MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Object, handling geometry. More...
#include <MimmoGeometry.hpp>
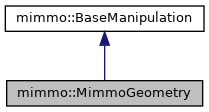
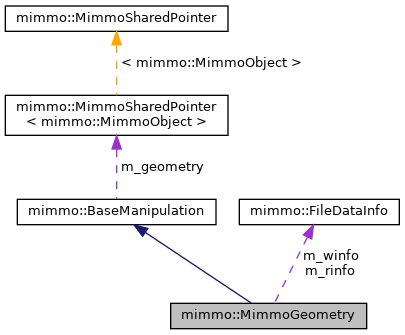
Public Types | |
enum | IOMode { IOMode::READ = 0, IOMode::WRITE = 1, IOMode::CONVERT = 2 } |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
Public Member Functions | |
MimmoGeometry (const bitpit::Config::Section &rootXML) | |
MimmoGeometry (const MimmoGeometry &other) | |
MimmoGeometry (IOMode mode=IOMode::READ) | |
virtual | ~MimmoGeometry () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clear () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
bitpit::PiercedVector< bitpit::Cell > * | getCells () |
const MimmoGeometry * | getCopy () |
bitpit::PiercedVector< bitpit::Vertex > * | getVertices () |
bool | isEmpty () |
MimmoGeometry & | operator= (MimmoGeometry other) |
bool | read () |
void | setBuildKdTree (bool build) |
void | setBuildSkdTree (bool build) |
void | setClean (bool clean=true) |
void | setCodex (bool binary=true) |
void | setDir (std::string dir) |
void | setFilename (std::string filename) |
void | setFileType (FileType type) |
void | setFileType (int type) |
void | setFormatNAS (WFORMAT wform) |
void | setGeometry (int type=1) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setMultiSolidSTL (bool multi=true) |
void | setParallelRestore (bool parallelRestore=0) |
void | setPID (livector1D pids) |
void | setPID (std::unordered_map< long, long > pidsMap) |
void | setReadDir (std::string dir) |
void | setReadFilename (std::string filename) |
void | setReadFileType (FileType type) |
void | setReadFileType (int type) |
void | setReferencePID (long pid) |
void | setTolerance (double tol) |
void | setWriteDir (std::string dir) |
void | setWriteFilename (std::string filename) |
void | setWriteFileType (FileType type) |
void | setWriteFileType (int type) |
bool | write () |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | _setRead (bool read=true) |
void | _setWrite (bool write=true) |
bool | fileExist (const std::string &filename) |
void | setDefaults () |
void | setIOMode (int mode) |
void | setIOMode (IOMode mode) |
void | swap (MimmoGeometry &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_buildKdTree |
bool | m_buildSkdTree |
bool | m_clean |
bool | m_codex |
bool | m_multiSolidSTL |
bool | m_parallelRestore |
bool | m_read |
long | m_refPID |
FileDataInfo | m_rinfo |
double | m_tolerance |
WFORMAT | m_wformat |
FileDataInfo | m_winfo |
bool | m_write |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Object, handling geometry.
MimmoGeometry is the object to manage the import/export/substantial modifications of geometries. It returns a standard MimmoObject class as product of its execution; The mesh to import/export/convert has to be a mesh with constant type elements. The valid format are: binary .stl, ascii .vtu (triangle/quadrilateral elements) and ascii .nas (triangle elements) for surface mesh; ascii .vtu (tetra/hexa elements) for volume mesh.
On distributed archs, MimmoGeometry can write and read in parallel in VTU formats and in mimmo dumping format. In case of VTU formats the collective file extension .pvtu is used for the input/output filename. When reading, the number of processors used to write the input file had to be equal to the current number of processors used to read. If MPI support is enabled but the number of processors is 1, MimmoGeometry reads/writes VTU file as serial one, by postponing the usual file extension .vtu. Nastran files are always written in serial mode, i.e. only the master rank 0 writes on file its partition. In order to write a distributed mesh in a Nastran format, the user has to serialize it before to pass the MimmoObject to the MimmoGeometry block.
