Class for 3D uniform structured mesh. More...
#include <BasicMeshes.hpp>
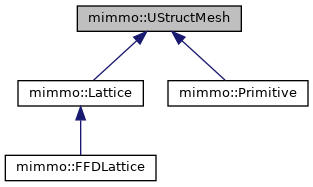
Protected Member Functions | |
void | destroyNodalStructure () |
void | reshapeNodalStructure () |
void | resizeMesh () |
Protected Attributes | |
double | m_dx |
double | m_dy |
double | m_dz |
bool | m_isBuild |
int | m_nx |
int | m_ny |
int | m_nz |
bool | m_setInfLimits |
bool | m_setorigin |
bool | m_setRefSys |
bool | m_setspan |
std::unique_ptr< BasicShape > | m_shape |
dvector1D | m_xedge |
dvector1D | m_xnode |
dvector1D | m_yedge |
dvector1D | m_ynode |
dvector1D | m_zedge |
dvector1D | m_znode |
Detailed Description
Class for 3D uniform structured mesh.
Interface class for uniform structured grids, suitable for derivation of meshes on pure cartesian, cylindrical or spherical coordinates system. The class retains internal members of Class BasicShape who determine the shape of your current grid. The mesh works and its nodal structures are defined in the its local reference frame, that is, the local reference frame retained by its core BasicShape object.
Definition at line 44 of file BasicMeshes.hpp.
Constructor & Destructor Documentation
◆ UStructMesh() [1/2]
mimmo::UStructMesh::UStructMesh | ( | ) |
Basic Constructor. Default Shape CUBE
Definition at line 35 of file BasicMeshes.cpp.
◆ ~UStructMesh()
|
virtual |
Basic destructor
Definition at line 56 of file BasicMeshes.cpp.
◆ UStructMesh() [2/2]
mimmo::UStructMesh::UStructMesh | ( | const UStructMesh & | other | ) |
Copy Constructor.
- Parameters
-
[in] other UStructMesh object where copy from
Definition at line 62 of file BasicMeshes.cpp.
Member Function Documentation
◆ accessCellIndex() [1/2]
int mimmo::UStructMesh::accessCellIndex | ( | int | i, |
int | j, | ||
int | k | ||
) |
Return global index of the cell given its cartesian indices. Follows the ordering sequences z-y-x
- Parameters
-
[in] i x cartesian index [in] j y cartesian index [in] k z cartesian index
- Returns
- global index
Definition at line 1049 of file BasicMeshes.cpp.
◆ accessCellIndex() [2/2]
void mimmo::UStructMesh::accessCellIndex | ( | int | N_, |
int & | i, | ||
int & | j, | ||
int & | k | ||
) |
- Returns
- cartesian indices of the cell given its global index. Follows the ordering sequences z-y-x
- Parameters
-
[in] N_ global index [out] i x cartesian index [out] j y cartesian index [out] k z cartesian index
Definition at line 1063 of file BasicMeshes.cpp.
◆ accessPointIndex() [1/2]
|
inline |
- Returns
- global index of the point given its cartesian indices. Follows the ordering sequences z-y-x
- Parameters
-
[in] i x cartesian index [in] j y cartesian index [in] k z cartesian index
- Examples
- manipulators_example_00003.cpp, and manipulators_example_00004.cpp.
Definition at line 204 of file BasicMeshes.hpp.
◆ accessPointIndex() [2/2]
void mimmo::UStructMesh::accessPointIndex | ( | int | N_, |
int & | i, | ||
int & | j, | ||
int & | k | ||
) |
- Returns
- cartesian indices of the point given its global index. Follows the ordering sequences z-y-x
- Parameters
-
[in] N_ global index [out] i x cartesian index [out] j y cartesian index [out] k z cartesian index
Definition at line 1078 of file BasicMeshes.cpp.
◆ build()
|
virtual |
Apply stored mesh information and build the mesh. If no core shape is build, does nothing and exit.
Reimplemented in mimmo::FFDLattice, and mimmo::Lattice.
