Structured 3D Cartesian Mesh. More...
#include <Lattice.hpp>
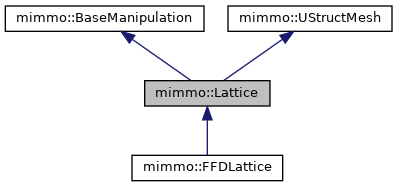
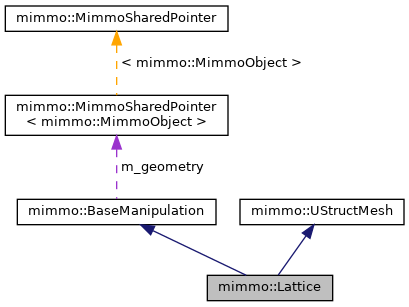
Protected Member Functions | |
virtual void | plotOptionalResults () |
void | resizeMapDof () |
void | swap (Lattice &) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
![]() | |
void | destroyNodalStructure () |
void | reshapeNodalStructure () |
void | resizeMesh () |
Protected Attributes | |
ivector1D | m_intMapDOF |
int | m_np |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
double | m_dx |
double | m_dy |
double | m_dz |
bool | m_isBuild |
int | m_nx |
int | m_ny |
int | m_nz |
bool | m_setInfLimits |
bool | m_setorigin |
bool | m_setRefSys |
bool | m_setspan |
std::unique_ptr< BasicShape > | m_shape |
dvector1D | m_xedge |
dvector1D | m_xnode |
dvector1D | m_yedge |
dvector1D | m_ynode |
dvector1D | m_zedge |
dvector1D | m_znode |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Structured 3D Cartesian Mesh.
Basically, it builds an elemental 3D shape (box, sphere, cylinder, wedge or part of them) around the geometry and set a structured cartesian mesh of control points on it (lattice). No displacements for control points and NO NURBS parameters for FFD are present in this structure, only geometrical information are stored in the object.
Ports available in Lattice class:
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | m_geometry | (MC_SCALAR, MD_MIMMO_) |
M_DIMENSION | setDimension | (MC_ARRAY3, MD_INT) |
M_INFLIMITS | setInfLimits | (MC_ARRAY3, MD_FLOAT) |
M_AXES | setRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_SPAN | setSpan | (MC_ARRAY3, MD_FLOAT) |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_SHAPE | setShape(mimmo::ShapeType) | (MC_SCALAR, MD_SHAPET) |
M_COPYSHAPE | setShape(const BasicShape* ) | (MC_SCALAR, MD_SHAPE_) |
M_SHAPEI | setShape(int) | (MC_SCALAR, MD_INT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GLOBAL | getGlobalCoords | (MC_VECARR3, MD_FLOAT) |
M_LOCAL | getLocalCoords | (MC_VECARR3, MD_FLOAT) |
M_POINT | getOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXES | getRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_SPAN | getSpan | (MC_ARRAY3, MD_FLOAT) |
M_DIMENSION | getDimension | (MC_ARRAY3, MD_INT) |
M_COPYSHAPE | getShape | (MC_SCALAR, MD_SHAPE_) |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following:
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.Lattice
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class.
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Shape: type of basic shape for your lattice. Available choice are CUBE, CYLINDER,SPHERE,WEDGE
- Origin: 3D point marking the shape barycenter
- Span: span dimensions of your shape (width-height-depth for CUBE, baseRadius-azimuthalspan-height for CYLINDER, radius-azimuthalspan-polarspan for SPHERE, triangle width-triangle height-depth for WEDGE)
- RefSystem: axes of current shape reference system. written in XML as:
<RefSystem>
<axis0> 1.0 0.0 0.0 </axis0>
<axis1> 0.0 1.0 0.0 </axis1>
<axis2> 0.0 0.0 1.0 </axis2>
</RefSystem>
- InfLimits: inferior limits for shape coordinates (meaningful only for CYLINDER AND SPHERE curvilinear coordinates)
- Dimension: number of nodes in each coordinate direction to get the structured lattice mesh
Geometry has to be mandatorily passed through port.
- Examples
- core_example_00002.cpp.
Definition at line 103 of file Lattice.hpp.
Constructor & Destructor Documentation
◆ Lattice() [1/3]
mimmo::Lattice::Lattice | ( | ) |
Basic Constructor
Definition at line 35 of file Lattice.cpp.
◆ Lattice() [2/3]
mimmo::Lattice::Lattice | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 45 of file Lattice.cpp.
◆ ~Lattice()
|
virtual |
Destructor
Definition at line 60 of file Lattice.cpp.
◆ Lattice() [3/3]
mimmo::Lattice::Lattice | ( | const Lattice & | other | ) |
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::FFDLattice.
Definition at line 292 of file Lattice.cpp.
◆ accessDOFFromGrid() [1/2]
int mimmo::Lattice::accessDOFFromGrid | ( | int | index | ) |
Find a corrispondent degree of freedom index of a lattice grid node. Not found indices are marked as -1.