When MPI is enabled a read geometry in mimmo dumping can be distributed among the processes (parallel) or repeated serially among the processes (not parallel). The different behavior can be controlled by a set method (setParallelRestore). Note that the parallel/not parallel property set on block has to be coherent with the restored geometry, i.e. it has to be known a-priori by the user. Default value of the feature is true (parallel) in case of MPI enabled when reading a mimmo dumping format geometry.
It can be used in three modes reader/writer/converter. To set the mode it uses an enum IOMode list:
- READ = 0
- WRITE = 1
- CONVERT = 2
It uses smart enums FileType list of available geometry formats, which are:
- STL = 0 Ascii/Binary triangulation stl.
- SURFVTU = 1 Surface mesh vtu, of any 2D bitpit::ElementType.
- VOLVTU = 2 Volume mesh VTU, of any 3D bitpit::ElementType.
- NAS = 3 Nastran surface triangular/quad surface meshes.
- PCVTU = 4 Point Cloud VTU, of only VERTEX elements
- CURVEVTU= 5 3D Curve in VTU, of only LINE elements
- MIMMO = 99 mimmo dump/restore format *.geomimmo
Outside this list of options, the class cannot hold any other type of formats for now. The smart enum can be recalled in every moment in your code, just using mimmo::FileType
and including MimmoGeometry header.
Ports available in MimmoGeometry Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | m_geometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.Geometry
; - Priority: uint marking priority in multi-chain execution;
- IOMode: activate READ, WRITE or CONVERT mode;
- Dir: directory path;
- Filename: name of file for reading/writing;
- FileType: file type identifier;
- ReadFileType: file type identifier for reader (to be used in case of converter mode);
- WriteFileType: file type identifier for writer (to be used in case of converter mode);
- WriteMultiSolidSTL: 0-false/1-true, if WriteFileType is STL, MultiSolid STL writing can be activated or not
- ReadDir: directory path (to be used in case of converter mode and different paths);
- ReadFilename: name of file for reading/writing (to be used in case of converter mode and different filenames);
- WriteDir: directory path (to be used in case of converter mode and different paths);
- WriteFilename: name of file for reading/writing (to be used in case of converter mode and different filenames);
- Codex: boolean to write ascii/binary;
- SkdTree: evaluate SkdTree true 1/false 0;
- KdTree: evaluate kdTree true 1/false 0.
- AssignRefPID: assign a reference PID on the whole geometry, after reading or just before writing. If the geometry is already pidded, translate all existent PIDs w.r.t. the reference PID assigned. Default value is RefPID = 0.
- Tolerance:value of the geometric tolerance to be used;
- Clean: clean the geometry after read true 1/false 0;
- FormatNAS: 0-singlePrecision 1-doubleprecision for writing nas files;
- ParallelRestore: set if the read geometry is parallel true 1/false 0;
In case of writing mode Geometry has to be mandatorily passed through port.
- Examples
- core_example_00001.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, ioofoam_example_00001.cpp, ioofoam_example_00002.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 151 of file MimmoGeometry.hpp.
Constructor & Destructor Documentation
◆ MimmoGeometry() [1/3]
mimmo::MimmoGeometry::MimmoGeometry | ( | MimmoGeometry::IOMode | mode = IOMode::READ | ) |
Default constructor of MimmoGeometry. Require to specify mode of the block as reader, writer or converter. NOTE: in case of converter the directory and filename will be the same for input & output (the I/O extensions have to be set by setReadFileType & setWriteFileType).
- Parameters
-
[in] mode Modality of the block -READ/WRITE/CONVERT
Definition at line 40 of file MimmoGeometry.cpp.
◆ MimmoGeometry() [2/3]
mimmo::MimmoGeometry::MimmoGeometry | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 50 of file MimmoGeometry.cpp.
◆ ~MimmoGeometry()
|
virtual |
Default destructor of MimmoGeometry.
Definition at line 76 of file MimmoGeometry.cpp.
◆ MimmoGeometry() [3/3]
mimmo::MimmoGeometry::MimmoGeometry | ( | const MimmoGeometry & | other | ) |
Copy constructor of MimmoGeometry.If to-be-copied object has an internal MimmoObject instantiated, it will be soft linked in the current class (its pointer only will be copied in m_geometry member);
Definition at line 84 of file MimmoGeometry.cpp.
