Apply is the class that applies the deformation resulting from a manipulation object to the geometry. More...
#include <Apply.hpp>
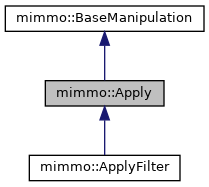
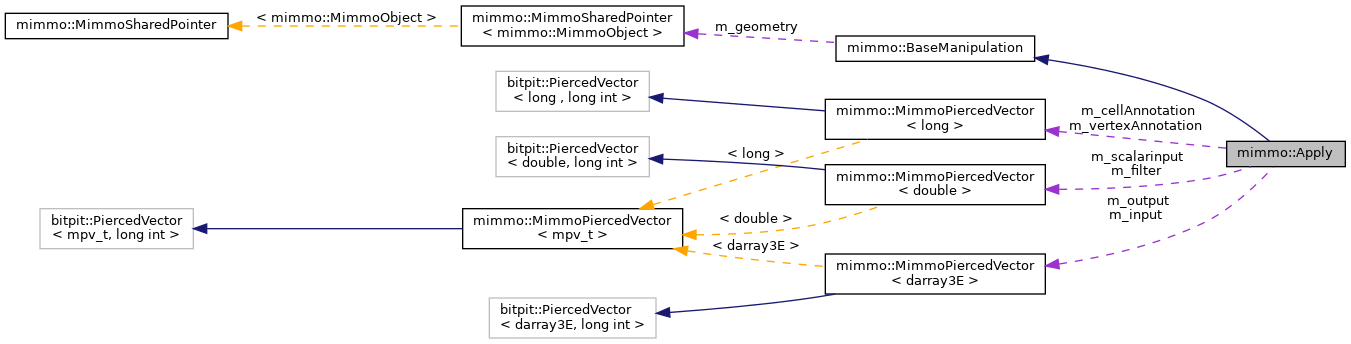
Public Member Functions | |
Apply () | |
Apply (const Apply &other) | |
Apply (const bitpit::Config::Section &rootXML) | |
~Apply () | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
MimmoPiercedVector< long > * | getAnnotatedCells () |
MimmoPiercedVector< long > * | getAnnotatedVertices () |
dmpvecarr3E * | getOutput () |
void | setAnnotation (bool activate) |
void | setAnnotationThreshold (double threshold) |
void | setCellsAnnotationName (const std::string &label) |
void | setFilter (dmpvector1D *input) |
void | setInput (dmpvecarr3E *input) |
void | setScalarInput (dmpvector1D *input) |
void | setScaling (double alpha) |
void | setVerticesAnnotationName (const std::string &label) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | checkInput () |
void | swap (Apply &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
std::string | m_annCellLabel |
bool | m_annotation |
double | m_annotationThres |
std::string | m_annVertexLabel |
MimmoPiercedVector< long > | m_cellAnnotation |
double | m_factor |
dmpvector1D | m_filter |
dmpvecarr3E | m_input |
dmpvecarr3E | m_output |
dmpvector1D | m_scalarinput |
MimmoPiercedVector< long > | m_vertexAnnotation |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Apply is derived from BaseManipulation class. It uses the base member m_geometry to apply the result of the parent manipulator to the target mimmo object. The deformation displacements have to be passed to the input member of base class through a pin linking or set by the user, i.e. one has to use setInput method to set the displacements to be applied to the geometry. The displacements can be isotropically scaled by setting a scaling factor. The displacements can be passed as scalar field. In this case the scalar field is used as magnitude of a displacements field with direction along the normal of the surface on each vertex. The Apply block allows to pass a scalar filter field; the filter field is applied to the displacements field before the geometry deformation.
After the execution of an object Apply, the original geometry will be modified. The resulting deformation field is provided as output of the block.
