Collection of general methods and functions related to mimmo. More...

Functions | |
std::array< double, 3 > | operator- (const bitpit::Vertex &v1, const bitpit::Vertex &v2) |
int | countSubstring (const std::string &str, const std::string &sub) |
template<class T > | |
void | freeContainer (std::vector< T > &t) |
template<class T > | |
T | summing_Vector (std::vector< T > &t) |
bool | logical_summing (bool &root, bool &target, bool *policy) |
int | logical_summing (int &root, int &target, bool policy) |
template<class T > | |
T | getSign (T &t) |
template<class T > | |
int | posVectorFind (std::vector< T > &vect, T &target) |
template<class T , size_t d> | |
int | posVectorFind (std::array< T, d > &vect, T &target) |
template<class T > | |
bool | checkVectorFind (std::vector< T > &vect, T &target) |
template<class T , size_t d> | |
bool | checkVectorFind (std::array< T, d > &vect, T &target) |
template<class T , size_t d> | |
std::vector< T > | conVect (std::array< T, d > &origin) |
template<class T , size_t d> | |
std::array< T, d > | conArray (std::vector< T > &origin) |
template<class T , size_t d> | |
std::vector< std::vector< T > > | conVect (std::vector< std::array< T, d > > &origin) |
template<class T , size_t d> | |
std::vector< std::array< T, d > > | conArray (std::vector< std::vector< T > > &origin) |
template<class T > | |
T | findPosition (T &value, std::vector< T > &source) |
template<class T > | |
std::vector< T > | getVectorSubset (int i, int j, std::vector< T > &source) |
template<class T > | |
void | fillVectorSubset (int i, int j, std::vector< T > &source, T cValue) |
Detailed Description
Collection of general methods and functions related to mimmo.
Function Documentation
◆ checkVectorFind() [1/2]
|
inline |
Check if a target element belong to a std::array list. If the element is not found, return false.
- Parameters
-
[in] vect std::array list [in] target target element
- Returns
- true if the element belongs to the list
Definition at line 205 of file customOperators.hpp.
◆ checkVectorFind() [2/2]
|
inline |
Check if a target element belong to a std::vector list. If the element is not found, return false.
- Parameters
-
[in] vect std::vector list [in] target target element
- Returns
- true if the element belongs to the list
Definition at line 190 of file customOperators.hpp.
◆ conArray() [1/2]
|
inline |
Converter from vector of vectors to vector of arrays
- Parameters
-
[in] origin std::vector of std::vector's
- Returns
- std::vector of std::array's
Definition at line 257 of file customOperators.hpp.
◆ conArray() [2/2]
|
inline |
Converter from vector to array
- Parameters
-
[in] origin std::vector
- Returns
- std::array result
Definition at line 231 of file customOperators.hpp.
◆ conVect() [1/2]
|
inline |
Converter from array to vector
- Parameters
-
[in] origin std::array
- Returns
- std::vector result
Definition at line 218 of file customOperators.hpp.
◆ conVect() [2/2]
|
inline |
Converter from vector of arrays to vector of vectors
- Parameters
-
[in] origin std::vector of std::array's
- Returns
- std::vector of std::vector's
Definition at line 244 of file customOperators.hpp.
◆ countSubstring()
int countSubstring | ( | const std::string & | str, |
const std::string & | sub | ||
) |
Count of non-overlapping occurrences of a sub-string in a string
- Returns
- count of non-overlapping occurrences of a sub-string in a string
- Parameters
-
[in] str input string [in] sub input sub-string
Definition at line 46 of file customOperators.cpp.
◆ fillVectorSubset()
void fillVectorSubset | ( | int | i, |
int | j, | ||
std::vector< T > & | source, | ||
T | cValue | ||
) |
Fill std::vector structure subset containing all source elements included between two consecutive local positions i and j, with j-th element excluded. With a custom value. According to i and j values, the following behaviours occurr:
- i < 0, j < 0 ==> return empty subset;
- i >= source.size(), j >= source.size() ==> return empty subset;
- i < 0, j >= source.size() ==> return whole source;
- i >= source.size(), j < 0 ==> return empty subset;
- i = j ==> return whole source, reordered starting from i-th element;
- i < j ==> return subset from i-th element up to j-th element excluded
- j > i ==> source looped, return subset with ith -> up to vector end + vector begin -> up to jth element
- Parameters
-
[in] i start position [in] j end position [in,out] source std::vector< > structure [in] cValue custom value
Definition at line 350 of file customOperators.hpp.