Definition at line 1622 of file BasicMeshes.cpp.
◆ clearMesh()
void mimmo::UStructMesh::clearMesh | ( | ) |
Clear the Mesh structure. Unlink external shapes or destroy internal shapes. Destroy nodal structure.
Definition at line 987 of file BasicMeshes.cpp.
◆ destroyNodalStructure()
|
protected |
Destroy all nodal structures of the mesh.
Definition at line 1585 of file BasicMeshes.cpp.
◆ execute()
void mimmo::UStructMesh::execute | ( | ) |
Execute the object, that is build the mesh with current stored parameters.
Definition at line 1670 of file BasicMeshes.cpp.
◆ getCellNeighs() [1/2]
ivector1D mimmo::UStructMesh::getCellNeighs | ( | int | index | ) |
- Returns
- the list of neighbor vertices (by their global indices and in VTK-hexahedron ordering) of a given cell
- Parameters
-
[in] index cell global index
Definition at line 478 of file BasicMeshes.cpp.
◆ getCellNeighs() [2/2]
ivector1D mimmo::UStructMesh::getCellNeighs | ( | int | i_, |
int | j_, | ||
int | k_ | ||
) |
- Returns
- the list of neighbor vertices (by their global indices and in VTK-hexahedron ordering) of a given cell
- Parameters
-
[in] i_ x coordinate cell index [in] j_ y coordinate cell index [in] k_ z coordinate cell index
Definition at line 459 of file BasicMeshes.cpp.
◆ getCoordType() [1/2]
std::array< CoordType, 3 > mimmo::UStructMesh::getCoordType | ( | ) |
Return coordinates type of a BasicShape mesh core. See CoordType enum.
- Returns
- Type of all the cooordinates.
Definition at line 316 of file BasicMeshes.cpp.
◆ getCoordType() [2/2]
CoordType mimmo::UStructMesh::getCoordType | ( | int | i | ) |
- Returns
- coordinate type of a component of a BasicShape mesh core. See CoordType enum.
- Parameters
-
[in] i index of component.
Definition at line 289 of file BasicMeshes.cpp.
◆ getCoordTypex()
CoordType mimmo::UStructMesh::getCoordTypex | ( | ) |
- Returns
- coordinate type of component 0 a BasicShape mesh core. See CoordType enum.
Definition at line 280 of file BasicMeshes.cpp.
◆ getCoordTypey()
CoordType mimmo::UStructMesh::getCoordTypey | ( | ) |
- Returns
- coordinate type of component 1 a BasicShape mesh core. See CoordType enum.
Definition at line 298 of file BasicMeshes.cpp.
◆ getCoordTypez()
CoordType mimmo::UStructMesh::getCoordTypez | ( | ) |
- Returns
- coordinate type of component 2 a BasicShape mesh core. See CoordType enum.
Definition at line 307 of file BasicMeshes.cpp.
◆ getDimension()
iarray3E mimmo::UStructMesh::getDimension | ( | ) |
- Returns
- current dimensions of the mesh (number of mesh nodes in each direction)
Definition at line 337 of file BasicMeshes.cpp.
◆ getGlobalCCell() [1/2]
darray3E mimmo::UStructMesh::getGlobalCCell | ( | int | index | ) |
- Returns
- the n-th center-cell coordinates in global absolute reference frame, given its global cell index on the mesh.
- Parameters
-
[in] index cell index in the global nodal list.
Definition at line 412 of file BasicMeshes.cpp.
◆ getGlobalCCell() [2/2]
darray3E mimmo::UStructMesh::getGlobalCCell | ( | int | i_, |
int | j_, | ||
int | k_ | ||
) |
- Returns
- the n-th center cell coordinates in global absolute reference frame, given its cartesian cell indices on the mesh.
- Parameters
-
[in] i_ x cartesian index. [in] j_ y cartesian index. [in] k_ z cartesian index.
Definition at line 424 of file BasicMeshes.cpp.
