ApplyFilter is a class that applies a filter field to a deformation field defined on a geometry. More...
#include <ApplyFilter.hpp>
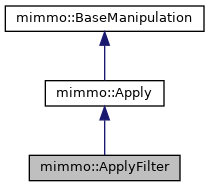
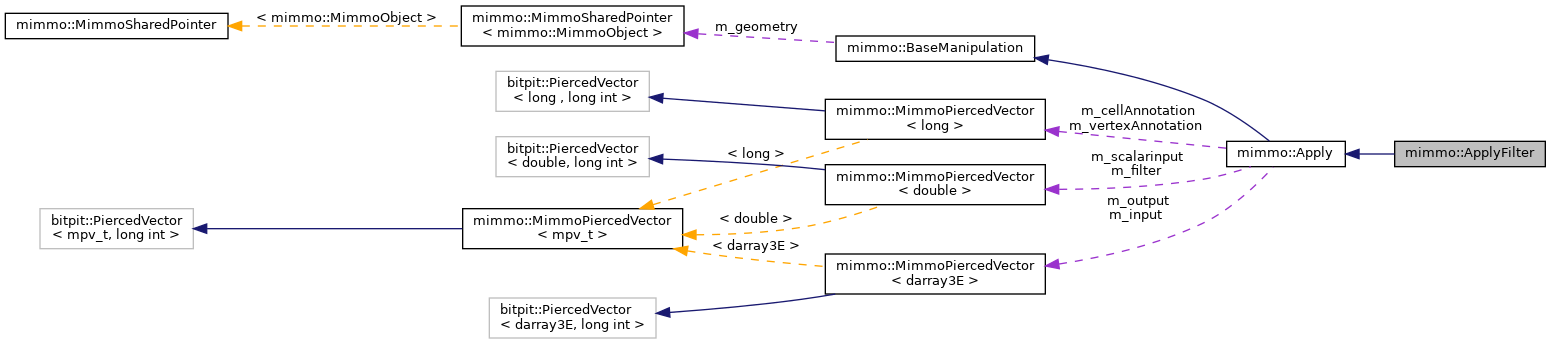
Public Member Functions | |
ApplyFilter () | |
ApplyFilter (const ApplyFilter &other) | |
ApplyFilter (const bitpit::Config::Section &rootXML) | |
~ApplyFilter () | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
dmpvecarr3E * | getOutput () |
void | setScaling (double alpha) |
![]() | |
Apply () | |
Apply (const Apply &other) | |
Apply (const bitpit::Config::Section &rootXML) | |
~Apply () | |
MimmoPiercedVector< long > * | getAnnotatedCells () |
MimmoPiercedVector< long > * | getAnnotatedVertices () |
dmpvecarr3E * | getOutput () |
void | setAnnotation (bool activate) |
void | setAnnotationThreshold (double threshold) |
void | setCellsAnnotationName (const std::string &label) |
void | setFilter (dmpvector1D *input) |
void | setInput (dmpvecarr3E *input) |
void | setScalarInput (dmpvector1D *input) |
void | setScaling (double alpha) |
void | setVerticesAnnotationName (const std::string &label) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | checkInput () |
void | swap (ApplyFilter &x) noexcept |
![]() | |
void | checkInput () |
void | swap (Apply &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
double | m_factor |
dmpvector1D | m_filter |
dmpvecarr3E | m_input |
dmpvecarr3E | m_output |
dmpvector1D | m_scalarinput |
![]() | |
std::string | m_annCellLabel |
bool | m_annotation |
double | m_annotationThres |
std::string | m_annVertexLabel |
MimmoPiercedVector< long > | m_cellAnnotation |
double | m_factor |
dmpvector1D | m_filter |
dmpvecarr3E | m_input |
dmpvecarr3E | m_output |
dmpvector1D | m_scalarinput |
MimmoPiercedVector< long > | m_vertexAnnotation |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
ApplyFilter is a class that applies a filter field to a deformation field defined on a geometry.
ApplyFilter is custom derivation of Apply class, targeted to the manipulation/modulation of a displacements field by means of a custom filter field, both externally provided, both referring to a target geometry. After the execution, ApplyFilter provides as result the filtered deformation field. BEWARE: Differently from its base class, it does not apply the final displacement field to the geometry.To achieve it, use an Apply block.
Ports available in ApplyFilter Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | setInput | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | setScalarInput | (MC_SCALAR,MD_MPVECFLOAT_) |
M_FILTER | setFilter | (MC_SCALAR,MD_MPVECFLOAT_) |
M_GEOM | setGeometry | (MC_SCALAR,MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | geOutput | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName : name of the class as
mimmo.ApplyFilter
; - Priority : uint marking priority in multi-chain execution;
Proper of the class:
Definition at line 73 of file ApplyFilter.hpp.
Constructor & Destructor Documentation
◆ ApplyFilter() [1/3]
mimmo::ApplyFilter::ApplyFilter | ( | ) |
Default constructor of ApplyFilter
Definition at line 31 of file ApplyFilter.cpp.
◆ ApplyFilter() [2/3]
mimmo::ApplyFilter::ApplyFilter | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 40 of file ApplyFilter.cpp.
◆ ~ApplyFilter()
mimmo::ApplyFilter::~ApplyFilter | ( | ) |
Default destructor of ApplyFilter
Definition at line 57 of file ApplyFilter.cpp.
◆ ApplyFilter() [3/3]
mimmo::ApplyFilter::ApplyFilter | ( | const ApplyFilter & | other | ) |
Copy constructor of ApplyFilter.
Definition at line 62 of file ApplyFilter.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::Apply.
Definition at line 117 of file ApplyFilter.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Reimplemented from mimmo::Apply.
Definition at line 77 of file ApplyFilter.cpp.
◆ checkInput()
|
protected |
Check if the input is related to the target geometry. Check the coherence of filter field. If not create a zero input field.
Definition at line 156 of file ApplyFilter.cpp.
◆ execute()
|
virtual |
Execution command. It applies the filter field to the deformation field stored in the input of base class (casting the input for apply object to dvecarr3E). Scaling factor is applied too if passed by the user. After exec() the linked geometry is not modified.
Reimplemented from mimmo::Apply.
Definition at line 96 of file ApplyFilter.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::Apply.
Definition at line 141 of file ApplyFilter.cpp.
◆ getOutput()
dmpvecarr3E * mimmo::Apply::getOutput |
◆ setScaling()
void mimmo::Apply::setScaling |
◆ swap()
|
protectednoexcept |
< storing vector fields of floats for output (input modified with filter and factor)
Swap function
- Parameters
-
[in] x object to be swapped
Definition at line 69 of file ApplyFilter.cpp.
Member Data Documentation
◆ m_factor
|
protected |
◆ m_filter
|
protected |
◆ m_input
|
protected |
◆ m_output
|
protected |
◆ m_scalarinput
|
protected |
The documentation for this class was generated from the following files:
- src/manipulators/ApplyFilter.hpp
- src/manipulators/ApplyFilter.cpp
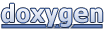