◆ findPosition()
T findPosition | ( | T & | value, |
std::vector< T > & | source | ||
) |
Find position of a target T value in a vector source of T elements. Returns -1 if value is not found.
- Parameters
-
[in] value target [in] source vector source
- Returns
- position value
Definition at line 272 of file customOperators.hpp.
◆ freeContainer()
void freeContainer | ( | std::vector< T > & | t | ) |
Template for freeing any std::vector container, by swap
- Parameters
-
[in] t std::vector structure to be freed.
Definition at line 53 of file customOperators.hpp.
◆ getSign()
T getSign | ( | T & | t | ) |
Get sign of a scalar element of Class T
- Parameters
-
[in] t element
- Returns
- return sign of the element
Definition at line 141 of file customOperators.hpp.
◆ getVectorSubset()
std::vector< T > getVectorSubset | ( | int | i, |
int | j, | ||
std::vector< T > & | source | ||
) |
Get a subset of a std::vector structure containing all source elements included between two consecutive local positions i and j, with j-th element excluded. According to i and j values, the following behaviours occurr:
- i < 0, j < 0 ==> fill nothing;
- i >= source.size(), j >= source.size() ==> fill nothing;
- i < 0, j >= source.size() ==> fill whole source;
- i >= source.size(), j < 0 ==> fill nothing;
- i = j ==> fill whole source;
- i < j ==> fill subset from i-th element up to j-th element excluded
- j > i ==> source looped, fill subset from ith -> up to vector end + vector begin -> up to jth element
- Parameters
-
[in] i start position [in] j end position [in] source std::vector< > structure
- Returns
- vector subset
Definition at line 300 of file customOperators.hpp.
◆ logical_summing() [1/2]
|
inline |
Logical summing of given booleans, according to a given policy. The policy array is an array of 3 booleans, initialized to false.
- policy[0] = true -> function return the result of target.
- policy[1] = true -> function return the boolean sum of root and target.
- policy[2] = true -> function return the boolean difference of root and target.
An exception is made if root is FALSE and target is TRUE; the boolean difference here is handled as FALSE. If more than one TRUE is defined in the policy array, the policy with high index in the array is applied.
- Parameters
-
[in] root first boolean [in] target second boolean [in] policy pointer to a boolean C-array of 3 Elements.
- Returns
- boolean true/false
Definition at line 90 of file customOperators.hpp.
◆ logical_summing() [2/2]
|
inline |
Logical summing of given booleans, according to a given policy. The policy array is an array of 3 booleans, initialized to false.
- policy[0] = true -> function return the result of target.
- policy[1] = true -> function return the boolean sum of root and target.
- policy[2] = true -> function return the boolean difference of root and target.
An exception is made if root is FALSE and target is TRUE; the boolean difference here is handled as FALSE. If more than one TRUE is defined in the policy array, the policy with high index in the array is applied.
- Parameters
-
[in] root first boolean [in] target second boolean [in] policy pointer to a boolean C-array of 3 Elements.
- Returns
- integer 1/0 for true/false
Definition at line 122 of file customOperators.hpp.
◆ operator-()
std::array<double, 3> operator- | ( | const bitpit::Vertex & | v1, |
const bitpit::Vertex & | v2 | ||
) |
Custom Operator - for bitpit::Vertex
- Parameters
-
[in] v1 first vertex [in] v2 second vertex
- Returns
- difference vertex coordinates V1-V2;
Definition at line 34 of file customOperators.cpp.
◆ posVectorFind() [1/2]
|
inline |
Get index position of a target element in an std::array list. If the element is not found, return -1.
- Parameters
-
[in] vect std::array list [in] target target element
- Returns
- position of the element in the list
Definition at line 173 of file customOperators.hpp.
◆ posVectorFind() [2/2]
|
inline |
Get index position of a target element in a std::vector list. If the element is not found, return -1.
- Parameters
-
[in] vect std::vector list [in] target target element
- Returns
- position of the element in the list
Definition at line 155 of file customOperators.hpp.
◆ summing_Vector()
T summing_Vector | ( | std::vector< T > & | t | ) |
Template for summing elements of any std::vector container, provided that sum operators is defined for your element class in std library
- Parameters
-
[in] t std::vector structure to summed.
Definition at line 63 of file customOperators.hpp.
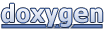