SelectionByMapping.cpp
126 SelectionByMapping::SelectionByMapping(std::unordered_map<std::string, int> & geolist, mimmo::MimmoSharedPointer<MimmoObject> target, double tolerance){
171 SelectionByMapping::SelectionByMapping(const SelectionByMapping & other):GenericSelection(other){
213 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, SelectionByMapping>(this, &SelectionByMapping::addMappingGeometry,M_GEOM2));
412 (*m_log)<< m_name << " failed to read or unsuitable geometry in SelectionByMapping::getProximity"<<std::endl;
418 livector1D result = mimmo::skdTreeUtils::selectByGlobalPatch(geo->getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tolerance);
420 livector1D result = mimmo::skdTreeUtils::selectByPatch(geo->getGeometry()->getSkdTree(), getGeometry()->getSkdTree(), m_tolerance);
440 livector1D result = mimmo::skdTreeUtils::selectByGlobalPatch(obj->getSkdTree(), getGeometry()->getSkdTree(), m_tolerance);
442 livector1D result = mimmo::skdTreeUtils::selectByPatch(obj->getSkdTree(), getGeometry()->getSkdTree(), m_tolerance);
480 SelectionByMapping::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
void setTolerance(double tol=1.e-8)
Definition: SelectionByMapping.cpp:233
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject > geometry)
Definition: SelectionByMapping.cpp:246
void addFile(std::pair< std::string, int >)
Definition: SelectionByMapping.cpp:291
@ MAPPING
void removeFile(std::string)
Definition: SelectionByMapping.cpp:314
void swap(SelectionByMapping &) noexcept
Definition: SelectionByMapping.cpp:192
const std::unordered_map< std::string, int > & getFiles() const
Definition: SelectionByMapping.cpp:271
void setBuildSkdTree(bool build)
Definition: MimmoGeometry.cpp:466
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByMapping.cpp:480
mimmo::MimmoSharedPointer< MimmoObject > m_subpatch
Definition: MeshSelection.hpp:85
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
Selection mapping external surfaces/volume/3D curves on a target mesh of the same topology.
Definition: MeshSelection.hpp:458
SelectionByMapping(int topo=1)
Definition: SelectionByMapping.cpp:36
livector1D extractSelection()
Definition: SelectionByMapping.cpp:350
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectionByMapping.cpp:561
SelectionByMapping & operator=(SelectionByMapping other)
Definition: SelectionByMapping.cpp:182
void setFiles(std::unordered_map< std::string, int >)
Definition: SelectionByMapping.cpp:280
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void addMappingGeometry(mimmo::MimmoSharedPointer< MimmoObject > obj)
Definition: SelectionByMapping.cpp:303
std::vector< long > selectByPatch(bitpit::PatchSkdTree *selection, bitpit::PatchSkdTree *target, double tol)
Definition: SkdTreeUtils.cpp:251
void swap(GenericSelection &x) noexcept
Definition: GenericSelection.cpp:71
void setFilename(std::string filename)
Definition: MimmoGeometry.cpp:324
virtual ~SelectionByMapping()
Definition: SelectionByMapping.cpp:166
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void removeMappingGeometries()
Definition: SelectionByMapping.cpp:330
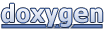