Member Function Documentation
◆ _setRead()
|
protected |
It sets the condition to read the geometry on file during the execution.
- Parameters
-
[in] read Does it read the geometry in execution?
Definition at line 183 of file MimmoGeometry.cpp.
◆ _setWrite()
|
protected |
It sets the condition to write the geometry on file during the execution.
- Parameters
-
[in] write Does it write the geometry in execution?
Definition at line 250 of file MimmoGeometry.cpp.
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 1004 of file MimmoGeometry.cpp.
◆ buildPorts()
|
virtual |
Building the ports available in the class
Implements mimmo::BaseManipulation.
Definition at line 136 of file MimmoGeometry.cpp.
◆ clear()
void mimmo::MimmoGeometry::clear | ( | ) |
Clear all stuffs in your class
Definition at line 493 of file MimmoGeometry.cpp.
◆ execute()
|
virtual |
Execution command. It reads the geometry if the condition m_read is true. It writes the geometry if the condition m_write is true.
Implements mimmo::BaseManipulation.
- Examples
- core_example_00001.cpp, and utils_example_00005.cpp.
Definition at line 969 of file MimmoGeometry.cpp.
◆ fileExist()
|
protected |
Return true if a file exists on the filesystem and it is readable (check successfull opening with ifstream)
- Parameters
-
[in] filename full path to file to be checked \ return true/false if the file does/does-not exist.
Definition at line 957 of file MimmoGeometry.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 1209 of file MimmoGeometry.cpp.
◆ getCells()
bitpit::PiercedVector< bitpit::Cell > * mimmo::MimmoGeometry::getCells | ( | ) |
Return a pointer to the Cell structure of the MimmoObject geometry actually pointed or allocated by the class. If no geometry is actually available return a nullptr
- Returns
- pointer to the cells structure
Definition at line 427 of file MimmoGeometry.cpp.
◆ getCopy()
const MimmoGeometry * mimmo::MimmoGeometry::getCopy | ( | ) |
Return a const pointer to your current class.
Definition at line 147 of file MimmoGeometry.cpp.
◆ getVertices()
bitpit::PiercedVector< bitpit::Vertex > * mimmo::MimmoGeometry::getVertices | ( | ) |
Return a pointer to the Vertex structure of the MimmoObject geometry actually pointed or allocated by the class. If no geometry is actually available return a nullptr
- Returns
- pointer to the vertices structure
Definition at line 418 of file MimmoGeometry.cpp.
◆ isEmpty()
bool mimmo::MimmoGeometry::isEmpty | ( | ) |
Check if geometry is not linked or not locally instantiated in your class. True - no geometry present, False otherwise.
- Returns
- is the geometry empty?
Definition at line 484 of file MimmoGeometry.cpp.
◆ operator=()
MimmoGeometry & mimmo::MimmoGeometry::operator= | ( | MimmoGeometry | other | ) |
Assignement operator of MimmoGeometry.If to-be-copied object has an internal MimmoObject instantiated, it will be soft linked in the current class (its pointer only will be copied in m_geometry member);
Definition at line 105 of file MimmoGeometry.cpp.
◆ read()
bool mimmo::MimmoGeometry::read | ( | ) |
It reads the mesh geometry from an input file and reverse it in the internal MimmoObject container. If an external container is linked skip reading and doing nothing.
- Returns
- False if file doesn't exists or not found geometry container address.
Definition at line 673 of file MimmoGeometry.cpp.
◆ setBuildKdTree()
void mimmo::MimmoGeometry::setBuildKdTree | ( | bool | build | ) |
It sets if the KdTree of the patch has to be built during execution.
- Parameters
-
[in] build If true the KdTree is built in execution and stored in the related MimmoObject member.
Definition at line 475 of file MimmoGeometry.cpp.
◆ setBuildSkdTree()
void mimmo::MimmoGeometry::setBuildSkdTree | ( | bool | build | ) |
It sets if the SkdTree of the patch has to be built during execution.
