Apply.hpp
MimmoPiercedVector< long > * getAnnotatedVertices()
Definition: Apply.cpp:226
void setAnnotationThreshold(double threshold)
Definition: Apply.cpp:179
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setCellsAnnotationName(const std::string &label)
Definition: Apply.cpp:189
MimmoPiercedVector< long > m_vertexAnnotation
Definition: Apply.hpp:135
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Apply.cpp:310
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
MimmoPiercedVector< long > * getAnnotatedCells()
Definition: Apply.cpp:235
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Apply.cpp:369
void setVerticesAnnotationName(const std::string &label)
Definition: Apply.cpp:203
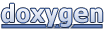