- Parameters
-
[in] index lattice grid global index
- Returns
- corrispondent DOF global index
Definition at line 172 of file Lattice.cpp.
◆ accessDOFFromGrid() [2/2]
Find a corrispondent degree of freedom index list of a lattice grid node list. Not found indices are marked as -1.
- Parameters
-
[in] gNindex lattice grid global index list
- Returns
- corrispondent DOF global index list
Definition at line 194 of file Lattice.cpp.
◆ accessGridFromDOF() [1/2]
int mimmo::Lattice::accessGridFromDOF | ( | int | index | ) |
Find a corrispondent lattice grid index of a degree of freedom node. Not found indices are marked as -1.
- Parameters
-
[in] index DOF global index
- Returns
- corrispondent lattice grid global index
- Examples
- manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 183 of file Lattice.cpp.
◆ accessGridFromDOF() [2/2]
Find a corrispondent lattice grid index list of a degree of freedom node list. Not found indices are marked as -1.
- Parameters
-
[in] dofIndex DOF global index list
- Returns
- corrispondent lattice grid global index
Definition at line 211 of file Lattice.cpp.
◆ build()
|
virtual |
Build the structured mesh and create a wrapped map of effective degree of freedom of the current lattice mesh. Map is stored in internal member m_intMapDOF and accessible through methods accessDOFFromGrid and accessGridFromDOF. Reimplemented from UstructMesh::build();
- Returns
- true if the mesh is built.
Reimplemented from mimmo::UStructMesh.
Reimplemented in mimmo::FFDLattice.
Definition at line 270 of file Lattice.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::FFDLattice.
Definition at line 86 of file Lattice.cpp.
◆ clearLattice()
void mimmo::Lattice::clearLattice | ( | ) |
Clean every setting and data hold by the class
Definition at line 115 of file Lattice.cpp.
◆ execute()
|
virtual |
Execute your object, that is, recall the method Lattice::build().
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::FFDLattice.
Definition at line 280 of file Lattice.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::FFDLattice.
Definition at line 383 of file Lattice.cpp.
◆ getGlobalCoords()
dvecarr3E mimmo::Lattice::getGlobalCoords | ( | ) |
Return position of effective mesh nodes in the lattice, in absolute reference system. Reimplemented from UstructMesh::getGlobalCoords.
- Returns
- effective mesh nodes position
Definition at line 135 of file Lattice.cpp.
◆ getLocalCoords()
dvecarr3E mimmo::Lattice::getLocalCoords | ( | ) |
Return position of effective mesh nodes in the lattice, in local shape reference system. Reimplemented from UstructMesh::getLocalCoords.
- Returns
- effective mesh nodes position
Definition at line 153 of file Lattice.cpp.
◆ getNNodes()
int mimmo::Lattice::getNNodes | ( | ) |
Get the total number of control nodes.
- Returns
- number of control nodes
- Examples
- manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 125 of file Lattice.cpp.
◆ plotCloud()
void mimmo::Lattice::plotCloud | ( | std::string | directory, |
std::string | filename, | ||
int | counter, | ||
bool | binary | ||
) |
Plot your current lattice as a point cloud to VTK *.vtu file. Wrapped method of plotCloud of its base class UStructMesh.
- Parameters
-
[in] directory output directory [in] filename output filename w/out tag [in] counter integer identifier of the file [in] binary boolean flag for 0-"ascii" or 1-"appended" writing
Definition at line 251 of file Lattice.cpp.
◆ plotGrid()
void mimmo::Lattice::plotGrid | ( | std::string | directory, |
std::string | filename, | ||
int | counter, | ||
bool | binary | ||
) |
Plot your current lattice as a structured grid to a VTK *.vtu file. Wrapped method of plotGrid of its base class UStructMesh.
- Parameters
-
[in] directory output directory [in] filename output filename w/out tag [in] counter integer identifier of the file [in] binary boolean flag for 0-"ascii" or 1-"appended" writing
Definition at line 230 of file Lattice.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot Optional results of the class, that is, the lattice grid as an unstructured mesh of hexahedrons in a VTK *.vtu format.
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::FFDLattice.
Definition at line 446 of file Lattice.cpp.
◆ resizeMapDof()
|
protected |
Resize map of effective nodes of the lattice grid to fit a total number od degree of freedom nx*ny*nz. Old structure is deleted and reset to zero.
Definition at line 524 of file Lattice.cpp.
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_intMapDOF
|
protected |
Map of grid nodes -> degrees of freedom of lattice
Definition at line 107 of file Lattice.hpp.
◆ m_np
|
protected |
Number of control nodes.
Definition at line 106 of file Lattice.hpp.
The documentation for this class was generated from the following files:
- src/core/Lattice.hpp
- src/core/Lattice.cpp
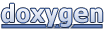