FFDLattice.hpp
180 void setLattice(darray3E & origin, darray3E & span, ShapeType, iarray3E & dimensions, iarray3E & degrees);
181 void setLattice(darray3E & origin, darray3E & span, ShapeType, dvector1D & spacing, iarray3E & degrees);
192 void plotGrid(std::string directory, std::string filename, int counter, bool binary, bool deformed);
193 void plotCloud(std::string directory, std::string filename, int counter, bool binary, bool deformed);
Abstract Interface class for Elementary Shape Representation.
Definition: BasicShapes.hpp:91
CoordType
Specify type of conditions to distribute NURBS nodes in a given coordinate of the shape.
Definition: BasicShapes.hpp:49
void returnKnotsStructure(dvector2D &, ivector2D &)
Definition: FFDLattice.cpp:194
void setDisplacements(dvecarr3E displacements)
Definition: FFDLattice.cpp:284
int accessPointIndex(int i, int j, int k)
Definition: BasicMeshes.hpp:204
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FFDLattice.cpp:1651
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void plotCloud(std::string directory, std::string filename, int counter, bool binary, bool deformed)
Definition: FFDLattice.cpp:546
void plotGrid(std::string directory, std::string filename, int counter, bool binary, bool deformed)
Definition: FFDLattice.cpp:506
ShapeType
Identifies the type of elemental shape supported by BasicShape class.
Definition: BasicShapes.hpp:38
void setLattice(darray3E &origin, darray3E &span, ShapeType, iarray3E &dimensions, iarray3E °rees)
Definition: FFDLattice.cpp:316
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FFDLattice.cpp:1596
virtual void plotOptionalResults()
Definition: FFDLattice.cpp:1428
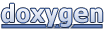