◆ getGlobalCellCentroids()
dvecarr3E mimmo::UStructMesh::getGlobalCellCentroids | ( | ) |
- Returns
- complete list of mesh cell centers, in global absolute reference system
Definition at line 526 of file BasicMeshes.cpp.
◆ getGlobalCoords()
dvecarr3E mimmo::UStructMesh::getGlobalCoords | ( | ) |
- Returns
- teh complete list of mesh nodes, in global absolute reference system
Definition at line 501 of file BasicMeshes.cpp.
◆ getGlobalPoint() [1/2]
darray3E mimmo::UStructMesh::getGlobalPoint | ( | int | index | ) |
- Returns
- the n-th nodal vertex coordinates in global absolute reference frame, given its global point index on the mesh.
- Parameters
-
[in] index point index in the global nodal list.
Definition at line 435 of file BasicMeshes.cpp.
◆ getGlobalPoint() [2/2]
darray3E mimmo::UStructMesh::getGlobalPoint | ( | int | i_, |
int | j_, | ||
int | k_ | ||
) |
- Returns
- the n-th nodal vertex coordinates in global absolute reference frame, given its cartesian point indices on the mesh.
- Parameters
-
[in] i_ x cartesian index. [in] j_ y cartesian index. [in] k_ z cartesian index.
Definition at line 446 of file BasicMeshes.cpp.
◆ getInfLimits()
darray3E mimmo::UStructMesh::getInfLimits | ( | ) |
- Returns
- current lower limits of coordinates in BasicShape core of the mesh
Definition at line 239 of file BasicMeshes.cpp.
◆ getLocalCCell() [1/2]
darray3E mimmo::UStructMesh::getLocalCCell | ( | int | index | ) |
- Returns
- the n-th center-cell coordinates in local mesh reference frame, given its global cell index on the mesh.
- Parameters
-
[in] index cell index in the global nodal list.
Definition at line 351 of file BasicMeshes.cpp.
◆ getLocalCCell() [2/2]
darray3E mimmo::UStructMesh::getLocalCCell | ( | int | i_, |
int | j_, | ||
int | k_ | ||
) |
- Returns
- the n-th center-cell coordinates in local mesh reference frame, given its cartesian cell indices on the mesh.
- Parameters
-
[in] i_ x cartesian index. [in] j_ y cartesian index. [in] k_ z cartesian index.
Definition at line 367 of file BasicMeshes.cpp.
◆ getLocalCellCentroids()
dvecarr3E mimmo::UStructMesh::getLocalCellCentroids | ( | ) |
- Returns
- complete list of mesh cell centers, in local shape reference system
Definition at line 513 of file BasicMeshes.cpp.
◆ getLocalCoords()
dvecarr3E mimmo::UStructMesh::getLocalCoords | ( | ) |
- Returns
- the complete list of mesh nodes, in local shape reference system
Definition at line 488 of file BasicMeshes.cpp.
◆ getLocalPoint() [1/2]
darray3E mimmo::UStructMesh::getLocalPoint | ( | int | index | ) |
- Returns
- teh n-th nodal vertex coordinates in local mesh reference frame, given its global point index on the mesh.
- Parameters
-
[in] index point index in the global nodal list.
- Examples
- manipulators_example_00004.cpp.
Definition at line 382 of file BasicMeshes.cpp.
◆ getLocalPoint() [2/2]
darray3E mimmo::UStructMesh::getLocalPoint | ( | int | i_, |
int | j_, | ||
int | k_ | ||
) |
- Returns
- the n-th nodal vertex coordinates in local reference frame, given its cartesian point indices on the mesh.
- Parameters
-
[in] i_ x cartesian index. [in] j_ y cartesian index. [in] k_ z cartesian index.
Definition at line 397 of file BasicMeshes.cpp.
◆ getLocalSpan()
darray3E mimmo::UStructMesh::getLocalSpan | ( | ) |
- Returns
- local span of the primitive shape associated to BasicShape core of the mesh
Definition at line 263 of file BasicMeshes.cpp.
