BasicShapes.hpp
187 darray3E checkNearestPointToAABBox(const darray3E &point, const darray3E &bMin, const darray3E &bMax);
235 void searchKdTreeMatches(bitpit::KdTree<3,bitpit::Vertex,long> & tree, livector1D & result, bool squeeze = true);
236 void searchBvTreeMatches(bitpit::PatchSkdTree & tree, bitpit::PatchKernel * geo, livector1D & result, bool squeeze = true);
livector1D includeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:355
Abstract Interface class for Elementary Shape Representation.
Definition: BasicShapes.hpp:91
@ CUBE
void setInfLimits(double val, int dir)
Definition: BasicShapes.cpp:163
bool intersectShapeAABBox(const darray3E &bMin, const darray3E &bMax)
Definition: BasicShapes.cpp:1875
static dmatrix33E inverse(const dmatrix33E &mat)
Definition: BasicShapes.cpp:829
virtual darray3E toWorldCoord(const darray3E &point)=0
CoordType
Specify type of conditions to distribute NURBS nodes in a given coordinate of the shape.
Definition: BasicShapes.hpp:49
bool intersectShapeAABBox(const darray3E &bMin, const darray3E &bMax)
Definition: BasicShapes.cpp:1635
Elementary Shape Representation of a Prism with triangular basis.
Definition: BasicShapes.hpp:357
bool isSimplexIncluded(const dvecarr3E &)
Definition: BasicShapes.cpp:604
mimmo custom derivation of bitpit OBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:55
virtual bool intersectShapeAABBox(const darray3E &bMin, const darray3E &bMax)=0
bool intersectShapeAABBox(const darray3E &bMin, const darray3E &bMax)
Definition: BasicShapes.cpp:1352
mimmo::IBinaryStream & operator>>(mimmo::IBinaryStream &buf, mimmo::CoordType &element)
Definition: BasicShapes.cpp:77
Elementary Shape Representation of a Sphere or portion of it.
Definition: BasicShapes.hpp:322
darray3E toLocalCoord(const darray3E &point)
Definition: BasicShapes.cpp:1185
virtual darray3E toLocalCoord(const darray3E &point)=0
darray3E toWorldCoord(const darray3E &point)
Definition: BasicShapes.cpp:928
Elementary Shape Representation of a Cylinder or portion of it.
Definition: BasicShapes.hpp:288
darray3E toWorldCoord(const darray3E &point)
Definition: BasicShapes.cpp:1421
static darray3E matmul(const darray3E &vec, const dmatrix33E &mat)
Definition: BasicShapes.cpp:856
static dmatrix33E transpose(const dmatrix33E &mat)
Definition: BasicShapes.cpp:812
livector1D includeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:456
darray3E checkNearestPointToAABBox(const darray3E &point, const darray3E &bMin, const darray3E &bMax)
Definition: BasicShapes.cpp:666
mimmo::OBinaryStream & operator<<(mimmo::OBinaryStream &buf, const mimmo::CoordType &element)
Definition: BasicShapes.cpp:64
darray3E toLocalCoord(const darray3E &point)
Definition: BasicShapes.cpp:1736
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicShapes.cpp:196
bool isPointIncluded(const darray3E &)
Definition: BasicShapes.cpp:634
uint32_t intersectShapePlane(int level, const darray3E &target)
Definition: BasicShapes.cpp:798
bool intersectShapeAABBox(const darray3E &bMin, const darray3E &bMax)
Definition: BasicShapes.cpp:1085
ShapeType
Identifies the type of elemental shape supported by BasicShape class.
Definition: BasicShapes.hpp:38
darray3E toWorldCoord(const darray3E &point)
Definition: BasicShapes.cpp:1157
livector1D excludeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:376
@ UNCLAMPED
@ WEDGE
@ SPHERE
mimmo custom derivation of bitpit IBinaryStream (see relative doc)
Definition: mimmo_binary_stream.hpp:41
@ CLAMPED
livector1D excludeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:479
virtual darray3E getLocalOrigin()=0
void setCoordinateType(CoordType, int dir)
Definition: BasicShapes.cpp:275
darray3E toLocalCoord(const darray3E &point)
Definition: BasicShapes.cpp:1450
darray3E toWorldCoord(const darray3E &point)
Definition: BasicShapes.cpp:1705
@ PERIODIC
@ CYLINDER
CoordType getCoordinateType(int dir)
Definition: BasicShapes.cpp:317
darray3E toLocalCoord(const darray3E &point)
Definition: BasicShapes.cpp:955
@ SYMMETRIC
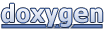