersects
- Parameters
-
[in] bMin min point of AABB [in] bMax max point of AABB
Implemented in mimmo::Wedge, mimmo::Sphere, mimmo::Cylinder, and mimmo::Cube.
◆ intersectShapePlane()
|
protected |
Given a 3D point, Check if the Axis Aligned Bounding box of your current shape intersects a given fundamental plane, x=a, y=b, z=c passing from such point.
Return unsigned integer 0,1,2 with the following meaning:
- 0 : no intersection , shape on the left/bottom/before the plane
- 1 : no intersection , shape on the right/top/after the plane
- 2 : intersection occurs
- Parameters
-
[in] level current level of kdTree. 0-> xplane, 1-> yplane, 2->zplane, no other value are allowed. [in] target 3D point
- Returns
- unsigned integer flag between 0 and 2
Definition at line 798 of file BasicShapes.cpp.
◆ inverse()
|
staticprotected |
Invert a 3x3 double matrix
- Parameters
-
[in] mat input matrix
- Returns
- new transposed matrix
Definition at line 829 of file BasicShapes.cpp.
◆ isPointIncluded() [1/2]
bool mimmo::BasicShape::isPointIncluded | ( | bitpit::PatchKernel * | tri, |
const long int & | indexV | ||
) |
- Returns
- true if the given point is included in the volume of the patch
- Parameters
-
[in] tri pointer to a bitpit::Patch tesselation / point cloud [in] indexV id of a vertex belonging to tri;
Definition at line 652 of file BasicShapes.cpp.
◆ isPointIncluded() [2/2]
bool mimmo::BasicShape::isPointIncluded | ( | const darray3E & | point | ) |
- Returns
- true if the given point is included in the volume of the patch
- Parameters
-
[in] point given vertex
Definition at line 634 of file BasicShapes.cpp.
◆ isSimplexIncluded() [1/2]
bool mimmo::BasicShape::isSimplexIncluded | ( | bitpit::PatchKernel * | tri, |
const long int & | indexT | ||
) |
- Returns
- true if if all vertices of a given simplex are included in the volume of the shape
- Parameters
-
[in] tri pointer to a Class_SurfTri tesselation [in] indexT triangle index of tri.
Definition at line 618 of file BasicShapes.cpp.
◆ isSimplexIncluded() [2/2]
bool mimmo::BasicShape::isSimplexIncluded | ( | const dvecarr3E & | simplexVert | ) |
- Returns
- true if all vertices of a given simplex are included in the volume of the shape
- Parameters
-
[in] simplexVert 3 vertices of the given Triangle
Definition at line 604 of file BasicShapes.cpp.
◆ matmul() [1/2]
|
staticprotected |
Matrix M-vector V multiplication V*M (V row dot M columns).
- Parameters
-
[in] vec vector V with 3 elements [in] mat matrix M 3x3 square
- Returns
- 3 element vector result of multiplication
Definition at line 856 of file BasicShapes.cpp.
◆ matmul() [2/2]
|
staticprotected |
Matrix M-vector V multiplication M*V (M rows dot V column).
- Parameters
-
[in] vec vector V with 3 elements [in] mat matrix M 3x3 square
- Returns
- 3 element vector result of multiplication
Definition at line 875 of file BasicShapes.cpp.
◆ setCoordinateType()
void mimmo::BasicShape::setCoordinateType | ( | CoordType | type, |
int | dir | ||
) |
Set type to treat your shape coordinates.
- Parameters
-
[in] type CoordType enum. [in] dir 0,1,2 int flag identifying coordinate
Definition at line 275 of file BasicShapes.cpp.
◆ setInfLimits() [1/2]
void mimmo::BasicShape::setInfLimits | ( | darray3E | val | ) |
Set inferior limits your shape, in its local reference system. The method is useful when drawing part of your original shape, as in the case of cylinders or sphere portions, by manipulating the adimensional curvilinear coordinate.
- Parameters
-
[in] val lower value origin for all three coordinates
Definition at line 182 of file BasicShapes.cpp.
◆ setInfLimits() [2/2]
void mimmo::BasicShape::setInfLimits | ( | double | orig, |
int | dir | ||
) |
Set inferior limits of your shape, in its local reference system. The method is useful when drawing part of your original shape, as in the case of cylinders or sphere portions, by manipulating the adimensional curvilinear coordinate.
- Parameters
-
[in] orig first coordinate origin [in] dir 0,1,2 int flag identifying coordinate
- Examples
- geohandlers_example_00000.cpp.