◆ getOrigin()
darray3E mimmo::UStructMesh::getOrigin | ( | ) |
- Returns
- current origin of BasicShape core of the mesh
Definition at line 223 of file BasicMeshes.cpp.
◆ getRefSystem()
dmatrix33E mimmo::UStructMesh::getRefSystem | ( | ) |
- Returns
- current local Reference System af axes
Definition at line 247 of file BasicMeshes.cpp.
◆ getScaling()
darray3E mimmo::UStructMesh::getScaling | ( | ) |
- Returns
- actual scaling to primitive shape used in BasicShape core of the mesh
Definition at line 255 of file BasicMeshes.cpp.
◆ getShape()
BasicShape * mimmo::UStructMesh::getShape | ( | ) | const |
- Returns
- a const pointer to the inner BasicShape object the current mesh is built on. Const method
- a pointer to the inner BasicShape object the current mesh is built on
Definition at line 209 of file BasicMeshes.cpp.
◆ getShapeType()
ShapeType mimmo::UStructMesh::getShapeType | ( | ) |
- Returns
- type of shape associated to mesh core. See ShapeType enum
Definition at line 271 of file BasicMeshes.cpp.
◆ getSpacing()
darray3E mimmo::UStructMesh::getSpacing | ( | ) |
- Returns
- current mesh spacing in all three coordinates
Definition at line 327 of file BasicMeshes.cpp.
◆ getSpan()
darray3E mimmo::UStructMesh::getSpan | ( | ) |
- Returns
- current span of BasicShape core of the mesh
Definition at line 231 of file BasicMeshes.cpp.
◆ interpolateCellData() [1/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] celldata data field defined on centercells
Definition at line 1228 of file BasicMeshes.cpp.
◆ interpolateCellData() [2/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] celldata data field defined on centercells
Definition at line 1152 of file BasicMeshes.cpp.
◆ interpolateCellData() [3/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] celldata data field defined on centercells
Definition at line 1190 of file BasicMeshes.cpp.
◆ interpolatePointData() [1/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] pointdata data field defined on grid points
Definition at line 1348 of file BasicMeshes.cpp.
◆ interpolatePointData() [2/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] pointdata data field defined on grid points
Definition at line 1266 of file BasicMeshes.cpp.
◆ interpolatePointData() [3/3]
- Returns
- interpolated value of a given data field on a target point inside the mesh
- Parameters
-
[in] point target point [in] pointdata data field defined on grid points
Definition at line 1306 of file BasicMeshes.cpp.
◆ isBuilt()
bool mimmo::UStructMesh::isBuilt | ( | ) |
- Returns
- true if mesh is built according to the currently set parameters,
Definition at line 1677 of file BasicMeshes.cpp.
◆ locateCellByPoint() [1/2]
void mimmo::UStructMesh::locateCellByPoint | ( | darray3E & | point, |
int & | i, | ||
int & | j, | ||
int & | k | ||
) |
- Returns
- cartesian indices of the cell containing the target point in global reference frame.
- Parameters
-
[in] point 3D coordinate of target point [out] i x cell index [out] j y cell index [out] k z cell index
Definition at line 1018 of file BasicMeshes.cpp.
◆ locateCellByPoint() [2/2]
void mimmo::UStructMesh::locateCellByPoint | ( | dvector1D & | point, |
int & | i, | ||
int & | j, | ||
int & | k | ||
) |
- Returns
- cartesian indices of the cell containing the target point in global reference framne
- Parameters
-
[in] point 3D coordinate of target point [out] i x cell index [out] j y cell index [out] k z cell index
Definition at line 1036 of file BasicMeshes.cpp.
◆ operator=()
UStructMesh & mimmo::UStructMesh::operator= | ( | UStructMesh | other | ) |
Assignement Operator.
- Parameters
-
[in] other UStructMesh object where copy from
Definition at line 128 of file BasicMeshes.cpp.