- Parameters
-
[in] build If true the SkdTree is built in execution and stored in the related MimmoObject member.
- Examples
- manipulators_example_00002.cpp, and manipulators_example_00005.cpp.
Definition at line 466 of file MimmoGeometry.cpp.
◆ setClean()
void mimmo::MimmoGeometry::setClean | ( | bool | clean = true | ) |
Set if the geometry has to cleaned after reading. param[in] clean cleaning flag
Definition at line 384 of file MimmoGeometry.cpp.
◆ setCodex()
void mimmo::MimmoGeometry::setCodex | ( | bool | binary = true | ) |
set codex ASCII false, BINARY true for writing sessions ONLY. Default is Binary/Appended. Pay attention, binary writing is effective only those file formats which support it.(ex STL, STVTU, SQVTU, VTVTU, VHVTU)
- Parameters
-
[in] binary codex flag.
Definition at line 357 of file MimmoGeometry.cpp.
◆ setDefaults()
|
protected |
Set proper member of the class to defaults
Definition at line 155 of file MimmoGeometry.cpp.
◆ setDir()
void mimmo::MimmoGeometry::setDir | ( | std::string | dir | ) |
It sets the name of directory to read/write the geometry.
- Parameters
-
[in] dir Name of directory.
Definition at line 315 of file MimmoGeometry.cpp.
◆ setFilename()
void mimmo::MimmoGeometry::setFilename | ( | std::string | filename | ) |
It sets the name of file to read/write the geometry.
- Parameters
-
[in] filename Name of output file.
Definition at line 324 of file MimmoGeometry.cpp.
◆ setFileType() [1/2]
void mimmo::MimmoGeometry::setFileType | ( | FileType | type | ) |
It sets the type of file to read/write the geometry during the execution.
- Parameters
-
[in] type Extension of file. NOTE: in case of converter mode use separate setReadFileType and setWriteFileType
Definition at line 334 of file MimmoGeometry.cpp.
◆ setFileType() [2/2]
void mimmo::MimmoGeometry::setFileType | ( | int | type | ) |
It sets the type of file to read/write the geometry during the execution.
- Parameters
-
[in] type Label of file type (0 = bynary STL). NOTE: in case of converter mode use separate setReadFileType and setWriteFileType
Definition at line 344 of file MimmoGeometry.cpp.
◆ setFormatNAS()
void mimmo::MimmoGeometry::setFormatNAS | ( | WFORMAT | wform | ) |
It sets the format to export .nas file.
- Parameters
-
[in] wform Format of .nas file (Short/Long).
Definition at line 502 of file MimmoGeometry.cpp.
◆ setGeometry() [1/2]
void mimmo::MimmoGeometry::setGeometry | ( | int | type = 1 | ) |
Force your class to allocate an internal MimmoObject of type 1-Superficial mesh 2-Volume Mesh,3-Point Cloud, 4-3DCurve. Other internal object allocated or externally linked geometries will be destroyed/unlinked.
- Parameters
-
[in] type 1-Surface MimmoObject, 2-Volume MimmoObject, 3-Point Cloud, 4-3DCurve. Default is 1, no other type are supported
Definition at line 406 of file MimmoGeometry.cpp.
◆ setGeometry() [2/2]
void mimmo::BaseManipulation::setGeometry |
It sets the geometry linked by the manipulator object.
- Parameters
-
[in] geometry Pointer to geometry to be deformed by the manipulator object.
Definition at line 433 of file BaseManipulation.cpp.
◆ setIOMode() [1/2]
|
protected |
Set the mode of the block as reader, writer or converter.
- Parameters
-
[in] mode Modality of the block NOTE: in case of converter the directory and filename will be the same for input & output (the I/O extensions have to be set by setReadFileType & setWriteFileType).
Definition at line 307 of file MimmoGeometry.cpp.
◆ setIOMode() [2/2]
|
protected |
Set the mode of the block as reader, writer or converter.
- Parameters
-
[in] mode Modality of the block NOTE: in case of converter the directory and filename will be the same for input & output (the I/O extensions have to be set by setReadFileType & setWriteFileType).
Definition at line 280 of file MimmoGeometry.cpp.
