manipulators_example_00002.cpp
Example of usage of free form deformation Lattice to manipulate an input geometry.Using: MimmoGeometry, FFDLattice , GenericInput, Apply, Chain, Partition(MPI version).
To run : ./manipulators_example_00002
To run (MPI version): mpirun -np X manipulators_example_00002
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_manipulators.hpp"
#include "mimmo_iogeneric.hpp"
#if MIMMO_ENABLE_MPI
#include "mimmo_parallel.hpp"
#endif
// =================================================================================== //
void test00002() {
/*
Read a sphere from STL file. Convert mode is to save the just read geometry in
another file with name manipulators_output_00002.0000.stl
*/
mimmo0->setReadFileType(FileType::STL);
mimmo0->setWriteFileType(FileType::STL);
/*
Write final deformed mesh on file
*/
mimmo1->setWriteDir(".");
mimmo1->setWriteFileType(FileType::STL);
mimmo1->setWriteFilename("manipulators_output_00002.0001");
#if MIMMO_ENABLE_MPI
/*
Distribute target mesh among processes.
*/
mimmo::Partition* partition= new mimmo::Partition();
partition->setPartitionMethod(mimmo::PartitionMethod::PARTGEOM);
#endif
/*
Create a FFDLattice manipulator, shaped as a box.
Span and origin of the box are required.
Dimensions and nurbs degrees for each spatial directions must be provided.
Plot Optional results during execution active for FFD block.
*/
darray3E origin = {0.0, 0.0, 0.0};
darray3E span;
span[0]= 1.2;
span[1]= 1.2;
span[2]= 1.2;
/*
Set number of nodes of the mesh (dim) and degree of nurbs functions (deg).
*/
iarray3E dim, deg;
dim[0] = 20;
dim[1] = 20;
dim[2] = 20;
deg[0] = 2;
deg[1] = 2;
deg[2] = 2;
/*
Reading displacements associated ot the lattice's nodes from external
plain file.
*/
/*
It applies the deformation displacements to the original input geometry.
*/
/*
Setup pin connections.
*/
#if MIMMO_ENABLE_MPI
#else
#endif
/*
Setup execution chain.
*/
mimmo::Chain ch0;
ch0.addObject(mimmo0);
#if MIMMO_ENABLE_MPI
ch0.addObject(partition);
#endif
ch0.addObject(input);
ch0.addObject(lattice);
ch0.addObject(applier);
ch0.addObject(mimmo1);
//force the chain to plot all the optional results of its children...
//...in the path specified by the User.
/*
Execute the chain.
* Use debug flag true to full print out the execution steps.
*/
/* Clean up & exit;
*/
#if MIMMO_ENABLE_MPI
delete partition;
#endif
delete lattice;
delete applier;
delete input;
delete mimmo0;
delete mimmo1;
}
int main( int argc, char *argv[] ) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI
MPI_Init(&argc, &argv);
#endif
try{
test00002() ;
}
catch(std::exception & e){
std::cout<<"manipulators_example_00002 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI
MPI_Finalize();
#endif
return 0;
}
@ CUBE
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setBuildSkdTree(bool build)
Definition: MimmoGeometry.cpp:466
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setOutputDebugResults(std::string path)
Definition: Chain.cpp:256
void setReadFromFile(bool readFromFile)
Definition: GenericInput.cpp:123
void setFilename(std::string filename)
Definition: GenericInput.cpp:131
void setLattice(darray3E &origin, darray3E &span, ShapeType, iarray3E &dimensions, iarray3E °rees)
Definition: FFDLattice.cpp:316
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
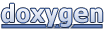