Ports available in Apply Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | setInput | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | setScalarInput | (MC_SCALAR,MD_MPVECFLOAT_) |
M_FILTER | setFilter | (MC_SCALAR,MD_MPVECFLOAT_) |
M_GEOM | setGeometry | (MC_SCALAR,MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
M_GDISPLS | getOutput | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
M_LONGFIELD | getAnnotatedVertices | (MC_SCALAR,MD_MPVECLONG_) |
M_LONGFIELD2 | getAnnotatedCells | (MC_SCALAR,MD_MPVECLONG_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName : name of the class as
mimmo.Apply
; - Priority : uint marking priority in multi-chain execution;
Proper of the class:
- Annotation : 0/1 boolean, if 1 provide list of cell/vertices into deformation as annotation list;
- CellsAnnotationName : (string) define label to mark annotated cells (list of cell-ids involved into deformation);
- VerticesAnnotationName : (string) define label to mark annotated vertices (list of vertex-ids involved into deformation);
- AnnotationThreshold : double val >0, threshold over which all mesh elements with deformation field over it are annotated;
Geometry and one of the Inputs have to be mandatorily passed through port.
- Examples
- genericinput_example_00005.cpp, geohandlers_example_00003.cpp, iocgns_example_00001.cpp, ioofoam_example_00001.cpp, manipulators_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, manipulators_example_00008.cpp, and utils_example_00003.cpp.
Constructor & Destructor Documentation
◆ Apply() [1/3]
◆ Apply() [2/3]
mimmo::Apply::Apply | ( | const bitpit::Config::Section & | rootXML | ) |
◆ ~Apply()
◆ Apply() [3/3]
mimmo::Apply::Apply | ( | const Apply & | other | ) |
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::ApplyFilter.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::ApplyFilter.
◆ checkInput()
|
protected |
◆ execute()
|
virtual |
Execution command. It applies the deformation stored in the input of base class (casting the input for apply object to dvecarr3E) to the linked geometry. After exec() the original geometry will be permanently modified.
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::ApplyFilter.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::ApplyFilter.
◆ getAnnotatedCells()
MimmoPiercedVector< long > * mimmo::Apply::getAnnotatedCells | ( | ) |
◆ getAnnotatedVertices()
MimmoPiercedVector< long > * mimmo::Apply::getAnnotatedVertices | ( | ) |
◆ getOutput()
dmpvecarr3E * mimmo::Apply::getOutput | ( | ) |
◆ setAnnotation()
void mimmo::Apply::setAnnotation | ( | bool | activate | ) |
◆ setAnnotationThreshold()
void mimmo::Apply::setAnnotationThreshold | ( | double | threshold | ) |
◆ setCellsAnnotationName()
void mimmo::Apply::setCellsAnnotationName | ( | const std::string & | label | ) |
◆ setFilter()
void mimmo::Apply::setFilter | ( | dmpvector1D * | input | ) |
◆ setInput()
void mimmo::Apply::setInput | ( | dmpvecarr3E * | input | ) |
◆ setScalarInput()
void mimmo::Apply::setScalarInput | ( | dmpvector1D * | input | ) |
It sets the displacements given as scalar input. It makes sense only for surface geometries. The displacements will be directed as the surface normals.
- Parameters
-
[in] input Input displacements of the geometry vertices given as module of normal directed vectors.
◆ setScaling()
void mimmo::Apply::setScaling | ( | double | alpha | ) |
It sets the displacements scalar factor. The displacements will be scaled by using this factor.
- Parameters
-
[in] alpha Scale factor of displacements field.
- Examples
- ioofoam_example_00001.cpp.
◆ setVerticesAnnotationName()
void mimmo::Apply::setVerticesAnnotationName | ( | const std::string & | label | ) |
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_annCellLabel
|
protected |
◆ m_annotation
|
protected |
◆ m_annotationThres
|
protected |
◆ m_annVertexLabel
|
protected |
◆ m_cellAnnotation
|
protected |
◆ m_factor
|
protected |
◆ m_filter
|
protected |
◆ m_input
|
protected |
◆ m_output
|
protected |
◆ m_scalarinput
|
protected |
◆ m_vertexAnnotation
|
protected |
The documentation for this class was generated from the following files:
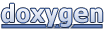