◆ setMultiSolidSTL()
void mimmo::MimmoGeometry::setMultiSolidSTL | ( | bool | multi = true | ) |
Set ASCII Multi Solid STL writing. The method has effect only while writing standard surface triangulations in STL format type.
- Parameters
-
[in] multi boolean, true activate Multi Solid STL writing.
Definition at line 366 of file MimmoGeometry.cpp.
◆ setParallelRestore()
void mimmo::MimmoGeometry::setParallelRestore | ( | bool | parallelRestore = 0 | ) |
Set if the geometry to read is parallel or not. In case of reading a geometry dumped in mimmo (*.geomimmo) format this function has to be called in order to restore correctly a parallel patch. param[in] parallelRestore is parallel flag
Definition at line 395 of file MimmoGeometry.cpp.
◆ setPID() [1/2]
void mimmo::MimmoGeometry::setPID | ( | livector1D | pids | ) |
It sets the PIDs of all the cells of the geometry Patch.
- Parameters
-
[in] pids PIDs of the cells of geometry mesh, in compact sequential order. If pids size does not match number of current cell does nothing
Definition at line 436 of file MimmoGeometry.cpp.
◆ setPID() [2/2]
void mimmo::MimmoGeometry::setPID | ( | std::unordered_map< long, long > | pidsMap | ) |
It sets the PIDs of part of/all the cells of the geometry Patch.
- Parameters
-
[in] pidsMap PIDs map list w/ id of the cell as first value, and pid as second value.
Definition at line 444 of file MimmoGeometry.cpp.
◆ setReadDir()
void mimmo::MimmoGeometry::setReadDir | ( | std::string | dir | ) |
It sets the name of directory to read the geometry.
- Parameters
-
[in] dir Name of directory. NOTE: use it only for converter mode, otherwise setDir is recommended
- Examples
- core_example_00001.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 212 of file MimmoGeometry.cpp.
◆ setReadFilename()
void mimmo::MimmoGeometry::setReadFilename | ( | std::string | filename | ) |
It sets the name of file to read the geometry.
- Parameters
-
[in] filename Name of input file. NOTE: use it only for converter mode, otherwise setFilename is recommended
- Examples
- core_example_00001.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 221 of file MimmoGeometry.cpp.
◆ setReadFileType() [1/2]
void mimmo::MimmoGeometry::setReadFileType | ( | FileType | type | ) |
It sets the type of file to read the geometry during the execution.
- Parameters
-
[in] type Extension of file. NOTE: use it only for converter mode, otherwise setFileType is recommended
- Examples
- core_example_00001.cpp, genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00002.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 192 of file MimmoGeometry.cpp.
◆ setReadFileType() [2/2]
void mimmo::MimmoGeometry::setReadFileType | ( | int | type | ) |
It sets the type of file to read the geometry during the execution.
- Parameters
-
[in] type Label of file type (0 = bynary STL). NOTE: use it only for converter mode, otherwise setFileType is recommended
Definition at line 201 of file MimmoGeometry.cpp.
◆ setReferencePID()
void mimmo::MimmoGeometry::setReferencePID | ( | long | pid | ) |
Set a reference PID for the whole geometry cell. If the geometry is already pidded, all existent pid's will be translated w.r.t. the value of the reference pid. Default value is 0. Maximum value allowed is 30000. The effect of reference pidding will be available only after the execution of the class. After execution, reference PID will be reset ot zero.
- Parameters
-
[in] pid reference pid to assign to the geometry cells
Definition at line 456 of file MimmoGeometry.cpp.
◆ setTolerance()
void mimmo::MimmoGeometry::setTolerance | ( | double | tol | ) |
Set geometric tolerance used to perform geometric operations on the mimmo object. param[in] tol input geometric tolerance
Definition at line 375 of file MimmoGeometry.cpp.
◆ setWriteDir()
void mimmo::MimmoGeometry::setWriteDir | ( | std::string | dir | ) |
It sets the name of directory to write the geometry.
- Parameters
-
[in] dir Name of directory. NOTE: use it only for converter mode, otherwise setDir is recommended
- Examples
- genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, ioofoam_example_00001.cpp, ioofoam_example_00002.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 259 of file MimmoGeometry.cpp.