◆ plotCloud()
void mimmo::UStructMesh::plotCloud | ( | std::string & | folder, |
std::string | outfile, | ||
int | counterFile, | ||
bool | codexFlag, | ||
const ivector1D & | labels, | ||
dvecarr3E * | extPoints = nullptr |
||
) |
Write the grid as a point cloud in a *.vtu file.
- Parameters
-
[in] folder output directory path [in] outfile output namefile w/out tag [in] counterFile integer to mark output with a counter number [in] codexFlag boolean to distinguish between "ascii" false, and "appended" true [in] labels list of labels associated to lattice points [in] extPoints OPTIONAL. If defined, use the current vertexList and write them as point cloud, provided that coherent dimensions with the mesh are set. If Not, write the current mesh vertices.
Definition at line 1396 of file BasicMeshes.cpp.
◆ plotCloudScalar()
void mimmo::UStructMesh::plotCloudScalar | ( | std::string | folder, |
std::string | outfile, | ||
int | counterFile, | ||
bool | codexFlag, | ||
dvector1D & | data | ||
) |
Write the grid as a point cloud in a *.vtu file with an attached scalar.
- Parameters
-
[in] folder output directory path [in] outfile output namefile w/out tag [in] counterFile integer to mark output with a counter number [in] codexFlag boolean to distinguish between "ascii" false, and "appended" true [in] data scalar to be attached
Definition at line 1448 of file BasicMeshes.cpp.
◆ plotGrid()
void mimmo::UStructMesh::plotGrid | ( | std::string & | folder, |
std::string | outfile, | ||
int | counterFile, | ||
bool | codexFlag, | ||
const ivector1D & | labels, | ||
dvecarr3E * | extPoints = nullptr |
||
) |
Write the grid as a hexahedrical one in a *.vtu file.
- Parameters
-
[in] folder output directory path [in] outfile output namefile w/out tag [in] counterFile integer to mark output with a counter number [in] codexFlag boolean to distinguish between "ascii" false, and "appended" true [in] labels list of labels associated to lattice nodes. [in] extPoints OPTIONAL. If defined, use the current vertexList and write them as point cloud, provided that coherent dimensions with the mesh are set. If Not, write the current mesh vertices.
Definition at line 1492 of file BasicMeshes.cpp.
◆ plotGridScalar()
void mimmo::UStructMesh::plotGridScalar | ( | std::string | folder, |
std::string | outfile, | ||
int | counterFile, | ||
bool | codexFlag, | ||
dvector1D & | data | ||
) |
Write the grid as a hexahedrical one in a *.vtu file.
- Parameters
-
[in] folder output directory path [in] outfile output namefile w/out tag [in] counterFile integer to mark output with a counter number [in] codexFlag boolean to distinguish between "ascii" false, and "appended" true [in] data Scalar field
Definition at line 1544 of file BasicMeshes.cpp.
◆ reshapeNodalStructure()
|
protected |
Destroy all nodal structures of the mesh, and reinitialize them to current mesh dimensions.
Definition at line 1598 of file BasicMeshes.cpp.
◆ resizeMesh()
|
protected |
Resize nodal structures to current dimensions set
Definition at line 1606 of file BasicMeshes.cpp.
◆ setCoordType() [1/2]
void mimmo::UStructMesh::setCoordType | ( | CoordType | type, |
int | i | ||
) |
Set coordinate type of a component of a BasicShape mesh core. See CoordType enum.
- Parameters
-
[in] type CoordType enum input [in] i index of component.
- Examples
- manipulators_example_00004.cpp.
Definition at line 832 of file BasicMeshes.cpp.
◆ setCoordType() [2/2]
void mimmo::UStructMesh::setCoordType | ( | std::array< CoordType, 3 > | types | ) |
Set coordinates type of a BasicShape mesh core. See CoordType enum.
- Parameters
-
[in] types array of CoordType enum for all the cooordinates.
Definition at line 861 of file BasicMeshes.cpp.
◆ setCoordTypex()
void mimmo::UStructMesh::setCoordTypex | ( | CoordType | type | ) |
Set coordinate type of component 0 of a BasicShape mesh core. See CoordType enum.
