Apply.cpp
81 //annotation structures are not copied, they are filled each time in execution if m_annotation is true.
110 built = (built && createPortIn<dmpvecarr3E*, Apply>(this, &Apply::setInput, M_GDISPLS, true, 1));
111 built = (built && createPortIn<dmpvector1D*, Apply>(this, &Apply::setScalarInput, M_SCALARFIELD, true, 1));
113 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, Apply>(this, &BaseManipulation::setGeometry, M_GEOM, true));
115 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, Apply>(this, &BaseManipulation::getGeometry, M_GEOM));
117 built = (built && createPortOut<MimmoPiercedVector<long>*, Apply>(this, &Apply::getAnnotatedVertices, M_LONGFIELD));
118 built = (built && createPortOut<MimmoPiercedVector<long>*, Apply>(this, &Apply::getAnnotatedCells, M_LONGFIELD2));
285 livector1D celllist = getGeometry()->getCellFromVertexList(m_vertexAnnotation.getIds(), false); //false! any cell sharing a moved vertex
445 (*m_log)<<"Not valid input found in "<<m_name<<". Proceeding with default zero field"<<std::endl;
MimmoPiercedVector< long > * getAnnotatedVertices()
Definition: Apply.cpp:226
void setAnnotationThreshold(double threshold)
Definition: Apply.cpp:179
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
void setCellsAnnotationName(const std::string &label)
Definition: Apply.cpp:189
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MimmoPiercedVector< long > m_vertexAnnotation
Definition: Apply.hpp:135
@ CELL
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Apply.cpp:310
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
MimmoPiercedVector< long > * getAnnotatedCells()
Definition: Apply.cpp:235
@ POINT
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Apply.cpp:369
void setVerticesAnnotationName(const std::string &label)
Definition: Apply.cpp:203
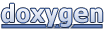