◆ setWriteFilename()
void mimmo::MimmoGeometry::setWriteFilename | ( | std::string | filename | ) |
It sets the name of file to write the geometry.
- Parameters
-
[in] filename Name of output file. NOTE: use it only for converter mode, otherwise setFilename is recommended
- Examples
- genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, ioofoam_example_00001.cpp, ioofoam_example_00002.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 268 of file MimmoGeometry.cpp.
◆ setWriteFileType() [1/2]
void mimmo::MimmoGeometry::setWriteFileType | ( | FileType | type | ) |
It sets the type of file to write the geometry during the execution.
- Parameters
-
[in] type Extension of file. NOTE: use it only for converter mode, otherwise setFileType is recommended
- Examples
- genericinput_example_00003.cpp, genericinput_example_00004.cpp, geohandlers_example_00000.cpp, geohandlers_example_00001.cpp, geohandlers_example_00002.cpp, geohandlers_example_00003.cpp, ioofoam_example_00001.cpp, ioofoam_example_00002.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, utils_example_00001.cpp, utils_example_00003.cpp, utils_example_00004.cpp, and utils_example_00005.cpp.
Definition at line 230 of file MimmoGeometry.cpp.
◆ setWriteFileType() [2/2]
void mimmo::MimmoGeometry::setWriteFileType | ( | int | type | ) |
It sets the type of file to write the geometry during the execution.
- Parameters
-
[in] type Label of file type (0 = bynary STL). NOTE: use it only for converter mode, otherwise setFileType is recommended
Definition at line 239 of file MimmoGeometry.cpp.
◆ swap()
|
protectednoexcept |
◆ write()
bool mimmo::MimmoGeometry::write | ( | ) |
It writes the mesh geometry on output .vtu file.
- Returns
- False if geometry is not linked.
Definition at line 510 of file MimmoGeometry.cpp.
Member Data Documentation
◆ m_buildKdTree
|
protected |
If true the vertex ordered KdTree of the geometry is built in execution
Definition at line 164 of file MimmoGeometry.hpp.
◆ m_buildSkdTree
|
protected |
If true the simplex ordered SkdTree of the geometry is built in execution, whenever geometry support simplicies.
Definition at line 163 of file MimmoGeometry.hpp.
◆ m_clean
|
protected |
Set if the geometry has to cleaned after reading.
Definition at line 169 of file MimmoGeometry.hpp.
◆ m_codex
|
protected |
Set codex format for writing true binary, false ascii
Definition at line 160 of file MimmoGeometry.hpp.
◆ m_multiSolidSTL
|
protected |
activate or not MultiSolid STL writing if STL writing Filetype is selected
Definition at line 166 of file MimmoGeometry.hpp.
◆ m_parallelRestore
|
protected |
Set if the geometry to read is parallel.
Definition at line 171 of file MimmoGeometry.hpp.
◆ m_read
|
protected |
If true it reads the geometry from file during the execution.
Definition at line 154 of file MimmoGeometry.hpp.
◆ m_refPID
|
protected |
Reference PID, to be assigned on all cells of geometry in read/convert mode
Definition at line 165 of file MimmoGeometry.hpp.
◆ m_rinfo
|
protected |
Info on the external file to read
Definition at line 155 of file MimmoGeometry.hpp.
◆ m_tolerance
|
protected |
Geometric tolerance of the related geometry.
Definition at line 168 of file MimmoGeometry.hpp.
◆ m_wformat
|
protected |
Format for .nas import/export. (Short/Long).
Definition at line 161 of file MimmoGeometry.hpp.
◆ m_winfo
|
protected |
Info on the external file to write
Definition at line 158 of file MimmoGeometry.hpp.
◆ m_write
|
protected |
If true it writes the geometry on file during the execution.
Definition at line 157 of file MimmoGeometry.hpp.
The documentation for this class was generated from the following files:
- src/iogeneric/MimmoGeometry.hpp
- src/iogeneric/MimmoGeometry.cpp
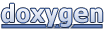