Definition at line 821 of file BasicMeshes.cpp.
◆ setCoordTypey()
void mimmo::UStructMesh::setCoordTypey | ( | CoordType | type | ) |
Set coordinate type of component 1 a BasicShape mesh core. See CoordType enum.
- Parameters
-
[in] type CoordType enum input
Definition at line 842 of file BasicMeshes.cpp.
◆ setCoordTypez()
void mimmo::UStructMesh::setCoordTypez | ( | CoordType | type | ) |
Set coordinate type of component 2 a BasicShape mesh core. See CoordType enum.
- Parameters
-
[in] type CoordType enum input
Definition at line 852 of file BasicMeshes.cpp.
◆ setDimension() [1/2]
void mimmo::UStructMesh::setDimension | ( | iarray3E | dim | ) |
Set the dimensions of the mesh. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] dim number of mesh nodes in each direction
Definition at line 690 of file BasicMeshes.cpp.
◆ setDimension() [2/2]
void mimmo::UStructMesh::setDimension | ( | ivector1D | dim | ) |
Set the dimensions of the mesh. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] dim number of mesh nodes in each direction
- Examples
- core_example_00002.cpp, genericinput_example_00003.cpp, ioofoam_example_00001.cpp, and utils_example_00004.cpp.
Definition at line 676 of file BasicMeshes.cpp.
◆ setInfLimits() [1/2]
void mimmo::UStructMesh::setInfLimits | ( | darray3E | inflim | ) |
Set coordinates inferior limits of the shape, according to its local reference system. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] inflim coordinates inferior limits
Definition at line 603 of file BasicMeshes.cpp.
◆ setInfLimits() [2/2]
void mimmo::UStructMesh::setInfLimits | ( | double | inflim, |
int | dir | ||
) |
Set inferior limits of your shape, according to its local reference system. Info is just passed and stored in memory, but no modifications are a*pplied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] inflim coordinate inferior limit [in] dir 0,1,2 int flag identifying coordinate
- Examples
- core_example_00002.cpp.
Definition at line 588 of file BasicMeshes.cpp.
◆ setMesh() [1/4]
void mimmo::UStructMesh::setMesh | ( | BasicShape * | shape, |
dvector1D & | spacing | ||
) |
Set the mesh, according to the following input parameters
- Parameters
-
[in] shape pointer to an external allocated BasicShape object [in] spacing fixed spacing for each coordinate
Definition at line 948 of file BasicMeshes.cpp.
◆ setMesh() [2/4]
void mimmo::UStructMesh::setMesh | ( | BasicShape * | shape, |
iarray3E & | dimensions | ||
) |
Set the mesh, according to the following input parameters
- Parameters
-
[in] shape pointer to an external allocated BasicShape object [in] dimensions number of mesh points for each coordinate.
Definition at line 934 of file BasicMeshes.cpp.
◆ setMesh() [3/4]
void mimmo::UStructMesh::setMesh | ( | darray3E & | origin, |
darray3E & | span, | ||
ShapeType | type, | ||
dvector1D & | spacing | ||
) |
Set the mesh, according to the following input parameters
- Parameters
-
[in] origin 3D point baricenter of your mesh [in] span span for each coordinate defining your mesh [in] type shape of your mesh, based on ShapeType enum.(option available are: CUBE(default), CYLINDER, SPHERE,WEDGE) [in] spacing fixed spacing for each coordinate
Definition at line 893 of file BasicMeshes.cpp.
◆ setMesh() [4/4]
void mimmo::UStructMesh::setMesh | ( | darray3E & | origin, |
darray3E & | span, | ||
ShapeType | type, | ||
iarray3E & | dimensions | ||
) |
Set the mesh, according to the following input parameters
- Parameters
-
[in] origin 3D point baricenter of your mesh [in] span span for each coordinate defining your mesh [in] type shape of your mesh, based on ShapeType enum.(option available are: CUBE(default), CYLINDER, SPHERE, WEDGE) [in] dimensions number of mesh points for each coordinate.
