SelectionByPID.cpp
59 SelectionByPID::SelectionByPID(livector1D & pidlist, mimmo::MimmoSharedPointer<MimmoObject> target){
108 built = (built && createPortIn<long, SelectionByPID>(this, &SelectionByPID::setPID, M_VALUELI));
109 built = (built && createPortIn<std::vector<long>, SelectionByPID>(this, &SelectionByPID::setPID, M_VECTORLI2));
livector1D extractSelection()
Definition: SelectionByPID.cpp:229
SelectionByPID & operator=(SelectionByPID other)
Definition: SelectionByPID.cpp:81
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByPID.cpp:306
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
livector1D getActivePID(bool active=true)
Definition: SelectionByPID.cpp:135
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: SelectionByPID.cpp:149
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByPID.cpp:357
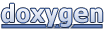