Lattice.cpp
89 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, Lattice>(&m_geometry, M_GEOM, true));
90 built = (built && createPortIn<iarray3E, Lattice>(this, &mimmo::Lattice::setDimension, M_DIMENSION));
91 built = (built && createPortIn<darray3E, Lattice>(this, &mimmo::Lattice::setInfLimits, M_INFLIMITS));
92 built = (built && createPortIn<dmatrix33E, Lattice>(this, &mimmo::Lattice::setRefSystem, M_AXES));
95 built = (built && createPortIn<mimmo::ShapeType, Lattice>(this, &mimmo::Lattice::setShape, M_SHAPE));
96 built = (built && createPortIn<const BasicShape *, Lattice>(this, &mimmo::Lattice::setShape, M_COPYSHAPE));
100 built = (built && createPortOut<dvecarr3E, Lattice>(this, &mimmo::Lattice::getGlobalCoords, M_GLOBAL));
101 built = (built && createPortOut<dvecarr3E, Lattice>(this, &mimmo::Lattice::getLocalCoords, M_LOCAL));
103 built = (built && createPortOut<dmatrix33E, Lattice>(this, &mimmo::Lattice::getRefSystem, M_AXES));
105 built = (built && createPortOut<iarray3E, Lattice>(this, &mimmo::Lattice::getDimension, M_DIMENSION));
106 built = (built && createPortOut<BasicShape *, Lattice>(this, &mimmo::Lattice::getShape, M_COPYSHAPE));
107 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, Lattice>(this, &mimmo::Lattice::getGeometry, M_GEOM));
@ CUBE
void plotGrid(std::string directory, std::string filename, int counter, bool binary)
Definition: Lattice.cpp:230
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicMeshes.cpp:619
int accessPointIndex(int i, int j, int k)
Definition: BasicMeshes.hpp:204
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
std::array< CoordType, 3 > getCoordType()
Definition: BasicMeshes.cpp:316
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Lattice.cpp:383
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
int posVectorFind(std::vector< T > &vect, T &target)
Definition: customOperators.hpp:155
void plotCloud(std::string directory, std::string filename, int counter, bool binary)
Definition: Lattice.cpp:251
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Lattice.cpp:292
void plotCloud(std::string &, std::string, int, bool, const ivector1D &labels, dvecarr3E *extPoints=nullptr)
Definition: BasicMeshes.cpp:1396
@ WEDGE
@ SPHERE
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void plotGrid(std::string &, std::string, int, bool, const ivector1D &labels, dvecarr3E *extPoints=nullptr)
Definition: BasicMeshes.cpp:1492
@ PERIODIC
void setInfLimits(double val, int dir)
Definition: BasicMeshes.cpp:588
@ CYLINDER
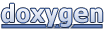