Definition at line 874 of file BasicMeshes.cpp.
◆ setOrigin()
void mimmo::UStructMesh::setOrigin | ( | darray3E | origin | ) |
Set the origin of your shape. The origin is meant as the baricenter of your shape in absolute r.s. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] origin new origin point
Definition at line 541 of file BasicMeshes.cpp.
◆ setRefSystem() [1/3]
Set new axis orientation of the local reference system of your mesh core shape. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] axis0 first axis [in] axis1 second axis [in] axis2 third axis
if chosen axes are not orthogonal, doing nothing
Definition at line 619 of file BasicMeshes.cpp.
◆ setRefSystem() [2/3]
void mimmo::UStructMesh::setRefSystem | ( | dmatrix33E | axes | ) |
Set new axis orientation of the local reference system of your mesh core shape Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] axes new direction of all local axes.
Definition at line 658 of file BasicMeshes.cpp.
◆ setRefSystem() [3/3]
void mimmo::UStructMesh::setRefSystem | ( | int | label, |
darray3E | axis | ||
) |
Set new axis orientation of the local reference system of your mesh core shape Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] label 0,1,2 identify local x,y,z axis of the primitive shape [in] axis new direction of selected local axis.
Definition at line 638 of file BasicMeshes.cpp.
◆ setShape() [1/3]
void mimmo::UStructMesh::setShape | ( | const BasicShape * | shape | ) |
Set mesh shape, copying an external BasicShape object . Mesh is still not build. use UStructMesh::execute()/build() to build the mesh.
- Parameters
-
[in] shape pointer to an external allocated BasicShape object
Definition at line 777 of file BasicMeshes.cpp.
◆ setShape() [2/3]
void mimmo::UStructMesh::setShape | ( | int | itype = 0 | ) |
Set the shape, according to the following input parameters and the already saved/default parmaters. Mesh is still not build. use UStructMesh::execute()/build() to build the mesh.
- Parameters
-
[in] itype shape of your mesh, casted to enum.(option available are: 0-CUBE(default), 1-CYLINDER, 2-SPHERE)
Definition at line 703 of file BasicMeshes.cpp.
◆ setShape() [3/3]
void mimmo::UStructMesh::setShape | ( | ShapeType | type = ShapeType::CUBE | ) |
Set the shape, according to the following input parameters and the already saved/default parmaters. Mesh is still not build. use UStructMesh::execute()/build() to build the mesh.
- Parameters
-
[in] type shape of your mesh, based on ShapeType enum.(option available are: CUBE(default), CYLINDER, SPHERE)
- Examples
- core_example_00002.cpp, genericinput_example_00003.cpp, ioofoam_example_00001.cpp, and utils_example_00004.cpp.
Definition at line 713 of file BasicMeshes.cpp.
◆ setSpan() [1/2]
void mimmo::UStructMesh::setSpan | ( | darray3E | s | ) |
Set the span of your shape, according to its local reference system. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] s coordinates span
Definition at line 577 of file BasicMeshes.cpp.
◆ setSpan() [2/2]
void mimmo::UStructMesh::setSpan | ( | double | s0, |
double | s1, | ||
double | s2 | ||
) |
Set the span of your shape, according to its local reference system. Info is just passed and stored in memory, but no modifications are applied to your current mesh. To apply current modifications use UStructMesh::execute()/build() method.
- Parameters
-
[in] s0 first coordinate span [in] s1 second coordinate span [in] s2 third coordinate span
- Examples
- core_example_00002.cpp, genericinput_example_00003.cpp, ioofoam_example_00001.cpp, and utils_example_00004.cpp.
Definition at line 558 of file BasicMeshes.cpp.
◆ swap()
|
noexcept |
Swap method, exchange data between the current class and another of the same type. Data of one class will be assigned to the other and vice versa.
- Parameters
-
[in] x UstructMesh object to be swapped.
