SelectionBySphere.cpp
66 SelectionBySphere::SelectionBySphere(darray3E origin, darray3E span, double infLimTheta, double infLimPhi, mimmo::MimmoSharedPointer<MimmoObject> target){
84 SelectionBySphere::SelectionBySphere(const SelectionBySphere & other):GenericSelection(other), Sphere(other){
116 built = (built && createPortIn<darray3E, SelectionBySphere>(this, &SelectionBySphere::setOrigin,M_POINT));
117 built = (built && createPortIn<darray3E, SelectionBySphere>(this, &SelectionBySphere::setSpan, M_SPAN));
118 built = (built && createPortIn<dmatrix33E, SelectionBySphere>(this, &SelectionBySphere::setRefSystem, M_AXES));
119 built = (built && createPortIn<darray3E, SelectionBySphere>(this, &SelectionBySphere::setInfLimits, M_INFLIMITS));
livector1D includeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:355
void setInfLimits(double val, int dir)
Definition: BasicShapes.cpp:163
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
Elementary Shape Representation of a Sphere or portion of it.
Definition: BasicShapes.hpp:322
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
livector1D extractSelection()
Definition: SelectionBySphere.cpp:137
virtual ~SelectionBySphere()
Definition: SelectionBySphere.cpp:79
livector1D includeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:456
@ SPHERE
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicShapes.cpp:196
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
livector1D excludeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:376
SelectionBySphere & operator=(SelectionBySphere other)
Definition: SelectionBySphere.cpp:90
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionBySphere.cpp:258
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionBySphere.cpp:156
virtual void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: GenericSelection.cpp:130
livector1D excludeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:479
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(SelectionBySphere &) noexcept
Definition: SelectionBySphere.cpp:100
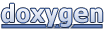