GenericSelection.cpp
87 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, GenericSelection>(this, &GenericSelection::setGeometry, M_GEOM, true));
88 built = (built && createPortIn<bool, GenericSelection>(this, &GenericSelection::setDual,M_VALUEB));
90 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, GenericSelection>(this, &GenericSelection::getPatch,M_GEOM));
91 built = (built && createPortOut<livector1D, GenericSelection>(this, &GenericSelection::constrainedBoundary, M_VECTORLI));
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
@ UNDEFINED
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
SelectionType
Enum class for choiche of method to select sub-patch of a MimmoObject tessellated mesh.
Definition: MeshSelection.hpp:43
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual ~GenericSelection()
Definition: GenericSelection.cpp:41
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
const mimmo::MimmoSharedPointer< MimmoObject > getPatch() const
Definition: GenericSelection.cpp:111
virtual void plotOptionalResults()
Definition: GenericSelection.cpp:282
virtual void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: GenericSelection.cpp:130
GenericSelection & operator=(const GenericSelection &other)
Definition: GenericSelection.cpp:57
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
virtual livector1D extractSelection()=0
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
livector1D constrainedBoundary()
Definition: GenericSelection.cpp:176
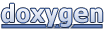