SelectionByBox.cpp
62 SelectionByBox::SelectionByBox(darray3E origin, darray3E span, mimmo::MimmoSharedPointer<MimmoObject> target){
78 SelectionByBox::SelectionByBox(const SelectionByBox & other):GenericSelection(other), Cube(other){
109 built = (built && createPortIn<darray3E, SelectionByBox>(this, &SelectionByBox::setOrigin, M_POINT ));
110 built = (built && createPortIn<darray3E, SelectionByBox>(this, &SelectionByBox::setSpan, M_SPAN));
111 built = (built && createPortIn<dmatrix33E, SelectionByBox>(this, &SelectionByBox::setRefSystem, M_AXES));
livector1D includeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:355
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBox.cpp:241
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
livector1D includeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:456
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicShapes.cpp:196
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByBox.cpp:153
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
livector1D excludeGeometry(MimmoSharedPointer< MimmoObject >)
Definition: BasicShapes.cpp:376
SelectionByBox & operator=(SelectionByBox other)
Definition: SelectionByBox.cpp:84
virtual void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: GenericSelection.cpp:130
livector1D excludeCloudPoints(const dvecarr3E &)
Definition: BasicShapes.cpp:479
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
livector1D extractSelection()
Definition: SelectionByBox.cpp:133
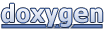