Definition at line 163 of file BasicShapes.cpp.
◆ setOrigin()
void mimmo::BasicShape::setOrigin | ( | darray3E | origin | ) |
Set origin of your shape. The origin is meant as the baricenter of your shape in absolute reference system.
- Parameters
-
[in] origin new origin point
Definition at line 130 of file BasicShapes.cpp.
◆ setRefSystem() [1/3]
Set new axis orientation of the local reference system
- Parameters
-
[in] axis0 first axis [in] axis1 second axis [in] axis2 third axis
if chosen axes are not orthogonal, doing nothing
- Examples
- geohandlers_example_00000.cpp.
Definition at line 196 of file BasicShapes.cpp.
◆ setRefSystem() [2/3]
void mimmo::BasicShape::setRefSystem | ( | dmatrix33E | axes | ) |
Set new axes orientation of the local reference system
- Parameters
-
[in] axes new direction of local axes.
Definition at line 266 of file BasicShapes.cpp.
◆ setRefSystem() [3/3]
void mimmo::BasicShape::setRefSystem | ( | int | label, |
darray3E | axis | ||
) |
Set new axis orientation of the local reference system
- Parameters
-
[in] label 0,1,2 identify local x,y,z axis of the primitive shape [in] axis new direction of selected local axis.
Definition at line 232 of file BasicShapes.cpp.
◆ setSpan() [1/2]
void mimmo::BasicShape::setSpan | ( | darray3E | span | ) |
Set span of your shape, according to its local reference system
- Parameters
-
[in] span coordinate span
Definition at line 149 of file BasicShapes.cpp.
◆ setSpan() [2/2]
void mimmo::BasicShape::setSpan | ( | double | s0, |
double | s1, | ||
double | s2 | ||
) |
Set span of your shape, according to its local reference system
- Parameters
-
[in] s0 first coordinate span [in] s1 second coordinate span [in] s2 third coordinate span
Definition at line 140 of file BasicShapes.cpp.
◆ swap()
|
protectednoexcept |
Swap method, assign data of the current class to an external one of the same type and vice-versa
- Parameters
-
[in] x BasicShape object to be swapped;
Definition at line 113 of file BasicShapes.cpp.
◆ toLocalCoord()
Pure virtual method to convert from World to Local Coordinates
- Parameters
-
[in] point 3D point in world global coordinates
- Returns
- point in local world coordinate
Implemented in mimmo::Wedge, mimmo::Sphere, mimmo::Cylinder, and mimmo::Cube.
◆ toWorldCoord()
Pure virtual method to convert from Local to World Coordinates
- Parameters
-
[in] point 3D point in local shape coordinates
- Returns
- point in world global coordinate
Implemented in mimmo::Wedge, mimmo::Sphere, mimmo::Cylinder, and mimmo::Cube.
◆ transpose()
|
staticprotected |
Transpose a 3x3 double matrix
- Parameters
-
[in] mat input matrix
- Returns
- new transposed matrix
Definition at line 812 of file BasicShapes.cpp.
Member Data Documentation
◆ m_bbox
|
protected |
points of bounding box of the shape
Definition at line 104 of file BasicShapes.hpp.
◆ m_infLimits
|
protected |
inferior limits of coordinate of your current shape
Definition at line 97 of file BasicShapes.hpp.
◆ m_origin
|
protected |
origin of your shape. May change according to the shape type
Definition at line 95 of file BasicShapes.hpp.
◆ m_scaling
|
protected |
scaling vector of dimensional coordinates
Definition at line 100 of file BasicShapes.hpp.
◆ m_sdr
|
protected |
axis position of the local reference system w.r.t absolute one
Definition at line 98 of file BasicShapes.hpp.
◆ m_sdr_inverse
|
protected |
inverse rotating sdr
Definition at line 99 of file BasicShapes.hpp.
◆ m_shape
|
protected |
shape identifier, see BasicShape::ShapeType enum
Definition at line 94 of file BasicShapes.hpp.
◆ m_span
|
protected |
coordinate span of your current shape, in its local reference system
Definition at line 96 of file BasicShapes.hpp.
◆ m_typeCoord
|
protected |
identifiers for coordinate type definition.DEFAULT is clamped
Definition at line 102 of file BasicShapes.hpp.
The documentation for this class was generated from the following files:
- src/core/BasicShapes.hpp
- src/core/BasicShapes.cpp
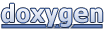