Definition at line 139 of file BasicMeshes.cpp.
◆ transfToGlobal() [1/3]
- Returns
- transformed point from Local to Global reference system
- Parameters
-
[in] point in local reference system
Definition at line 1090 of file BasicMeshes.cpp.
◆ transfToGlobal() [2/3]
- Returns
- list of transformed point from Local to Global reference system
- Parameters
-
[in] list_points in local reference system
Definition at line 1108 of file BasicMeshes.cpp.
◆ transfToGlobal() [3/3]
- Returns
- transformed point from Local to Global reference system
- Parameters
-
[in] point in local reference system
Definition at line 1098 of file BasicMeshes.cpp.
◆ transfToLocal() [1/3]
- Returns
- transformed point from Global to Local reference system
- Parameters
-
[in] point in global reference system
Definition at line 1121 of file BasicMeshes.cpp.
◆ transfToLocal() [2/3]
- Returns
- list of transformed point from Global to Local reference system
- Parameters
-
[in] list_points in global reference system
Definition at line 1137 of file BasicMeshes.cpp.
◆ transfToLocal() [3/3]
Transform point from Global to Local ref system
Definition at line 1126 of file BasicMeshes.cpp.
Member Data Documentation
◆ m_dx
|
protected |
Mesh spacing in x direction
Definition at line 48 of file BasicMeshes.hpp.
◆ m_dy
|
protected |
Mesh spacing in y direction
Definition at line 49 of file BasicMeshes.hpp.
◆ m_dz
|
protected |
Mesh spacing in z direction
Definition at line 50 of file BasicMeshes.hpp.
◆ m_isBuild
|
protected |
check if mesh is build according to the currently set parameters, or not
Definition at line 65 of file BasicMeshes.hpp.
◆ m_nx
|
protected |
Mesh number of cells in x direction
Definition at line 51 of file BasicMeshes.hpp.
◆ m_ny
|
protected |
Mesh number of cells in y direction
Definition at line 52 of file BasicMeshes.hpp.
◆ m_nz
|
protected |
Mesh number of cells in z direction
Definition at line 53 of file BasicMeshes.hpp.
◆ m_setInfLimits
|
protected |
True if inferior limits has been set and a shape is not available yet
Definition at line 63 of file BasicMeshes.hpp.
◆ m_setorigin
|
protected |
True if origin has been set and a shape is not available yet
Definition at line 61 of file BasicMeshes.hpp.
◆ m_setRefSys
|
protected |
True if reference system has been set and a shape is not available yet
Definition at line 64 of file BasicMeshes.hpp.
◆ m_setspan
|
protected |
True if span has been set and a shape is not available yet
Definition at line 62 of file BasicMeshes.hpp.
◆ m_shape
|
protected |
unique pointer to BasicShape core of the mesh, for Internal USE
Definition at line 47 of file BasicMeshes.hpp.
◆ m_xedge
|
protected |
Lists holding the x-point coordinate of the mesh, in local reference system
Definition at line 57 of file BasicMeshes.hpp.
◆ m_xnode
|
protected |
Lists holding the x-center cells coordinate of the mesh, in local reference sistem
Definition at line 54 of file BasicMeshes.hpp.
◆ m_yedge
|
protected |
Lists holding the y-point coordinate of the mesh, in local reference system
Definition at line 58 of file BasicMeshes.hpp.
◆ m_ynode
|
protected |
Lists holding the y-center cells coordinate of the mesh, in local reference sistem
Definition at line 55 of file BasicMeshes.hpp.
◆ m_zedge
|
protected |
Lists holding the z-point coordinate of the mesh, in local reference system
Definition at line 59 of file BasicMeshes.hpp.
◆ m_znode
|
protected |
Lists holding the z-center cells coordinate of the mesh, in local reference sistem
Definition at line 56 of file BasicMeshes.hpp.
The documentation for this class was generated from the following files:
- src/core/BasicMeshes.hpp
- src/core/BasicMeshes.cpp
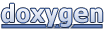